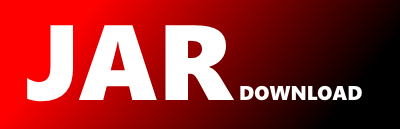
com.pulumi.vault.aws.kotlin.SecretBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.aws.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.aws.SecretBackendArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* ## Import
* AWS secret backends can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:aws/secretBackend:SecretBackend aws aws
* ```
* @property accessKey The AWS Access Key ID this backend should use to
* issue new credentials. Vault uses the official AWS SDK to authenticate, and thus can also use standard AWS environment credentials, shared file credentials or IAM role/ECS task credentials.
* @property defaultLeaseTtlSeconds The default TTL for credentials
* issued by this backend.
* @property description A human-friendly description for this backend.
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property iamEndpoint Specifies a custom HTTP IAM endpoint to use.
* @property identityTokenAudience The audience claim value. Requires Vault 1.16+.
* @property identityTokenKey The key to use for signing identity tokens. Requires Vault 1.16+.
* @property identityTokenTtl The TTL of generated identity tokens in seconds. Requires Vault 1.16+.
* @property local Specifies whether the secrets mount will be marked as local. Local mounts are not replicated to performance replicas.
* @property maxLeaseTtlSeconds The maximum TTL that can be requested
* for credentials issued by this backend.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `aws`.
* @property region The AWS region to make API calls against. Defaults to us-east-1.
* @property roleArn Role ARN to assume for plugin identity token federation. Requires Vault 1.16+.
* ```
* {{ if (eq .Type "STS") }}
* {{ printf "vault-%s-%s" (unix_time) (random 20) | truncate 32 }}
* {{ else }}
* {{ printf "vault-%s-%s-%s" (printf "%s-%s" (.DisplayName) (.PolicyName) | truncate 42) (unix_time) (random 20) | truncate 64 }}
* {{ end }}
* ```
* @property secretKey The AWS Secret Access Key to use when generating new credentials.
* @property stsEndpoint Specifies a custom HTTP STS endpoint to use.
* @property usernameTemplate Template describing how dynamic usernames are generated. The username template is used to generate both IAM usernames (capped at 64 characters) and STS usernames (capped at 32 characters). If no template is provided the field defaults to the template:
*/
public data class SecretBackendArgs(
public val accessKey: Output? = null,
public val defaultLeaseTtlSeconds: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val iamEndpoint: Output? = null,
public val identityTokenAudience: Output? = null,
public val identityTokenKey: Output? = null,
public val identityTokenTtl: Output? = null,
public val local: Output? = null,
public val maxLeaseTtlSeconds: Output? = null,
public val namespace: Output? = null,
public val path: Output? = null,
public val region: Output? = null,
public val roleArn: Output? = null,
public val secretKey: Output? = null,
public val stsEndpoint: Output? = null,
public val usernameTemplate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.aws.SecretBackendArgs =
com.pulumi.vault.aws.SecretBackendArgs.builder()
.accessKey(accessKey?.applyValue({ args0 -> args0 }))
.defaultLeaseTtlSeconds(defaultLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.iamEndpoint(iamEndpoint?.applyValue({ args0 -> args0 }))
.identityTokenAudience(identityTokenAudience?.applyValue({ args0 -> args0 }))
.identityTokenKey(identityTokenKey?.applyValue({ args0 -> args0 }))
.identityTokenTtl(identityTokenTtl?.applyValue({ args0 -> args0 }))
.local(local?.applyValue({ args0 -> args0 }))
.maxLeaseTtlSeconds(maxLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.region(region?.applyValue({ args0 -> args0 }))
.roleArn(roleArn?.applyValue({ args0 -> args0 }))
.secretKey(secretKey?.applyValue({ args0 -> args0 }))
.stsEndpoint(stsEndpoint?.applyValue({ args0 -> args0 }))
.usernameTemplate(usernameTemplate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendArgs].
*/
@PulumiTagMarker
public class SecretBackendArgsBuilder internal constructor() {
private var accessKey: Output? = null
private var defaultLeaseTtlSeconds: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var iamEndpoint: Output? = null
private var identityTokenAudience: Output? = null
private var identityTokenKey: Output? = null
private var identityTokenTtl: Output? = null
private var local: Output? = null
private var maxLeaseTtlSeconds: Output? = null
private var namespace: Output? = null
private var path: Output? = null
private var region: Output? = null
private var roleArn: Output? = null
private var secretKey: Output? = null
private var stsEndpoint: Output? = null
private var usernameTemplate: Output? = null
/**
* @param value The AWS Access Key ID this backend should use to
* issue new credentials. Vault uses the official AWS SDK to authenticate, and thus can also use standard AWS environment credentials, shared file credentials or IAM role/ECS task credentials.
*/
@JvmName("rdykueyatffsuctl")
public suspend fun accessKey(`value`: Output) {
this.accessKey = value
}
/**
* @param value The default TTL for credentials
* issued by this backend.
*/
@JvmName("uhguwkldcblljaci")
public suspend fun defaultLeaseTtlSeconds(`value`: Output) {
this.defaultLeaseTtlSeconds = value
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("rjjhtufgmomgqxih")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("vrdiknwojddyqktq")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value Specifies a custom HTTP IAM endpoint to use.
*/
@JvmName("qsddbdhppvhqhckl")
public suspend fun iamEndpoint(`value`: Output) {
this.iamEndpoint = value
}
/**
* @param value The audience claim value. Requires Vault 1.16+.
*/
@JvmName("rpmsegfvhpdtxivv")
public suspend fun identityTokenAudience(`value`: Output) {
this.identityTokenAudience = value
}
/**
* @param value The key to use for signing identity tokens. Requires Vault 1.16+.
*/
@JvmName("udamqxxsyfohcnkd")
public suspend fun identityTokenKey(`value`: Output) {
this.identityTokenKey = value
}
/**
* @param value The TTL of generated identity tokens in seconds. Requires Vault 1.16+.
*/
@JvmName("cdsjeqtdplfautfx")
public suspend fun identityTokenTtl(`value`: Output) {
this.identityTokenTtl = value
}
/**
* @param value Specifies whether the secrets mount will be marked as local. Local mounts are not replicated to performance replicas.
*/
@JvmName("cytqipuelklrkped")
public suspend fun local(`value`: Output) {
this.local = value
}
/**
* @param value The maximum TTL that can be requested
* for credentials issued by this backend.
*/
@JvmName("nwqbhpcbtpirgtiu")
public suspend fun maxLeaseTtlSeconds(`value`: Output) {
this.maxLeaseTtlSeconds = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("qnylwgahbjxfrjum")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `aws`.
*/
@JvmName("ojiihnteiupkatgg")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value The AWS region to make API calls against. Defaults to us-east-1.
*/
@JvmName("liejlkvrgphnrlyd")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value Role ARN to assume for plugin identity token federation. Requires Vault 1.16+.
* ```
* {{ if (eq .Type "STS") }}
* {{ printf "vault-%s-%s" (unix_time) (random 20) | truncate 32 }}
* {{ else }}
* {{ printf "vault-%s-%s-%s" (printf "%s-%s" (.DisplayName) (.PolicyName) | truncate 42) (unix_time) (random 20) | truncate 64 }}
* {{ end }}
* ```
*/
@JvmName("rfxfbkmyrycnuewu")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The AWS Secret Access Key to use when generating new credentials.
*/
@JvmName("wgsoteavuxxdqupn")
public suspend fun secretKey(`value`: Output) {
this.secretKey = value
}
/**
* @param value Specifies a custom HTTP STS endpoint to use.
*/
@JvmName("aurbokwdghrsswnp")
public suspend fun stsEndpoint(`value`: Output) {
this.stsEndpoint = value
}
/**
* @param value Template describing how dynamic usernames are generated. The username template is used to generate both IAM usernames (capped at 64 characters) and STS usernames (capped at 32 characters). If no template is provided the field defaults to the template:
*/
@JvmName("jbncgvujtymkabjj")
public suspend fun usernameTemplate(`value`: Output) {
this.usernameTemplate = value
}
/**
* @param value The AWS Access Key ID this backend should use to
* issue new credentials. Vault uses the official AWS SDK to authenticate, and thus can also use standard AWS environment credentials, shared file credentials or IAM role/ECS task credentials.
*/
@JvmName("jfndcuxewiquvtke")
public suspend fun accessKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessKey = mapped
}
/**
* @param value The default TTL for credentials
* issued by this backend.
*/
@JvmName("dymiguikoojkvmuk")
public suspend fun defaultLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultLeaseTtlSeconds = mapped
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("hbhosyifkbjcumty")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("hoqfntgkuweopxqm")
public suspend fun disableRemount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableRemount = mapped
}
/**
* @param value Specifies a custom HTTP IAM endpoint to use.
*/
@JvmName("oiaejdlullhnlxqy")
public suspend fun iamEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iamEndpoint = mapped
}
/**
* @param value The audience claim value. Requires Vault 1.16+.
*/
@JvmName("nglybrkitlfimusq")
public suspend fun identityTokenAudience(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityTokenAudience = mapped
}
/**
* @param value The key to use for signing identity tokens. Requires Vault 1.16+.
*/
@JvmName("mrtduxlllocylrud")
public suspend fun identityTokenKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityTokenKey = mapped
}
/**
* @param value The TTL of generated identity tokens in seconds. Requires Vault 1.16+.
*/
@JvmName("tfxmsvwewqlmioux")
public suspend fun identityTokenTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityTokenTtl = mapped
}
/**
* @param value Specifies whether the secrets mount will be marked as local. Local mounts are not replicated to performance replicas.
*/
@JvmName("vdccxfekcevrtfiw")
public suspend fun local(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.local = mapped
}
/**
* @param value The maximum TTL that can be requested
* for credentials issued by this backend.
*/
@JvmName("yovcmdyeefcvqlxa")
public suspend fun maxLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxLeaseTtlSeconds = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("npnfbmiuddfgmbva")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `aws`.
*/
@JvmName("tjwxvjruablopkia")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value The AWS region to make API calls against. Defaults to us-east-1.
*/
@JvmName("tkyrpckevchyprwc")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value Role ARN to assume for plugin identity token federation. Requires Vault 1.16+.
* ```
* {{ if (eq .Type "STS") }}
* {{ printf "vault-%s-%s" (unix_time) (random 20) | truncate 32 }}
* {{ else }}
* {{ printf "vault-%s-%s-%s" (printf "%s-%s" (.DisplayName) (.PolicyName) | truncate 42) (unix_time) (random 20) | truncate 64 }}
* {{ end }}
* ```
*/
@JvmName("fqmcwlitfjmdmeip")
public suspend fun roleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value The AWS Secret Access Key to use when generating new credentials.
*/
@JvmName("dwfidobrwmkripfr")
public suspend fun secretKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretKey = mapped
}
/**
* @param value Specifies a custom HTTP STS endpoint to use.
*/
@JvmName("djetxsqxhmerquuy")
public suspend fun stsEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stsEndpoint = mapped
}
/**
* @param value Template describing how dynamic usernames are generated. The username template is used to generate both IAM usernames (capped at 64 characters) and STS usernames (capped at 32 characters). If no template is provided the field defaults to the template:
*/
@JvmName("vopxpvpenwfamnls")
public suspend fun usernameTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameTemplate = mapped
}
internal fun build(): SecretBackendArgs = SecretBackendArgs(
accessKey = accessKey,
defaultLeaseTtlSeconds = defaultLeaseTtlSeconds,
description = description,
disableRemount = disableRemount,
iamEndpoint = iamEndpoint,
identityTokenAudience = identityTokenAudience,
identityTokenKey = identityTokenKey,
identityTokenTtl = identityTokenTtl,
local = local,
maxLeaseTtlSeconds = maxLeaseTtlSeconds,
namespace = namespace,
path = path,
region = region,
roleArn = roleArn,
secretKey = secretKey,
stsEndpoint = stsEndpoint,
usernameTemplate = usernameTemplate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy