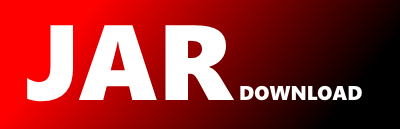
com.pulumi.vault.database.kotlin.inputs.SecretsMountSnowflakeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.database.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.database.inputs.SecretsMountSnowflakeArgs.builder
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property allowedRoles A list of roles that are allowed to use this
* connection.
* @property connectionUrl Connection string to use to connect to the database.
* @property data A map of sensitive data to pass to the endpoint. Useful for templated connection strings.
* Supported list of database secrets engines that can be configured:
* @property maxConnectionLifetime Maximum number of seconds a connection may be reused.
* @property maxIdleConnections Maximum number of idle connections to the database.
* @property maxOpenConnections Maximum number of open connections to the database.
* @property name Name of the database connection.
* @property password The root credential password used in the connection URL
* @property pluginName Specifies the name of the plugin to use.
* @property rootRotationStatements A list of database statements to be executed to rotate the root user's credentials.
* @property username The root credential username used in the connection URL
* @property usernameTemplate Username generation template.
* @property verifyConnection Whether the connection should be verified on
* initial configuration or not.
*/
public data class SecretsMountSnowflakeArgs(
public val allowedRoles: Output>? = null,
public val connectionUrl: Output? = null,
public val `data`: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy