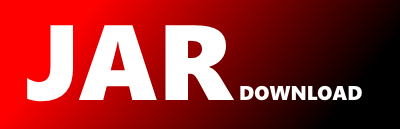
com.pulumi.vault.azure.kotlin.BackendRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.azure.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.azure.BackendRoleArgs.builder
import com.pulumi.vault.azure.kotlin.inputs.BackendRoleAzureGroupArgs
import com.pulumi.vault.azure.kotlin.inputs.BackendRoleAzureGroupArgsBuilder
import com.pulumi.vault.azure.kotlin.inputs.BackendRoleAzureRoleArgs
import com.pulumi.vault.azure.kotlin.inputs.BackendRoleAzureRoleArgsBuilder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const azure = new vault.azure.Backend("azure", {
* subscriptionId: subscriptionId,
* tenantId: tenantId,
* clientSecret: clientSecret,
* clientId: clientId,
* });
* const generatedRole = new vault.azure.BackendRole("generated_role", {
* backend: azure.path,
* role: "generated_role",
* signInAudience: "AzureADMyOrg",
* tags: [
* "team:engineering",
* "environment:development",
* ],
* ttl: "300",
* maxTtl: "600",
* azureRoles: [{
* roleName: "Reader",
* scope: `/subscriptions/${subscriptionId}/resourceGroups/azure-vault-group`,
* }],
* });
* const existingObjectId = new vault.azure.BackendRole("existing_object_id", {
* backend: azure.path,
* role: "existing_object_id",
* applicationObjectId: "11111111-2222-3333-4444-44444444444",
* ttl: "300",
* maxTtl: "600",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* azure = vault.azure.Backend("azure",
* subscription_id=subscription_id,
* tenant_id=tenant_id,
* client_secret=client_secret,
* client_id=client_id)
* generated_role = vault.azure.BackendRole("generated_role",
* backend=azure.path,
* role="generated_role",
* sign_in_audience="AzureADMyOrg",
* tags=[
* "team:engineering",
* "environment:development",
* ],
* ttl="300",
* max_ttl="600",
* azure_roles=[vault.azure.BackendRoleAzureRoleArgs(
* role_name="Reader",
* scope=f"/subscriptions/{subscription_id}/resourceGroups/azure-vault-group",
* )])
* existing_object_id = vault.azure.BackendRole("existing_object_id",
* backend=azure.path,
* role="existing_object_id",
* application_object_id="11111111-2222-3333-4444-44444444444",
* ttl="300",
* max_ttl="600")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var azure = new Vault.Azure.Backend("azure", new()
* {
* SubscriptionId = subscriptionId,
* TenantId = tenantId,
* ClientSecret = clientSecret,
* ClientId = clientId,
* });
* var generatedRole = new Vault.Azure.BackendRole("generated_role", new()
* {
* Backend = azure.Path,
* Role = "generated_role",
* SignInAudience = "AzureADMyOrg",
* Tags = new[]
* {
* "team:engineering",
* "environment:development",
* },
* Ttl = "300",
* MaxTtl = "600",
* AzureRoles = new[]
* {
* new Vault.Azure.Inputs.BackendRoleAzureRoleArgs
* {
* RoleName = "Reader",
* Scope = $"/subscriptions/{subscriptionId}/resourceGroups/azure-vault-group",
* },
* },
* });
* var existingObjectId = new Vault.Azure.BackendRole("existing_object_id", new()
* {
* Backend = azure.Path,
* Role = "existing_object_id",
* ApplicationObjectId = "11111111-2222-3333-4444-44444444444",
* Ttl = "300",
* MaxTtl = "600",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/azure"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* azure, err := azure.NewBackend(ctx, "azure", &azure.BackendArgs{
* SubscriptionId: pulumi.Any(subscriptionId),
* TenantId: pulumi.Any(tenantId),
* ClientSecret: pulumi.Any(clientSecret),
* ClientId: pulumi.Any(clientId),
* })
* if err != nil {
* return err
* }
* _, err = azure.NewBackendRole(ctx, "generated_role", &azure.BackendRoleArgs{
* Backend: azure.Path,
* Role: pulumi.String("generated_role"),
* SignInAudience: pulumi.String("AzureADMyOrg"),
* Tags: pulumi.StringArray{
* pulumi.String("team:engineering"),
* pulumi.String("environment:development"),
* },
* Ttl: pulumi.String("300"),
* MaxTtl: pulumi.String("600"),
* AzureRoles: azure.BackendRoleAzureRoleArray{
* &azure.BackendRoleAzureRoleArgs{
* RoleName: pulumi.String("Reader"),
* Scope: pulumi.String(fmt.Sprintf("/subscriptions/%v/resourceGroups/azure-vault-group", subscriptionId)),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = azure.NewBackendRole(ctx, "existing_object_id", &azure.BackendRoleArgs{
* Backend: azure.Path,
* Role: pulumi.String("existing_object_id"),
* ApplicationObjectId: pulumi.String("11111111-2222-3333-4444-44444444444"),
* Ttl: pulumi.String("300"),
* MaxTtl: pulumi.String("600"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.azure.Backend;
* import com.pulumi.vault.azure.BackendArgs;
* import com.pulumi.vault.azure.BackendRole;
* import com.pulumi.vault.azure.BackendRoleArgs;
* import com.pulumi.vault.azure.inputs.BackendRoleAzureRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var azure = new Backend("azure", BackendArgs.builder()
* .subscriptionId(subscriptionId)
* .tenantId(tenantId)
* .clientSecret(clientSecret)
* .clientId(clientId)
* .build());
* var generatedRole = new BackendRole("generatedRole", BackendRoleArgs.builder()
* .backend(azure.path())
* .role("generated_role")
* .signInAudience("AzureADMyOrg")
* .tags(
* "team:engineering",
* "environment:development")
* .ttl(300)
* .maxTtl(600)
* .azureRoles(BackendRoleAzureRoleArgs.builder()
* .roleName("Reader")
* .scope(String.format("/subscriptions/%s/resourceGroups/azure-vault-group", subscriptionId))
* .build())
* .build());
* var existingObjectId = new BackendRole("existingObjectId", BackendRoleArgs.builder()
* .backend(azure.path())
* .role("existing_object_id")
* .applicationObjectId("11111111-2222-3333-4444-44444444444")
* .ttl(300)
* .maxTtl(600)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* azure:
* type: vault:azure:Backend
* properties:
* subscriptionId: ${subscriptionId}
* tenantId: ${tenantId}
* clientSecret: ${clientSecret}
* clientId: ${clientId}
* generatedRole:
* type: vault:azure:BackendRole
* name: generated_role
* properties:
* backend: ${azure.path}
* role: generated_role
* signInAudience: AzureADMyOrg
* tags:
* - team:engineering
* - environment:development
* ttl: 300
* maxTtl: 600
* azureRoles:
* - roleName: Reader
* scope: /subscriptions/${subscriptionId}/resourceGroups/azure-vault-group
* existingObjectId:
* type: vault:azure:BackendRole
* name: existing_object_id
* properties:
* backend: ${azure.path}
* role: existing_object_id
* applicationObjectId: 11111111-2222-3333-4444-44444444444
* ttl: 300
* maxTtl: 600
* ```
*
* @property applicationObjectId Application Object ID for an existing service principal that will
* be used instead of creating dynamic service principals. If present, `azure_roles` and `permanently_delete` will be ignored.
* @property azureGroups List of Azure groups to be assigned to the generated service principal.
* @property azureRoles List of Azure roles to be assigned to the generated service principal.
* @property backend Path to the mounted Azure auth backend
* @property description Human-friendly description of the mount for the backend.
* @property maxTtl Specifies the maximum TTL for service principals generated using this role. Accepts time
* suffixed strings ("1h") or an integer number of seconds. Defaults to the system/engine max TTL time.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property permanentlyDelete Indicates whether the applications and service principals created by Vault will be permanently
* deleted when the corresponding leases expire. Defaults to `false`. For Vault v1.12+.
* @property role Name of the Azure role
* @property signInAudience Specifies the security principal types that are allowed to sign in to the application.
* Valid values are: AzureADMyOrg, AzureADMultipleOrgs, AzureADandPersonalMicrosoftAccount, PersonalMicrosoftAccount. Requires Vault 1.16+.
* @property tags A list of Azure tags to attach to an application. Requires Vault 1.16+.
* @property ttl Specifies the default TTL for service principals generated using this role.
* Accepts time suffixed strings ("1h") or an integer number of seconds. Defaults to the system/engine default TTL time.
*/
public data class BackendRoleArgs(
public val applicationObjectId: Output? = null,
public val azureGroups: Output>? = null,
public val azureRoles: Output>? = null,
public val backend: Output? = null,
public val description: Output? = null,
public val maxTtl: Output? = null,
public val namespace: Output? = null,
public val permanentlyDelete: Output? = null,
public val role: Output? = null,
public val signInAudience: Output? = null,
public val tags: Output>? = null,
public val ttl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.azure.BackendRoleArgs =
com.pulumi.vault.azure.BackendRoleArgs.builder()
.applicationObjectId(applicationObjectId?.applyValue({ args0 -> args0 }))
.azureGroups(
azureGroups?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.azureRoles(
azureRoles?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.backend(backend?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.maxTtl(maxTtl?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.permanentlyDelete(permanentlyDelete?.applyValue({ args0 -> args0 }))
.role(role?.applyValue({ args0 -> args0 }))
.signInAudience(signInAudience?.applyValue({ args0 -> args0 }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.ttl(ttl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackendRoleArgs].
*/
@PulumiTagMarker
public class BackendRoleArgsBuilder internal constructor() {
private var applicationObjectId: Output? = null
private var azureGroups: Output>? = null
private var azureRoles: Output>? = null
private var backend: Output? = null
private var description: Output? = null
private var maxTtl: Output? = null
private var namespace: Output? = null
private var permanentlyDelete: Output? = null
private var role: Output? = null
private var signInAudience: Output? = null
private var tags: Output>? = null
private var ttl: Output? = null
/**
* @param value Application Object ID for an existing service principal that will
* be used instead of creating dynamic service principals. If present, `azure_roles` and `permanently_delete` will be ignored.
*/
@JvmName("sfwaadjytklfpiss")
public suspend fun applicationObjectId(`value`: Output) {
this.applicationObjectId = value
}
/**
* @param value List of Azure groups to be assigned to the generated service principal.
*/
@JvmName("boavqkbkwnvmybmj")
public suspend fun azureGroups(`value`: Output>) {
this.azureGroups = value
}
@JvmName("dmqypybrhjklxnju")
public suspend fun azureGroups(vararg values: Output) {
this.azureGroups = Output.all(values.asList())
}
/**
* @param values List of Azure groups to be assigned to the generated service principal.
*/
@JvmName("vykihsughuouhuvl")
public suspend fun azureGroups(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy