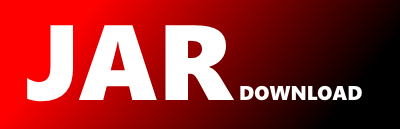
com.pulumi.vault.azure.kotlin.BackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.azure.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.azure.BackendArgs.builder
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ### *Vault-1.9 And Above*
* You can setup the Azure secrets engine with Workload Identity Federation (WIF) for a secret-less configuration:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const azure = new vault.azure.Backend("azure", {
* subscriptionId: "11111111-2222-3333-4444-111111111111",
* tenantId: "11111111-2222-3333-4444-222222222222",
* clientId: "11111111-2222-3333-4444-333333333333",
* identityTokenAudience: "",
* identityTokenTtl: "",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* azure = vault.azure.Backend("azure",
* subscription_id="11111111-2222-3333-4444-111111111111",
* tenant_id="11111111-2222-3333-4444-222222222222",
* client_id="11111111-2222-3333-4444-333333333333",
* identity_token_audience="",
* identity_token_ttl="")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var azure = new Vault.Azure.Backend("azure", new()
* {
* SubscriptionId = "11111111-2222-3333-4444-111111111111",
* TenantId = "11111111-2222-3333-4444-222222222222",
* ClientId = "11111111-2222-3333-4444-333333333333",
* IdentityTokenAudience = "",
* IdentityTokenTtl = "",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/azure"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := azure.NewBackend(ctx, "azure", &azure.BackendArgs{
* SubscriptionId: pulumi.String("11111111-2222-3333-4444-111111111111"),
* TenantId: pulumi.String("11111111-2222-3333-4444-222222222222"),
* ClientId: pulumi.String("11111111-2222-3333-4444-333333333333"),
* IdentityTokenAudience: pulumi.String(""),
* IdentityTokenTtl: pulumi.Int(""),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.azure.Backend;
* import com.pulumi.vault.azure.BackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var azure = new Backend("azure", BackendArgs.builder()
* .subscriptionId("11111111-2222-3333-4444-111111111111")
* .tenantId("11111111-2222-3333-4444-222222222222")
* .clientId("11111111-2222-3333-4444-333333333333")
* .identityTokenAudience("")
* .identityTokenTtl("")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* azure:
* type: vault:azure:Backend
* properties:
* subscriptionId: 11111111-2222-3333-4444-111111111111
* tenantId: 11111111-2222-3333-4444-222222222222
* clientId: 11111111-2222-3333-4444-333333333333
* identityTokenAudience:
* identityTokenTtl:
* ```
*
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const azure = new vault.azure.Backend("azure", {
* useMicrosoftGraphApi: true,
* subscriptionId: "11111111-2222-3333-4444-111111111111",
* tenantId: "11111111-2222-3333-4444-222222222222",
* clientId: "11111111-2222-3333-4444-333333333333",
* clientSecret: "12345678901234567890",
* environment: "AzurePublicCloud",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* azure = vault.azure.Backend("azure",
* use_microsoft_graph_api=True,
* subscription_id="11111111-2222-3333-4444-111111111111",
* tenant_id="11111111-2222-3333-4444-222222222222",
* client_id="11111111-2222-3333-4444-333333333333",
* client_secret="12345678901234567890",
* environment="AzurePublicCloud")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var azure = new Vault.Azure.Backend("azure", new()
* {
* UseMicrosoftGraphApi = true,
* SubscriptionId = "11111111-2222-3333-4444-111111111111",
* TenantId = "11111111-2222-3333-4444-222222222222",
* ClientId = "11111111-2222-3333-4444-333333333333",
* ClientSecret = "12345678901234567890",
* Environment = "AzurePublicCloud",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/azure"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := azure.NewBackend(ctx, "azure", &azure.BackendArgs{
* UseMicrosoftGraphApi: pulumi.Bool(true),
* SubscriptionId: pulumi.String("11111111-2222-3333-4444-111111111111"),
* TenantId: pulumi.String("11111111-2222-3333-4444-222222222222"),
* ClientId: pulumi.String("11111111-2222-3333-4444-333333333333"),
* ClientSecret: pulumi.String("12345678901234567890"),
* Environment: pulumi.String("AzurePublicCloud"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.azure.Backend;
* import com.pulumi.vault.azure.BackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var azure = new Backend("azure", BackendArgs.builder()
* .useMicrosoftGraphApi(true)
* .subscriptionId("11111111-2222-3333-4444-111111111111")
* .tenantId("11111111-2222-3333-4444-222222222222")
* .clientId("11111111-2222-3333-4444-333333333333")
* .clientSecret("12345678901234567890")
* .environment("AzurePublicCloud")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* azure:
* type: vault:azure:Backend
* properties:
* useMicrosoftGraphApi: true
* subscriptionId: 11111111-2222-3333-4444-111111111111
* tenantId: 11111111-2222-3333-4444-222222222222
* clientId: 11111111-2222-3333-4444-333333333333
* clientSecret: '12345678901234567890'
* environment: AzurePublicCloud
* ```
*
* ### *Vault-1.8 And Below*
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const azure = new vault.azure.Backend("azure", {
* useMicrosoftGraphApi: false,
* subscriptionId: "11111111-2222-3333-4444-111111111111",
* tenantId: "11111111-2222-3333-4444-222222222222",
* clientId: "11111111-2222-3333-4444-333333333333",
* clientSecret: "12345678901234567890",
* environment: "AzurePublicCloud",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* azure = vault.azure.Backend("azure",
* use_microsoft_graph_api=False,
* subscription_id="11111111-2222-3333-4444-111111111111",
* tenant_id="11111111-2222-3333-4444-222222222222",
* client_id="11111111-2222-3333-4444-333333333333",
* client_secret="12345678901234567890",
* environment="AzurePublicCloud")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var azure = new Vault.Azure.Backend("azure", new()
* {
* UseMicrosoftGraphApi = false,
* SubscriptionId = "11111111-2222-3333-4444-111111111111",
* TenantId = "11111111-2222-3333-4444-222222222222",
* ClientId = "11111111-2222-3333-4444-333333333333",
* ClientSecret = "12345678901234567890",
* Environment = "AzurePublicCloud",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/azure"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := azure.NewBackend(ctx, "azure", &azure.BackendArgs{
* UseMicrosoftGraphApi: pulumi.Bool(false),
* SubscriptionId: pulumi.String("11111111-2222-3333-4444-111111111111"),
* TenantId: pulumi.String("11111111-2222-3333-4444-222222222222"),
* ClientId: pulumi.String("11111111-2222-3333-4444-333333333333"),
* ClientSecret: pulumi.String("12345678901234567890"),
* Environment: pulumi.String("AzurePublicCloud"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.azure.Backend;
* import com.pulumi.vault.azure.BackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var azure = new Backend("azure", BackendArgs.builder()
* .useMicrosoftGraphApi(false)
* .subscriptionId("11111111-2222-3333-4444-111111111111")
* .tenantId("11111111-2222-3333-4444-222222222222")
* .clientId("11111111-2222-3333-4444-333333333333")
* .clientSecret("12345678901234567890")
* .environment("AzurePublicCloud")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* azure:
* type: vault:azure:Backend
* properties:
* useMicrosoftGraphApi: false
* subscriptionId: 11111111-2222-3333-4444-111111111111
* tenantId: 11111111-2222-3333-4444-222222222222
* clientId: 11111111-2222-3333-4444-333333333333
* clientSecret: '12345678901234567890'
* environment: AzurePublicCloud
* ```
*
* @property clientId The OAuth2 client id to connect to Azure.
* @property clientSecret The OAuth2 client secret to connect to Azure.
* @property description Human-friendly description of the mount for the backend.
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property environment The Azure environment.
* @property identityTokenAudience The audience claim value. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
* @property identityTokenKey The key to use for signing identity tokens. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
* @property identityTokenTtl The TTL of generated identity tokens in seconds. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path The unique path this backend should be mounted at. Defaults to `azure`.
* @property subscriptionId The subscription id for the Azure Active Directory.
* @property tenantId The tenant id for the Azure Active Directory.
* @property useMicrosoftGraphApi Use the Microsoft Graph API. Should be set to true on vault-1.10+
*/
public data class BackendArgs(
public val clientId: Output? = null,
public val clientSecret: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val environment: Output? = null,
public val identityTokenAudience: Output? = null,
public val identityTokenKey: Output? = null,
public val identityTokenTtl: Output? = null,
public val namespace: Output? = null,
public val path: Output? = null,
public val subscriptionId: Output? = null,
public val tenantId: Output? = null,
@Deprecated(
message = """
This field is not supported in Vault-1.12+ and is the default behavior. This field will be removed
in future version of the provider.
""",
)
public val useMicrosoftGraphApi: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.azure.BackendArgs =
com.pulumi.vault.azure.BackendArgs.builder()
.clientId(clientId?.applyValue({ args0 -> args0 }))
.clientSecret(clientSecret?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.environment(environment?.applyValue({ args0 -> args0 }))
.identityTokenAudience(identityTokenAudience?.applyValue({ args0 -> args0 }))
.identityTokenKey(identityTokenKey?.applyValue({ args0 -> args0 }))
.identityTokenTtl(identityTokenTtl?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.subscriptionId(subscriptionId?.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 }))
.useMicrosoftGraphApi(useMicrosoftGraphApi?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackendArgs].
*/
@PulumiTagMarker
public class BackendArgsBuilder internal constructor() {
private var clientId: Output? = null
private var clientSecret: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var environment: Output? = null
private var identityTokenAudience: Output? = null
private var identityTokenKey: Output? = null
private var identityTokenTtl: Output? = null
private var namespace: Output? = null
private var path: Output? = null
private var subscriptionId: Output? = null
private var tenantId: Output? = null
private var useMicrosoftGraphApi: Output? = null
/**
* @param value The OAuth2 client id to connect to Azure.
*/
@JvmName("jvspjmstmjbfetho")
public suspend fun clientId(`value`: Output) {
this.clientId = value
}
/**
* @param value The OAuth2 client secret to connect to Azure.
*/
@JvmName("cpdwxjaswtmetdtn")
public suspend fun clientSecret(`value`: Output) {
this.clientSecret = value
}
/**
* @param value Human-friendly description of the mount for the backend.
*/
@JvmName("gvaynuxovgpbxlhw")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("aqigknceubggyscr")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value The Azure environment.
*/
@JvmName("ermjvllqwlvysbjs")
public suspend fun environment(`value`: Output) {
this.environment = value
}
/**
* @param value The audience claim value. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
@JvmName("sgficnfcsntqcdfv")
public suspend fun identityTokenAudience(`value`: Output) {
this.identityTokenAudience = value
}
/**
* @param value The key to use for signing identity tokens. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
@JvmName("aqrsdwitwjgojgix")
public suspend fun identityTokenKey(`value`: Output) {
this.identityTokenKey = value
}
/**
* @param value The TTL of generated identity tokens in seconds. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
@JvmName("lfdgbrvaewktuveu")
public suspend fun identityTokenTtl(`value`: Output) {
this.identityTokenTtl = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("kpthwpvkfunkwchs")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value The unique path this backend should be mounted at. Defaults to `azure`.
*/
@JvmName("lfpunyjfneeqkcji")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value The subscription id for the Azure Active Directory.
*/
@JvmName("wtpmofodhnhlwayr")
public suspend fun subscriptionId(`value`: Output) {
this.subscriptionId = value
}
/**
* @param value The tenant id for the Azure Active Directory.
*/
@JvmName("jskitvkcssgomenh")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value Use the Microsoft Graph API. Should be set to true on vault-1.10+
*/
@Deprecated(
message = """
This field is not supported in Vault-1.12+ and is the default behavior. This field will be removed
in future version of the provider.
""",
)
@JvmName("jcfsgjrpcaspexte")
public suspend fun useMicrosoftGraphApi(`value`: Output) {
this.useMicrosoftGraphApi = value
}
/**
* @param value The OAuth2 client id to connect to Azure.
*/
@JvmName("alhcdviwgtaxaytu")
public suspend fun clientId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientId = mapped
}
/**
* @param value The OAuth2 client secret to connect to Azure.
*/
@JvmName("gpsgpmwjlxnnyjrr")
public suspend fun clientSecret(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientSecret = mapped
}
/**
* @param value Human-friendly description of the mount for the backend.
*/
@JvmName("jhnynarrthvsbsve")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("clvjsqeedsofuigl")
public suspend fun disableRemount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableRemount = mapped
}
/**
* @param value The Azure environment.
*/
@JvmName("yaxkadwniqprqnfq")
public suspend fun environment(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.environment = mapped
}
/**
* @param value The audience claim value. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
@JvmName("oljthpydjdulstje")
public suspend fun identityTokenAudience(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityTokenAudience = mapped
}
/**
* @param value The key to use for signing identity tokens. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
@JvmName("mbidjabjmculuyck")
public suspend fun identityTokenKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityTokenKey = mapped
}
/**
* @param value The TTL of generated identity tokens in seconds. Requires Vault 1.17+.
* *Available only for Vault Enterprise*
*/
@JvmName("nrnmqikontxvddgc")
public suspend fun identityTokenTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityTokenTtl = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("xdbedhprmnrbgwip")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value The unique path this backend should be mounted at. Defaults to `azure`.
*/
@JvmName("ykbwnyfdwueogpek")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value The subscription id for the Azure Active Directory.
*/
@JvmName("oitxccqgpmsmtuln")
public suspend fun subscriptionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriptionId = mapped
}
/**
* @param value The tenant id for the Azure Active Directory.
*/
@JvmName("oiajavmacplwqggi")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
/**
* @param value Use the Microsoft Graph API. Should be set to true on vault-1.10+
*/
@Deprecated(
message = """
This field is not supported in Vault-1.12+ and is the default behavior. This field will be removed
in future version of the provider.
""",
)
@JvmName("odjrloncfgfrhpei")
public suspend fun useMicrosoftGraphApi(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useMicrosoftGraphApi = mapped
}
internal fun build(): BackendArgs = BackendArgs(
clientId = clientId,
clientSecret = clientSecret,
description = description,
disableRemount = disableRemount,
environment = environment,
identityTokenAudience = identityTokenAudience,
identityTokenKey = identityTokenKey,
identityTokenTtl = identityTokenTtl,
namespace = namespace,
path = path,
subscriptionId = subscriptionId,
tenantId = tenantId,
useMicrosoftGraphApi = useMicrosoftGraphApi,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy