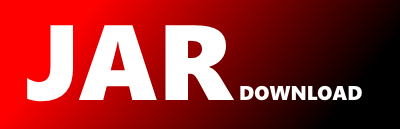
com.pulumi.vault.database.kotlin.inputs.SecretBackendConnectionOracleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.database.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.database.inputs.SecretBackendConnectionOracleArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property connectionUrl Connection string to use to connect to the database.
* @property disconnectSessions Set to true to disconnect any open sessions prior to running the revocation statements.
* @property maxConnectionLifetime Maximum number of seconds a connection may be reused.
* @property maxIdleConnections Maximum number of idle connections to the database.
* @property maxOpenConnections Maximum number of open connections to the database.
* @property password The root credential password used in the connection URL
* @property splitStatements Set to true in order to split statements after semi-colons.
* @property username The root credential username used in the connection URL
* @property usernameTemplate Username generation template.
*/
public data class SecretBackendConnectionOracleArgs(
public val connectionUrl: Output? = null,
public val disconnectSessions: Output? = null,
public val maxConnectionLifetime: Output? = null,
public val maxIdleConnections: Output? = null,
public val maxOpenConnections: Output? = null,
public val password: Output? = null,
public val splitStatements: Output? = null,
public val username: Output? = null,
public val usernameTemplate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.database.inputs.SecretBackendConnectionOracleArgs =
com.pulumi.vault.database.inputs.SecretBackendConnectionOracleArgs.builder()
.connectionUrl(connectionUrl?.applyValue({ args0 -> args0 }))
.disconnectSessions(disconnectSessions?.applyValue({ args0 -> args0 }))
.maxConnectionLifetime(maxConnectionLifetime?.applyValue({ args0 -> args0 }))
.maxIdleConnections(maxIdleConnections?.applyValue({ args0 -> args0 }))
.maxOpenConnections(maxOpenConnections?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.splitStatements(splitStatements?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 }))
.usernameTemplate(usernameTemplate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendConnectionOracleArgs].
*/
@PulumiTagMarker
public class SecretBackendConnectionOracleArgsBuilder internal constructor() {
private var connectionUrl: Output? = null
private var disconnectSessions: Output? = null
private var maxConnectionLifetime: Output? = null
private var maxIdleConnections: Output? = null
private var maxOpenConnections: Output? = null
private var password: Output? = null
private var splitStatements: Output? = null
private var username: Output? = null
private var usernameTemplate: Output? = null
/**
* @param value Connection string to use to connect to the database.
*/
@JvmName("desfjdqplybyalup")
public suspend fun connectionUrl(`value`: Output) {
this.connectionUrl = value
}
/**
* @param value Set to true to disconnect any open sessions prior to running the revocation statements.
*/
@JvmName("eqxsqalwpeokqceo")
public suspend fun disconnectSessions(`value`: Output) {
this.disconnectSessions = value
}
/**
* @param value Maximum number of seconds a connection may be reused.
*/
@JvmName("fvwthtylmfisqvcq")
public suspend fun maxConnectionLifetime(`value`: Output) {
this.maxConnectionLifetime = value
}
/**
* @param value Maximum number of idle connections to the database.
*/
@JvmName("cwiqrersdjecsdsq")
public suspend fun maxIdleConnections(`value`: Output) {
this.maxIdleConnections = value
}
/**
* @param value Maximum number of open connections to the database.
*/
@JvmName("doykynqvhpgpjfbp")
public suspend fun maxOpenConnections(`value`: Output) {
this.maxOpenConnections = value
}
/**
* @param value The root credential password used in the connection URL
*/
@JvmName("ntqinjcxsqgukhcp")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value Set to true in order to split statements after semi-colons.
*/
@JvmName("ctcbnpsdkwpeblst")
public suspend fun splitStatements(`value`: Output) {
this.splitStatements = value
}
/**
* @param value The root credential username used in the connection URL
*/
@JvmName("ivmwtikpvfbjfuvu")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Username generation template.
*/
@JvmName("hyktthwakrflmvdd")
public suspend fun usernameTemplate(`value`: Output) {
this.usernameTemplate = value
}
/**
* @param value Connection string to use to connect to the database.
*/
@JvmName("fnjjoidyhrhkncvw")
public suspend fun connectionUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionUrl = mapped
}
/**
* @param value Set to true to disconnect any open sessions prior to running the revocation statements.
*/
@JvmName("phxchjakbmbmgpaj")
public suspend fun disconnectSessions(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disconnectSessions = mapped
}
/**
* @param value Maximum number of seconds a connection may be reused.
*/
@JvmName("ynteplgelludwydx")
public suspend fun maxConnectionLifetime(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConnectionLifetime = mapped
}
/**
* @param value Maximum number of idle connections to the database.
*/
@JvmName("yhpcfdkglsbedryl")
public suspend fun maxIdleConnections(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxIdleConnections = mapped
}
/**
* @param value Maximum number of open connections to the database.
*/
@JvmName("hneoddbordivbpit")
public suspend fun maxOpenConnections(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxOpenConnections = mapped
}
/**
* @param value The root credential password used in the connection URL
*/
@JvmName("fkhdbpdkbqmtlgio")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value Set to true in order to split statements after semi-colons.
*/
@JvmName("yinvnxsmkhhqummf")
public suspend fun splitStatements(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.splitStatements = mapped
}
/**
* @param value The root credential username used in the connection URL
*/
@JvmName("mvolglejqyivttql")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
/**
* @param value Username generation template.
*/
@JvmName("ojvnoxxlljixqrfi")
public suspend fun usernameTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameTemplate = mapped
}
internal fun build(): SecretBackendConnectionOracleArgs = SecretBackendConnectionOracleArgs(
connectionUrl = connectionUrl,
disconnectSessions = disconnectSessions,
maxConnectionLifetime = maxConnectionLifetime,
maxIdleConnections = maxIdleConnections,
maxOpenConnections = maxOpenConnections,
password = password,
splitStatements = splitStatements,
username = username,
usernameTemplate = usernameTemplate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy