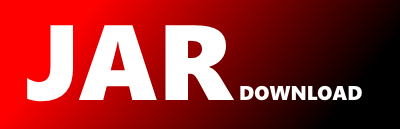
com.pulumi.vault.azure.kotlin.inputs.GetAccessCredentialsPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.azure.kotlin.inputs
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.azure.inputs.GetAccessCredentialsPlainArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getAccessCredentials.
* @property backend The path to the Azure secret backend to
* read credentials from, with no leading or trailing `/`s.
* @property environment The Azure environment to use during credential validation.
* Defaults to the environment configured in the Vault backend.
* Some possible values: `AzurePublicCloud`, `AzureGovernmentCloud`
* *See the caveats section for more information on this field.*
* @property maxCredValidationSeconds If 'validate_creds' is true,
* the number of seconds after which to give up validating credentials. Defaults
* to 300.
* @property namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property numSecondsBetweenTests If 'validate_creds' is true,
* the number of seconds to wait between each test of generated credentials.
* Defaults to 1.
* @property numSequentialSuccesses If 'validate_creds' is true,
* the number of sequential successes required to validate generated
* credentials. Defaults to 8.
* @property role The name of the Azure secret backend role to read
* credentials from, with no leading or trailing `/`s.
* @property subscriptionId The subscription ID to use during credential
* validation. Defaults to the subscription ID configured in the Vault `backend`.
* *See the caveats section for more information on this field.*
* @property tenantId The tenant ID to use during credential validation.
* Defaults to the tenant ID configured in the Vault `backend`.
* *See the caveats section for more information on this field.*
* @property validateCreds Whether generated credentials should be
* validated before being returned. Defaults to `false`, which returns
* credentials without checking whether they have fully propagated throughout
* Azure Active Directory. Designating `true` activates testing.
*/
public data class GetAccessCredentialsPlainArgs(
public val backend: String,
public val environment: String? = null,
public val maxCredValidationSeconds: Int? = null,
public val namespace: String? = null,
public val numSecondsBetweenTests: Int? = null,
public val numSequentialSuccesses: Int? = null,
public val role: String,
public val subscriptionId: String? = null,
public val tenantId: String? = null,
public val validateCreds: Boolean? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.azure.inputs.GetAccessCredentialsPlainArgs =
com.pulumi.vault.azure.inputs.GetAccessCredentialsPlainArgs.builder()
.backend(backend.let({ args0 -> args0 }))
.environment(environment?.let({ args0 -> args0 }))
.maxCredValidationSeconds(maxCredValidationSeconds?.let({ args0 -> args0 }))
.namespace(namespace?.let({ args0 -> args0 }))
.numSecondsBetweenTests(numSecondsBetweenTests?.let({ args0 -> args0 }))
.numSequentialSuccesses(numSequentialSuccesses?.let({ args0 -> args0 }))
.role(role.let({ args0 -> args0 }))
.subscriptionId(subscriptionId?.let({ args0 -> args0 }))
.tenantId(tenantId?.let({ args0 -> args0 }))
.validateCreds(validateCreds?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetAccessCredentialsPlainArgs].
*/
@PulumiTagMarker
public class GetAccessCredentialsPlainArgsBuilder internal constructor() {
private var backend: String? = null
private var environment: String? = null
private var maxCredValidationSeconds: Int? = null
private var namespace: String? = null
private var numSecondsBetweenTests: Int? = null
private var numSequentialSuccesses: Int? = null
private var role: String? = null
private var subscriptionId: String? = null
private var tenantId: String? = null
private var validateCreds: Boolean? = null
/**
* @param value The path to the Azure secret backend to
* read credentials from, with no leading or trailing `/`s.
*/
@JvmName("cpirsafgitygbsls")
public suspend fun backend(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.backend = mapped
}
/**
* @param value The Azure environment to use during credential validation.
* Defaults to the environment configured in the Vault backend.
* Some possible values: `AzurePublicCloud`, `AzureGovernmentCloud`
* *See the caveats section for more information on this field.*
*/
@JvmName("olylllobpywdleqn")
public suspend fun environment(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.environment = mapped
}
/**
* @param value If 'validate_creds' is true,
* the number of seconds after which to give up validating credentials. Defaults
* to 300.
*/
@JvmName("bdhlhhvyiulhljap")
public suspend fun maxCredValidationSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.maxCredValidationSeconds = mapped
}
/**
* @param value The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("krrxsbcwvbsskmug")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.namespace = mapped
}
/**
* @param value If 'validate_creds' is true,
* the number of seconds to wait between each test of generated credentials.
* Defaults to 1.
*/
@JvmName("bdwgjmlogbblttbf")
public suspend fun numSecondsBetweenTests(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.numSecondsBetweenTests = mapped
}
/**
* @param value If 'validate_creds' is true,
* the number of sequential successes required to validate generated
* credentials. Defaults to 8.
*/
@JvmName("xnpfkunqguloybdj")
public suspend fun numSequentialSuccesses(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.numSequentialSuccesses = mapped
}
/**
* @param value The name of the Azure secret backend role to read
* credentials from, with no leading or trailing `/`s.
*/
@JvmName("ljmwpnhunkmnsrke")
public suspend fun role(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.role = mapped
}
/**
* @param value The subscription ID to use during credential
* validation. Defaults to the subscription ID configured in the Vault `backend`.
* *See the caveats section for more information on this field.*
*/
@JvmName("yugelkujdjvspidt")
public suspend fun subscriptionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.subscriptionId = mapped
}
/**
* @param value The tenant ID to use during credential validation.
* Defaults to the tenant ID configured in the Vault `backend`.
* *See the caveats section for more information on this field.*
*/
@JvmName("lxgarvlkikskpdtr")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tenantId = mapped
}
/**
* @param value Whether generated credentials should be
* validated before being returned. Defaults to `false`, which returns
* credentials without checking whether they have fully propagated throughout
* Azure Active Directory. Designating `true` activates testing.
*/
@JvmName("mxguqkkdyulyyidn")
public suspend fun validateCreds(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.validateCreds = mapped
}
internal fun build(): GetAccessCredentialsPlainArgs = GetAccessCredentialsPlainArgs(
backend = backend ?: throw PulumiNullFieldException("backend"),
environment = environment,
maxCredValidationSeconds = maxCredValidationSeconds,
namespace = namespace,
numSecondsBetweenTests = numSecondsBetweenTests,
numSequentialSuccesses = numSequentialSuccesses,
role = role ?: throw PulumiNullFieldException("role"),
subscriptionId = subscriptionId,
tenantId = tenantId,
validateCreds = validateCreds,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy