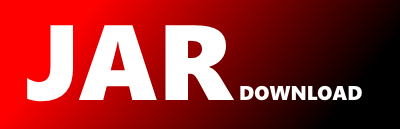
com.pulumi.vault.aws.kotlin.AwsFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.aws.kotlin
import com.pulumi.vault.aws.AwsFunctions.getAccessCredentialsPlain
import com.pulumi.vault.aws.AwsFunctions.getStaticAccessCredentialsPlain
import com.pulumi.vault.aws.kotlin.inputs.GetAccessCredentialsPlainArgs
import com.pulumi.vault.aws.kotlin.inputs.GetAccessCredentialsPlainArgsBuilder
import com.pulumi.vault.aws.kotlin.inputs.GetStaticAccessCredentialsPlainArgs
import com.pulumi.vault.aws.kotlin.inputs.GetStaticAccessCredentialsPlainArgsBuilder
import com.pulumi.vault.aws.kotlin.outputs.GetAccessCredentialsResult
import com.pulumi.vault.aws.kotlin.outputs.GetStaticAccessCredentialsResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.vault.aws.kotlin.outputs.GetAccessCredentialsResult.Companion.toKotlin as getAccessCredentialsResultToKotlin
import com.pulumi.vault.aws.kotlin.outputs.GetStaticAccessCredentialsResult.Companion.toKotlin as getStaticAccessCredentialsResultToKotlin
public object AwsFunctions {
/**
* ## Example Usage
*
* ```yaml
* resources:
* aws:
* type: vault:aws:SecretBackend
* properties:
* accessKey: AKIA.....
* secretKey: SECRETKEYFROMAWS
* role:
* type: vault:aws:SecretBackendRole
* properties:
* backend: ${aws.path}
* name: test
* policy: |
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Effect": "Allow",
* "Action": "iam:*",
* "Resource": "*"
* }
* ]
* }
* variables:
* # generally, these blocks would be in a different module
* creds:
* fn::invoke:
* Function: vault:aws:getAccessCredentials
* Arguments:
* backend: ${aws.path}
* role: ${role.name}
* ```
*
* @param argument A collection of arguments for invoking getAccessCredentials.
* @return A collection of values returned by getAccessCredentials.
*/
public suspend fun getAccessCredentials(argument: GetAccessCredentialsPlainArgs): GetAccessCredentialsResult =
getAccessCredentialsResultToKotlin(getAccessCredentialsPlain(argument.toJava()).await())
/**
* @see [getAccessCredentials].
* @param backend The path to the AWS secret backend to
* read credentials from, with no leading or trailing `/`s.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param region The region the read credentials belong to.
* @param role The name of the AWS secret backend role to read
* credentials from, with no leading or trailing `/`s.
* @param roleArn The specific AWS ARN to use
* from the configured role. If the role does not have multiple ARNs, this does
* not need to be specified.
* @param ttl Specifies the TTL for the use of the STS token. This
* is specified as a string with a duration suffix. Valid only when
* `credential_type` of the connected `vault.aws.SecretBackendRole` resource is `assumed_role` or `federation_token`
* @param type The type of credentials to read. Defaults
* to `"creds"`, which just returns an AWS Access Key ID and Secret
* Key. Can also be set to `"sts"`, which will return a security token
* in addition to the keys.
* @return A collection of values returned by getAccessCredentials.
*/
public suspend fun getAccessCredentials(
backend: String,
namespace: String? = null,
region: String? = null,
role: String,
roleArn: String? = null,
ttl: String? = null,
type: String? = null,
): GetAccessCredentialsResult {
val argument = GetAccessCredentialsPlainArgs(
backend = backend,
namespace = namespace,
region = region,
role = role,
roleArn = roleArn,
ttl = ttl,
type = type,
)
return getAccessCredentialsResultToKotlin(getAccessCredentialsPlain(argument.toJava()).await())
}
/**
* @see [getAccessCredentials].
* @param argument Builder for [com.pulumi.vault.aws.kotlin.inputs.GetAccessCredentialsPlainArgs].
* @return A collection of values returned by getAccessCredentials.
*/
public suspend fun getAccessCredentials(argument: suspend GetAccessCredentialsPlainArgsBuilder.() -> Unit): GetAccessCredentialsResult {
val builder = GetAccessCredentialsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccessCredentialsResultToKotlin(getAccessCredentialsPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getStaticAccessCredentials.
* @return A collection of values returned by getStaticAccessCredentials.
*/
public suspend fun getStaticAccessCredentials(argument: GetStaticAccessCredentialsPlainArgs): GetStaticAccessCredentialsResult =
getStaticAccessCredentialsResultToKotlin(getStaticAccessCredentialsPlain(argument.toJava()).await())
/**
* @see [getStaticAccessCredentials].
* @param backend
* @param name
* @param namespace
* @return A collection of values returned by getStaticAccessCredentials.
*/
public suspend fun getStaticAccessCredentials(
backend: String,
name: String,
namespace: String? = null,
): GetStaticAccessCredentialsResult {
val argument = GetStaticAccessCredentialsPlainArgs(
backend = backend,
name = name,
namespace = namespace,
)
return getStaticAccessCredentialsResultToKotlin(getStaticAccessCredentialsPlain(argument.toJava()).await())
}
/**
* @see [getStaticAccessCredentials].
* @param argument Builder for [com.pulumi.vault.aws.kotlin.inputs.GetStaticAccessCredentialsPlainArgs].
* @return A collection of values returned by getStaticAccessCredentials.
*/
public suspend fun getStaticAccessCredentials(argument: suspend GetStaticAccessCredentialsPlainArgsBuilder.() -> Unit): GetStaticAccessCredentialsResult {
val builder = GetStaticAccessCredentialsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStaticAccessCredentialsResultToKotlin(getStaticAccessCredentialsPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy