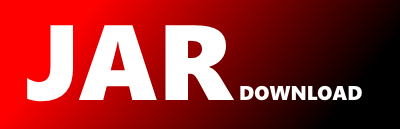
com.pulumi.vault.consul.kotlin.SecretBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.consul.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.consul.SecretBackendArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ### Creating a standard backend resource:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const test = new vault.consul.SecretBackend("test", {
* path: "consul",
* description: "Manages the Consul backend",
* address: "127.0.0.1:8500",
* token: "4240861b-ce3d-8530-115a-521ff070dd29",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* test = vault.consul.SecretBackend("test",
* path="consul",
* description="Manages the Consul backend",
* address="127.0.0.1:8500",
* token="4240861b-ce3d-8530-115a-521ff070dd29")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var test = new Vault.Consul.SecretBackend("test", new()
* {
* Path = "consul",
* Description = "Manages the Consul backend",
* Address = "127.0.0.1:8500",
* Token = "4240861b-ce3d-8530-115a-521ff070dd29",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/consul"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := consul.NewSecretBackend(ctx, "test", &consul.SecretBackendArgs{
* Path: pulumi.String("consul"),
* Description: pulumi.String("Manages the Consul backend"),
* Address: pulumi.String("127.0.0.1:8500"),
* Token: pulumi.String("4240861b-ce3d-8530-115a-521ff070dd29"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.consul.SecretBackend;
* import com.pulumi.vault.consul.SecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new SecretBackend("test", SecretBackendArgs.builder()
* .path("consul")
* .description("Manages the Consul backend")
* .address("127.0.0.1:8500")
* .token("4240861b-ce3d-8530-115a-521ff070dd29")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: vault:consul:SecretBackend
* properties:
* path: consul
* description: Manages the Consul backend
* address: 127.0.0.1:8500
* token: 4240861b-ce3d-8530-115a-521ff070dd29
* ```
*
* ### Creating a backend resource to bootstrap a new Consul instance:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const test = new vault.consul.SecretBackend("test", {
* path: "consul",
* description: "Bootstrap the Consul backend",
* address: "127.0.0.1:8500",
* bootstrap: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* test = vault.consul.SecretBackend("test",
* path="consul",
* description="Bootstrap the Consul backend",
* address="127.0.0.1:8500",
* bootstrap=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var test = new Vault.Consul.SecretBackend("test", new()
* {
* Path = "consul",
* Description = "Bootstrap the Consul backend",
* Address = "127.0.0.1:8500",
* Bootstrap = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/consul"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := consul.NewSecretBackend(ctx, "test", &consul.SecretBackendArgs{
* Path: pulumi.String("consul"),
* Description: pulumi.String("Bootstrap the Consul backend"),
* Address: pulumi.String("127.0.0.1:8500"),
* Bootstrap: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.consul.SecretBackend;
* import com.pulumi.vault.consul.SecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new SecretBackend("test", SecretBackendArgs.builder()
* .path("consul")
* .description("Bootstrap the Consul backend")
* .address("127.0.0.1:8500")
* .bootstrap(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: vault:consul:SecretBackend
* properties:
* path: consul
* description: Bootstrap the Consul backend
* address: 127.0.0.1:8500
* bootstrap: true
* ```
*
* ## Import
* Consul secret backends can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:consul/secretBackend:SecretBackend example consul
* ```
* @property address Specifies the address of the Consul instance, provided as "host:port" like "127.0.0.1:8500".
* @property bootstrap Denotes a backend resource that is used to bootstrap the Consul ACL system. Only one resource may be used to bootstrap.
* @property caCert CA certificate to use when verifying Consul server certificate, must be x509 PEM encoded.
* @property clientCert Client certificate used for Consul's TLS communication, must be x509 PEM encoded and if
* this is set you need to also set client_key.
* @property clientKey Client key used for Consul's TLS communication, must be x509 PEM encoded and if this is set
* you need to also set client_cert.
* @property defaultLeaseTtlSeconds The default TTL for credentials issued by this backend.
* @property description A human-friendly description for this backend.
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property local Specifies if the secret backend is local only.
* @property maxLeaseTtlSeconds The maximum TTL that can be requested
* for credentials issued by this backend.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path The unique location this backend should be mounted at. Must not begin or end with a `/`. Defaults
* to `consul`.
* @property scheme Specifies the URL scheme to use. Defaults to `http`.
* @property token Specifies the Consul token to use when managing or issuing new tokens.
*/
public data class SecretBackendArgs(
public val address: Output? = null,
public val bootstrap: Output? = null,
public val caCert: Output? = null,
public val clientCert: Output? = null,
public val clientKey: Output? = null,
public val defaultLeaseTtlSeconds: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val local: Output? = null,
public val maxLeaseTtlSeconds: Output? = null,
public val namespace: Output? = null,
public val path: Output? = null,
public val scheme: Output? = null,
public val token: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.consul.SecretBackendArgs =
com.pulumi.vault.consul.SecretBackendArgs.builder()
.address(address?.applyValue({ args0 -> args0 }))
.bootstrap(bootstrap?.applyValue({ args0 -> args0 }))
.caCert(caCert?.applyValue({ args0 -> args0 }))
.clientCert(clientCert?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.defaultLeaseTtlSeconds(defaultLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.local(local?.applyValue({ args0 -> args0 }))
.maxLeaseTtlSeconds(maxLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.scheme(scheme?.applyValue({ args0 -> args0 }))
.token(token?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendArgs].
*/
@PulumiTagMarker
public class SecretBackendArgsBuilder internal constructor() {
private var address: Output? = null
private var bootstrap: Output? = null
private var caCert: Output? = null
private var clientCert: Output? = null
private var clientKey: Output? = null
private var defaultLeaseTtlSeconds: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var local: Output? = null
private var maxLeaseTtlSeconds: Output? = null
private var namespace: Output? = null
private var path: Output? = null
private var scheme: Output? = null
private var token: Output? = null
/**
* @param value Specifies the address of the Consul instance, provided as "host:port" like "127.0.0.1:8500".
*/
@JvmName("lsjtgmwhuybioery")
public suspend fun address(`value`: Output) {
this.address = value
}
/**
* @param value Denotes a backend resource that is used to bootstrap the Consul ACL system. Only one resource may be used to bootstrap.
*/
@JvmName("ewpeslabmnnnkjvn")
public suspend fun bootstrap(`value`: Output) {
this.bootstrap = value
}
/**
* @param value CA certificate to use when verifying Consul server certificate, must be x509 PEM encoded.
*/
@JvmName("jnarkxofqbwdogwc")
public suspend fun caCert(`value`: Output) {
this.caCert = value
}
/**
* @param value Client certificate used for Consul's TLS communication, must be x509 PEM encoded and if
* this is set you need to also set client_key.
*/
@JvmName("fyjcxayhhkbthcpu")
public suspend fun clientCert(`value`: Output) {
this.clientCert = value
}
/**
* @param value Client key used for Consul's TLS communication, must be x509 PEM encoded and if this is set
* you need to also set client_cert.
*/
@JvmName("halgfqicmmgontan")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value The default TTL for credentials issued by this backend.
*/
@JvmName("svagnphirthhddpm")
public suspend fun defaultLeaseTtlSeconds(`value`: Output) {
this.defaultLeaseTtlSeconds = value
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("tmubygnhpaiwoktu")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("wbrlntxxdjtqxqmq")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value Specifies if the secret backend is local only.
*/
@JvmName("dwllwgftfbrbufye")
public suspend fun local(`value`: Output) {
this.local = value
}
/**
* @param value The maximum TTL that can be requested
* for credentials issued by this backend.
*/
@JvmName("puqjrmgslhqpbbvm")
public suspend fun maxLeaseTtlSeconds(`value`: Output) {
this.maxLeaseTtlSeconds = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("qwxolrlrwyxabmcp")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value The unique location this backend should be mounted at. Must not begin or end with a `/`. Defaults
* to `consul`.
*/
@JvmName("wittrqsbopwxihlq")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value Specifies the URL scheme to use. Defaults to `http`.
*/
@JvmName("ffbdigcnlxitbfsh")
public suspend fun scheme(`value`: Output) {
this.scheme = value
}
/**
* @param value Specifies the Consul token to use when managing or issuing new tokens.
*/
@JvmName("spxeniytydklbclq")
public suspend fun token(`value`: Output) {
this.token = value
}
/**
* @param value Specifies the address of the Consul instance, provided as "host:port" like "127.0.0.1:8500".
*/
@JvmName("fjxruanywpurndis")
public suspend fun address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address = mapped
}
/**
* @param value Denotes a backend resource that is used to bootstrap the Consul ACL system. Only one resource may be used to bootstrap.
*/
@JvmName("jlchqblaokykhwsb")
public suspend fun bootstrap(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bootstrap = mapped
}
/**
* @param value CA certificate to use when verifying Consul server certificate, must be x509 PEM encoded.
*/
@JvmName("pcxqnbvayhffhpug")
public suspend fun caCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCert = mapped
}
/**
* @param value Client certificate used for Consul's TLS communication, must be x509 PEM encoded and if
* this is set you need to also set client_key.
*/
@JvmName("stcfwkfcyhwmself")
public suspend fun clientCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCert = mapped
}
/**
* @param value Client key used for Consul's TLS communication, must be x509 PEM encoded and if this is set
* you need to also set client_cert.
*/
@JvmName("agiuhsrpchxvakbu")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value The default TTL for credentials issued by this backend.
*/
@JvmName("gvomdsyoindkghsr")
public suspend fun defaultLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultLeaseTtlSeconds = mapped
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("pvcoucgynqnduvdk")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("pndebaovbfskevni")
public suspend fun disableRemount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableRemount = mapped
}
/**
* @param value Specifies if the secret backend is local only.
*/
@JvmName("tccfuscujcljpmys")
public suspend fun local(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.local = mapped
}
/**
* @param value The maximum TTL that can be requested
* for credentials issued by this backend.
*/
@JvmName("clcgaiaajsuhgshk")
public suspend fun maxLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxLeaseTtlSeconds = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("yhcuybksllbndxjr")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value The unique location this backend should be mounted at. Must not begin or end with a `/`. Defaults
* to `consul`.
*/
@JvmName("qjyfnukcvmgpaewd")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value Specifies the URL scheme to use. Defaults to `http`.
*/
@JvmName("fcnywpegyxxqvhua")
public suspend fun scheme(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheme = mapped
}
/**
* @param value Specifies the Consul token to use when managing or issuing new tokens.
*/
@JvmName("jnfffsffgtbjsnjo")
public suspend fun token(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.token = mapped
}
internal fun build(): SecretBackendArgs = SecretBackendArgs(
address = address,
bootstrap = bootstrap,
caCert = caCert,
clientCert = clientCert,
clientKey = clientKey,
defaultLeaseTtlSeconds = defaultLeaseTtlSeconds,
description = description,
disableRemount = disableRemount,
local = local,
maxLeaseTtlSeconds = maxLeaseTtlSeconds,
namespace = namespace,
path = path,
scheme = scheme,
token = token,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy