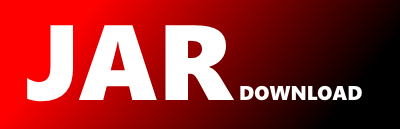
com.pulumi.vault.aws.kotlin.SecretBackendStaticRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.aws.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.aws.SecretBackendStaticRoleArgs.builder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const aws = new vault.aws.SecretBackend("aws", {
* path: "my-aws",
* description: "Obtain AWS credentials.",
* });
* const role = new vault.aws.SecretBackendStaticRole("role", {
* backend: aws.path,
* name: "test",
* username: "my-test-user",
* rotationPeriod: 3600,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* aws = vault.aws.SecretBackend("aws",
* path="my-aws",
* description="Obtain AWS credentials.")
* role = vault.aws.SecretBackendStaticRole("role",
* backend=aws.path,
* name="test",
* username="my-test-user",
* rotation_period=3600)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var aws = new Vault.Aws.SecretBackend("aws", new()
* {
* Path = "my-aws",
* Description = "Obtain AWS credentials.",
* });
* var role = new Vault.Aws.SecretBackendStaticRole("role", new()
* {
* Backend = aws.Path,
* Name = "test",
* Username = "my-test-user",
* RotationPeriod = 3600,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/aws"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* aws, err := aws.NewSecretBackend(ctx, "aws", &aws.SecretBackendArgs{
* Path: pulumi.String("my-aws"),
* Description: pulumi.String("Obtain AWS credentials."),
* })
* if err != nil {
* return err
* }
* _, err = aws.NewSecretBackendStaticRole(ctx, "role", &aws.SecretBackendStaticRoleArgs{
* Backend: aws.Path,
* Name: pulumi.String("test"),
* Username: pulumi.String("my-test-user"),
* RotationPeriod: pulumi.Int(3600),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.aws.SecretBackend;
* import com.pulumi.vault.aws.SecretBackendArgs;
* import com.pulumi.vault.aws.SecretBackendStaticRole;
* import com.pulumi.vault.aws.SecretBackendStaticRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var aws = new SecretBackend("aws", SecretBackendArgs.builder()
* .path("my-aws")
* .description("Obtain AWS credentials.")
* .build());
* var role = new SecretBackendStaticRole("role", SecretBackendStaticRoleArgs.builder()
* .backend(aws.path())
* .name("test")
* .username("my-test-user")
* .rotationPeriod("3600")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* aws:
* type: vault:aws:SecretBackend
* properties:
* path: my-aws
* description: Obtain AWS credentials.
* role:
* type: vault:aws:SecretBackendStaticRole
* properties:
* backend: ${aws.path}
* name: test
* username: my-test-user
* rotationPeriod: '3600'
* ```
*
* ## Import
* AWS secret backend static role can be imported using the full path to the role
* of the form: `/static-roles/` e.g.
* ```sh
* $ pulumi import vault:aws/secretBackendStaticRole:SecretBackendStaticRole role aws/static-roles/example-role
* ```
* @property backend The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `aws`
* @property name The name to identify this role within the backend.
* Must be unique within the backend.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property rotationPeriod How often Vault should rotate the password of the user entry.
* @property username The username of the existing AWS IAM to manage password rotation for.
*/
public data class SecretBackendStaticRoleArgs(
public val backend: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val rotationPeriod: Output? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.aws.SecretBackendStaticRoleArgs =
com.pulumi.vault.aws.SecretBackendStaticRoleArgs.builder()
.backend(backend?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.rotationPeriod(rotationPeriod?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendStaticRoleArgs].
*/
@PulumiTagMarker
public class SecretBackendStaticRoleArgsBuilder internal constructor() {
private var backend: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var rotationPeriod: Output? = null
private var username: Output? = null
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `aws`
*/
@JvmName("smbjujdgrxkkkrpq")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value The name to identify this role within the backend.
* Must be unique within the backend.
*/
@JvmName("etqjmtuyfjvxgsmi")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("jnxiukwdlfdamqgc")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value How often Vault should rotate the password of the user entry.
*/
@JvmName("uddoopqkhapydthk")
public suspend fun rotationPeriod(`value`: Output) {
this.rotationPeriod = value
}
/**
* @param value The username of the existing AWS IAM to manage password rotation for.
*/
@JvmName("hhtmcuahmlqcpscj")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `aws`
*/
@JvmName("lnqjnmaewcjwqkau")
public suspend fun backend(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backend = mapped
}
/**
* @param value The name to identify this role within the backend.
* Must be unique within the backend.
*/
@JvmName("pbnafsxniwbcdwlj")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("mcgvlkeymjavnoeb")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value How often Vault should rotate the password of the user entry.
*/
@JvmName("cnluqkhneuvboxdl")
public suspend fun rotationPeriod(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rotationPeriod = mapped
}
/**
* @param value The username of the existing AWS IAM to manage password rotation for.
*/
@JvmName("auaksmwkddvjewcm")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): SecretBackendStaticRoleArgs = SecretBackendStaticRoleArgs(
backend = backend,
name = name,
namespace = namespace,
rotationPeriod = rotationPeriod,
username = username,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy