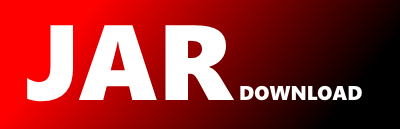
com.pulumi.vault.config.kotlin.UiCustomMessageArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.config.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.config.UiCustomMessageArgs.builder
import com.pulumi.vault.config.kotlin.inputs.UiCustomMessageLinkArgs
import com.pulumi.vault.config.kotlin.inputs.UiCustomMessageLinkArgsBuilder
import kotlin.Any
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property authenticated A flag indicating whether the custom message is displayed pre-login (false) or post-login (true)
* @property endTime The ending time of the active period of the custom message. Can be omitted for non-expiring message
* @property link A block containing a hyperlink associated with the custom message
* @property messageBase64 The base64-encoded content of the custom message
* @property namespace Target namespace. (requires Enterprise)
* @property options A map containing additional options for the custom message
* @property startTime The starting time of the active period of the custom message
* @property title The title of the custom message
* @property type The display type of custom message. Allowed values are banner and modal
*/
public data class UiCustomMessageArgs(
public val authenticated: Output? = null,
public val endTime: Output? = null,
public val link: Output? = null,
public val messageBase64: Output? = null,
public val namespace: Output? = null,
public val options: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy