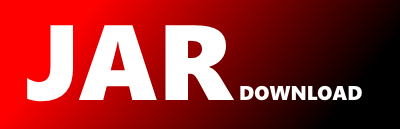
com.pulumi.vault.database.kotlin.inputs.SecretBackendConnectionElasticsearchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.database.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.database.inputs.SecretBackendConnectionElasticsearchArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property caCert The path to a PEM-encoded CA cert file to use to verify the Elasticsearch server's identity
* @property caPath The path to a directory of PEM-encoded CA cert files to use to verify the Elasticsearch server's identity
* @property clientCert The path to the certificate for the Elasticsearch client to present for communication
* @property clientKey The path to the key for the Elasticsearch client to use for communication
* @property insecure Whether to disable certificate verification
* @property password The password to be used in the connection URL
* @property tlsServerName This, if set, is used to set the SNI host when connecting via TLS
* @property url The URL for Elasticsearch's API
* @property username The username to be used in the connection URL
* @property usernameTemplate Template describing how dynamic usernames are generated.
*/
public data class SecretBackendConnectionElasticsearchArgs(
public val caCert: Output? = null,
public val caPath: Output? = null,
public val clientCert: Output? = null,
public val clientKey: Output? = null,
public val insecure: Output? = null,
public val password: Output,
public val tlsServerName: Output? = null,
public val url: Output,
public val username: Output,
public val usernameTemplate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.database.inputs.SecretBackendConnectionElasticsearchArgs =
com.pulumi.vault.database.inputs.SecretBackendConnectionElasticsearchArgs.builder()
.caCert(caCert?.applyValue({ args0 -> args0 }))
.caPath(caPath?.applyValue({ args0 -> args0 }))
.clientCert(clientCert?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.insecure(insecure?.applyValue({ args0 -> args0 }))
.password(password.applyValue({ args0 -> args0 }))
.tlsServerName(tlsServerName?.applyValue({ args0 -> args0 }))
.url(url.applyValue({ args0 -> args0 }))
.username(username.applyValue({ args0 -> args0 }))
.usernameTemplate(usernameTemplate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendConnectionElasticsearchArgs].
*/
@PulumiTagMarker
public class SecretBackendConnectionElasticsearchArgsBuilder internal constructor() {
private var caCert: Output? = null
private var caPath: Output? = null
private var clientCert: Output? = null
private var clientKey: Output? = null
private var insecure: Output? = null
private var password: Output? = null
private var tlsServerName: Output? = null
private var url: Output? = null
private var username: Output? = null
private var usernameTemplate: Output? = null
/**
* @param value The path to a PEM-encoded CA cert file to use to verify the Elasticsearch server's identity
*/
@JvmName("tknxcjlvmgmrfxrn")
public suspend fun caCert(`value`: Output) {
this.caCert = value
}
/**
* @param value The path to a directory of PEM-encoded CA cert files to use to verify the Elasticsearch server's identity
*/
@JvmName("vlcndkcshdxbavxr")
public suspend fun caPath(`value`: Output) {
this.caPath = value
}
/**
* @param value The path to the certificate for the Elasticsearch client to present for communication
*/
@JvmName("isuuswohkohruwsg")
public suspend fun clientCert(`value`: Output) {
this.clientCert = value
}
/**
* @param value The path to the key for the Elasticsearch client to use for communication
*/
@JvmName("gnhkkjeqkryrhbqk")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value Whether to disable certificate verification
*/
@JvmName("qkgaxyvdxxurbryx")
public suspend fun insecure(`value`: Output) {
this.insecure = value
}
/**
* @param value The password to be used in the connection URL
*/
@JvmName("hafisdetvfwbtbde")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value This, if set, is used to set the SNI host when connecting via TLS
*/
@JvmName("wmfsslhhwqvshbja")
public suspend fun tlsServerName(`value`: Output) {
this.tlsServerName = value
}
/**
* @param value The URL for Elasticsearch's API
*/
@JvmName("nqhkhhrnuaotpsbk")
public suspend fun url(`value`: Output) {
this.url = value
}
/**
* @param value The username to be used in the connection URL
*/
@JvmName("vsfewjbmufjbdynu")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Template describing how dynamic usernames are generated.
*/
@JvmName("lwwilqppmrodqqxw")
public suspend fun usernameTemplate(`value`: Output) {
this.usernameTemplate = value
}
/**
* @param value The path to a PEM-encoded CA cert file to use to verify the Elasticsearch server's identity
*/
@JvmName("ifrtgeywkwfkmfur")
public suspend fun caCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCert = mapped
}
/**
* @param value The path to a directory of PEM-encoded CA cert files to use to verify the Elasticsearch server's identity
*/
@JvmName("cwtuwccvwyhwpepj")
public suspend fun caPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caPath = mapped
}
/**
* @param value The path to the certificate for the Elasticsearch client to present for communication
*/
@JvmName("gnvoqceqxiptioxy")
public suspend fun clientCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCert = mapped
}
/**
* @param value The path to the key for the Elasticsearch client to use for communication
*/
@JvmName("ufxvtfvwuwuddrxo")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value Whether to disable certificate verification
*/
@JvmName("ovtlvqbirjxjwjuq")
public suspend fun insecure(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.insecure = mapped
}
/**
* @param value The password to be used in the connection URL
*/
@JvmName("slyamleboeiorurl")
public suspend fun password(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value This, if set, is used to set the SNI host when connecting via TLS
*/
@JvmName("okqkmtkpykjawjxf")
public suspend fun tlsServerName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsServerName = mapped
}
/**
* @param value The URL for Elasticsearch's API
*/
@JvmName("vrqrhpvbfwlxpujk")
public suspend fun url(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.url = mapped
}
/**
* @param value The username to be used in the connection URL
*/
@JvmName("ghgjuisnfqnfpyjv")
public suspend fun username(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.username = mapped
}
/**
* @param value Template describing how dynamic usernames are generated.
*/
@JvmName("ebwifxbkcvnklnxt")
public suspend fun usernameTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameTemplate = mapped
}
internal fun build(): SecretBackendConnectionElasticsearchArgs =
SecretBackendConnectionElasticsearchArgs(
caCert = caCert,
caPath = caPath,
clientCert = clientCert,
clientKey = clientKey,
insecure = insecure,
password = password ?: throw PulumiNullFieldException("password"),
tlsServerName = tlsServerName,
url = url ?: throw PulumiNullFieldException("url"),
username = username ?: throw PulumiNullFieldException("username"),
usernameTemplate = usernameTemplate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy