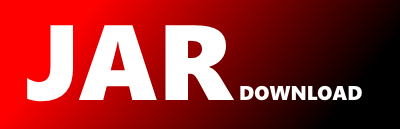
com.pulumi.vault.consul.kotlin.SecretBackendRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.consul.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.consul.SecretBackendRoleArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Consul secrets role for a Consul secrets engine in Vault. Consul secret backends can then issue Consul tokens.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const test = new vault.consul.SecretBackend("test", {
* path: "consul",
* description: "Manages the Consul backend",
* address: "127.0.0.1:8500",
* token: "4240861b-ce3d-8530-115a-521ff070dd29",
* });
* const example = new vault.consul.SecretBackendRole("example", {
* name: "test-role",
* backend: test.path,
* consulPolicies: ["example-policy"],
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* test = vault.consul.SecretBackend("test",
* path="consul",
* description="Manages the Consul backend",
* address="127.0.0.1:8500",
* token="4240861b-ce3d-8530-115a-521ff070dd29")
* example = vault.consul.SecretBackendRole("example",
* name="test-role",
* backend=test.path,
* consul_policies=["example-policy"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var test = new Vault.Consul.SecretBackend("test", new()
* {
* Path = "consul",
* Description = "Manages the Consul backend",
* Address = "127.0.0.1:8500",
* Token = "4240861b-ce3d-8530-115a-521ff070dd29",
* });
* var example = new Vault.Consul.SecretBackendRole("example", new()
* {
* Name = "test-role",
* Backend = test.Path,
* ConsulPolicies = new[]
* {
* "example-policy",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/consul"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := consul.NewSecretBackend(ctx, "test", &consul.SecretBackendArgs{
* Path: pulumi.String("consul"),
* Description: pulumi.String("Manages the Consul backend"),
* Address: pulumi.String("127.0.0.1:8500"),
* Token: pulumi.String("4240861b-ce3d-8530-115a-521ff070dd29"),
* })
* if err != nil {
* return err
* }
* _, err = consul.NewSecretBackendRole(ctx, "example", &consul.SecretBackendRoleArgs{
* Name: pulumi.String("test-role"),
* Backend: test.Path,
* ConsulPolicies: pulumi.StringArray{
* pulumi.String("example-policy"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.consul.SecretBackend;
* import com.pulumi.vault.consul.SecretBackendArgs;
* import com.pulumi.vault.consul.SecretBackendRole;
* import com.pulumi.vault.consul.SecretBackendRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new SecretBackend("test", SecretBackendArgs.builder()
* .path("consul")
* .description("Manages the Consul backend")
* .address("127.0.0.1:8500")
* .token("4240861b-ce3d-8530-115a-521ff070dd29")
* .build());
* var example = new SecretBackendRole("example", SecretBackendRoleArgs.builder()
* .name("test-role")
* .backend(test.path())
* .consulPolicies("example-policy")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: vault:consul:SecretBackend
* properties:
* path: consul
* description: Manages the Consul backend
* address: 127.0.0.1:8500
* token: 4240861b-ce3d-8530-115a-521ff070dd29
* example:
* type: vault:consul:SecretBackendRole
* properties:
* name: test-role
* backend: ${test.path}
* consulPolicies:
* - example-policy
* ```
*
* ## Note About Required Arguments
* *At least one* of the four arguments `consul_policies`, `consul_roles`, `service_identities`, or
* `node_identities` is required for a token. If desired, any combination of the four arguments up-to and
* including all four, is valid.
* ## Import
* Consul secret backend roles can be imported using the `backend`, `/roles/`, and the `name` e.g.
* ```sh
* $ pulumi import vault:consul/secretBackendRole:SecretBackendRole example consul/roles/my-role
* ```
* @property backend The unique name of an existing Consul secrets backend mount. Must not begin or end with a `/`. One of `path` or `backend` is required.
* @property consulNamespace The Consul namespace that the token will be created in.
* Applicable for Vault 1.10+ and Consul 1.7+".
* @property consulPolicies SEE NOTE The list of Consul ACL policies to associate with these roles.
* @property consulRoles SEE NOTE Set of Consul roles to attach to the token.
* Applicable for Vault 1.10+ with Consul 1.5+.
* @property local Indicates that the token should not be replicated globally and instead be local to the current datacenter.
* @property maxTtl Maximum TTL for leases associated with this role, in seconds.
* @property name The name of the Consul secrets engine role to create.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property nodeIdentities SEE NOTE Set of Consul node
* identities to attach to the token. Applicable for Vault 1.11+ with Consul 1.8+.
* @property partition The admin partition that the token will be created in.
* Applicable for Vault 1.10+ and Consul 1.11+".
* @property policies The list of Consul ACL policies to associate with these roles.
* **NOTE:** The new parameter `consul_policies` should be used in favor of this. This parameter,
* `policies`, remains supported for legacy users, but Vault has deprecated this field.
* @property serviceIdentities SEE NOTE Set of Consul
* service identities to attach to the token. Applicable for Vault 1.11+ with Consul 1.5+.
* @property ttl Specifies the TTL for this role.
*/
public data class SecretBackendRoleArgs(
public val backend: Output? = null,
public val consulNamespace: Output? = null,
public val consulPolicies: Output>? = null,
public val consulRoles: Output>? = null,
public val local: Output? = null,
public val maxTtl: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val nodeIdentities: Output>? = null,
public val partition: Output? = null,
public val policies: Output>? = null,
public val serviceIdentities: Output>? = null,
public val ttl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.consul.SecretBackendRoleArgs =
com.pulumi.vault.consul.SecretBackendRoleArgs.builder()
.backend(backend?.applyValue({ args0 -> args0 }))
.consulNamespace(consulNamespace?.applyValue({ args0 -> args0 }))
.consulPolicies(consulPolicies?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.consulRoles(consulRoles?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.local(local?.applyValue({ args0 -> args0 }))
.maxTtl(maxTtl?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.nodeIdentities(nodeIdentities?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.partition(partition?.applyValue({ args0 -> args0 }))
.policies(policies?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.serviceIdentities(serviceIdentities?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.ttl(ttl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendRoleArgs].
*/
@PulumiTagMarker
public class SecretBackendRoleArgsBuilder internal constructor() {
private var backend: Output? = null
private var consulNamespace: Output? = null
private var consulPolicies: Output>? = null
private var consulRoles: Output>? = null
private var local: Output? = null
private var maxTtl: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var nodeIdentities: Output>? = null
private var partition: Output? = null
private var policies: Output>? = null
private var serviceIdentities: Output>? = null
private var ttl: Output? = null
/**
* @param value The unique name of an existing Consul secrets backend mount. Must not begin or end with a `/`. One of `path` or `backend` is required.
*/
@JvmName("cmyhldxpfwrpdicg")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value The Consul namespace that the token will be created in.
* Applicable for Vault 1.10+ and Consul 1.7+".
*/
@JvmName("dhjqqeksfwetnptw")
public suspend fun consulNamespace(`value`: Output) {
this.consulNamespace = value
}
/**
* @param value SEE NOTE The list of Consul ACL policies to associate with these roles.
*/
@JvmName("ksdhhqfavvplylbf")
public suspend fun consulPolicies(`value`: Output>) {
this.consulPolicies = value
}
@JvmName("tshvuwcjsfmkqycv")
public suspend fun consulPolicies(vararg values: Output) {
this.consulPolicies = Output.all(values.asList())
}
/**
* @param values SEE NOTE The list of Consul ACL policies to associate with these roles.
*/
@JvmName("fxcbdxpidegxbfym")
public suspend fun consulPolicies(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy