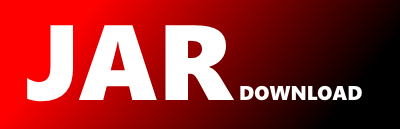
com.pulumi.vault.azure.kotlin.AuthBackendConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.azure.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [AuthBackendConfig].
*/
@PulumiTagMarker
public class AuthBackendConfigResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuthBackendConfigArgs = AuthBackendConfigArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuthBackendConfigArgsBuilder.() -> Unit) {
val builder = AuthBackendConfigArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AuthBackendConfig {
val builtJavaResource = com.pulumi.vault.azure.AuthBackendConfig(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AuthBackendConfig(builtJavaResource)
}
}
/**
* ## Example Usage
* You can setup the Azure auth engine with Workload Identity Federation (WIF) for a secret-less configuration:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.AuthBackend("example", {
* type: "azure",
* identityTokenKey: "example-key",
* });
* const exampleAuthBackendConfig = new vault.azure.AuthBackendConfig("example", {
* backend: example.path,
* tenantId: "11111111-2222-3333-4444-555555555555",
* clientId: "11111111-2222-3333-4444-555555555555",
* identityTokenAudience: "",
* identityTokenTtl: "",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.AuthBackend("example",
* type="azure",
* identity_token_key="example-key")
* example_auth_backend_config = vault.azure.AuthBackendConfig("example",
* backend=example.path,
* tenant_id="11111111-2222-3333-4444-555555555555",
* client_id="11111111-2222-3333-4444-555555555555",
* identity_token_audience="",
* identity_token_ttl="")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.AuthBackend("example", new()
* {
* Type = "azure",
* IdentityTokenKey = "example-key",
* });
* var exampleAuthBackendConfig = new Vault.Azure.AuthBackendConfig("example", new()
* {
* Backend = example.Path,
* TenantId = "11111111-2222-3333-4444-555555555555",
* ClientId = "11111111-2222-3333-4444-555555555555",
* IdentityTokenAudience = "",
* IdentityTokenTtl = "",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/azure"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := vault.NewAuthBackend(ctx, "example", &vault.AuthBackendArgs{
* Type: pulumi.String("azure"),
* IdentityTokenKey: pulumi.String("example-key"),
* })
* if err != nil {
* return err
* }
* _, err = azure.NewAuthBackendConfig(ctx, "example", &azure.AuthBackendConfigArgs{
* Backend: example.Path,
* TenantId: pulumi.String("11111111-2222-3333-4444-555555555555"),
* ClientId: pulumi.String("11111111-2222-3333-4444-555555555555"),
* IdentityTokenAudience: pulumi.String(""),
* IdentityTokenTtl: pulumi.Int(""),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.azure.AuthBackendConfig;
* import com.pulumi.vault.azure.AuthBackendConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AuthBackend("example", AuthBackendArgs.builder()
* .type("azure")
* .identityTokenKey("example-key")
* .build());
* var exampleAuthBackendConfig = new AuthBackendConfig("exampleAuthBackendConfig", AuthBackendConfigArgs.builder()
* .backend(example.path())
* .tenantId("11111111-2222-3333-4444-555555555555")
* .clientId("11111111-2222-3333-4444-555555555555")
* .identityTokenAudience("")
* .identityTokenTtl("")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:AuthBackend
* properties:
* type: azure
* identityTokenKey: example-key
* exampleAuthBackendConfig:
* type: vault:azure:AuthBackendConfig
* name: example
* properties:
* backend: ${example.path}
* tenantId: 11111111-2222-3333-4444-555555555555
* clientId: 11111111-2222-3333-4444-555555555555
* identityTokenAudience:
* identityTokenTtl:
* ```
*
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.AuthBackend("example", {type: "azure"});
* const exampleAuthBackendConfig = new vault.azure.AuthBackendConfig("example", {
* backend: example.path,
* tenantId: "11111111-2222-3333-4444-555555555555",
* clientId: "11111111-2222-3333-4444-555555555555",
* clientSecret: "01234567890123456789",
* resource: "https://vault.hashicorp.com",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.AuthBackend("example", type="azure")
* example_auth_backend_config = vault.azure.AuthBackendConfig("example",
* backend=example.path,
* tenant_id="11111111-2222-3333-4444-555555555555",
* client_id="11111111-2222-3333-4444-555555555555",
* client_secret="01234567890123456789",
* resource="https://vault.hashicorp.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.AuthBackend("example", new()
* {
* Type = "azure",
* });
* var exampleAuthBackendConfig = new Vault.Azure.AuthBackendConfig("example", new()
* {
* Backend = example.Path,
* TenantId = "11111111-2222-3333-4444-555555555555",
* ClientId = "11111111-2222-3333-4444-555555555555",
* ClientSecret = "01234567890123456789",
* Resource = "https://vault.hashicorp.com",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/azure"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := vault.NewAuthBackend(ctx, "example", &vault.AuthBackendArgs{
* Type: pulumi.String("azure"),
* })
* if err != nil {
* return err
* }
* _, err = azure.NewAuthBackendConfig(ctx, "example", &azure.AuthBackendConfigArgs{
* Backend: example.Path,
* TenantId: pulumi.String("11111111-2222-3333-4444-555555555555"),
* ClientId: pulumi.String("11111111-2222-3333-4444-555555555555"),
* ClientSecret: pulumi.String("01234567890123456789"),
* Resource: pulumi.String("https://vault.hashicorp.com"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.azure.AuthBackendConfig;
* import com.pulumi.vault.azure.AuthBackendConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AuthBackend("example", AuthBackendArgs.builder()
* .type("azure")
* .build());
* var exampleAuthBackendConfig = new AuthBackendConfig("exampleAuthBackendConfig", AuthBackendConfigArgs.builder()
* .backend(example.path())
* .tenantId("11111111-2222-3333-4444-555555555555")
* .clientId("11111111-2222-3333-4444-555555555555")
* .clientSecret("01234567890123456789")
* .resource("https://vault.hashicorp.com")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:AuthBackend
* properties:
* type: azure
* exampleAuthBackendConfig:
* type: vault:azure:AuthBackendConfig
* name: example
* properties:
* backend: ${example.path}
* tenantId: 11111111-2222-3333-4444-555555555555
* clientId: 11111111-2222-3333-4444-555555555555
* clientSecret: '01234567890123456789'
* resource: https://vault.hashicorp.com
* ```
*
* ## Import
* Azure auth backends can be imported using `auth/`, the `backend` path, and `/config` e.g.
* ```sh
* $ pulumi import vault:azure/authBackendConfig:AuthBackendConfig example auth/azure/config
* ```
*/
public class AuthBackendConfig internal constructor(
override val javaResource: com.pulumi.vault.azure.AuthBackendConfig,
) : KotlinCustomResource(javaResource, AuthBackendConfigMapper) {
/**
* The path the Azure auth backend being configured was
* mounted at. Defaults to `azure`.
*/
public val backend: Output?
get() = javaResource.backend().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The client id for credentials to query the Azure APIs.
* Currently read permissions to query compute resources are required.
*/
public val clientId: Output?
get() = javaResource.clientId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The client secret for credentials to query the
* Azure APIs.
*/
public val clientSecret: Output?
get() = javaResource.clientSecret().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Azure cloud environment. Valid values:
* AzurePublicCloud, AzureUSGovernmentCloud, AzureChinaCloud,
* AzureGermanCloud. Defaults to `AzurePublicCloud`.
*/
public val environment: Output?
get() = javaResource.environment().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The audience claim value for plugin identity tokens. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
public val identityTokenAudience: Output?
get() = javaResource.identityTokenAudience().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The TTL of generated identity tokens in seconds.
*/
public val identityTokenTtl: Output
get() = javaResource.identityTokenTtl().applyValue({ args0 -> args0 })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The configured URL for the application registered in
* Azure Active Directory.
*/
public val resource: Output
get() = javaResource.resource().applyValue({ args0 -> args0 })
/**
* The tenant id for the Azure Active Directory
* organization.
*/
public val tenantId: Output
get() = javaResource.tenantId().applyValue({ args0 -> args0 })
}
public object AuthBackendConfigMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.azure.AuthBackendConfig::class == javaResource::class
override fun map(javaResource: Resource): AuthBackendConfig = AuthBackendConfig(
javaResource as
com.pulumi.vault.azure.AuthBackendConfig,
)
}
/**
* @see [AuthBackendConfig].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AuthBackendConfig].
*/
public suspend fun authBackendConfig(
name: String,
block: suspend AuthBackendConfigResourceBuilder.() -> Unit,
): AuthBackendConfig {
val builder = AuthBackendConfigResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AuthBackendConfig].
* @param name The _unique_ name of the resulting resource.
*/
public fun authBackendConfig(name: String): AuthBackendConfig {
val builder = AuthBackendConfigResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy