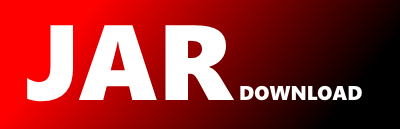
com.pulumi.vault.gcp.kotlin.AuthBackend.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.gcp.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import com.pulumi.vault.gcp.kotlin.outputs.AuthBackendCustomEndpoint
import com.pulumi.vault.gcp.kotlin.outputs.AuthBackendTune
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.vault.gcp.kotlin.outputs.AuthBackendCustomEndpoint.Companion.toKotlin as authBackendCustomEndpointToKotlin
import com.pulumi.vault.gcp.kotlin.outputs.AuthBackendTune.Companion.toKotlin as authBackendTuneToKotlin
/**
* Builder for [AuthBackend].
*/
@PulumiTagMarker
public class AuthBackendResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuthBackendArgs = AuthBackendArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuthBackendArgsBuilder.() -> Unit) {
val builder = AuthBackendArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AuthBackend {
val builtJavaResource = com.pulumi.vault.gcp.AuthBackend(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AuthBackend(builtJavaResource)
}
}
/**
* Provides a resource to configure the [GCP auth backend within Vault](https://www.vaultproject.io/docs/auth/gcp.html).
* ## Example Usage
* You can setup the GCP auth backend with Workload Identity Federation (WIF) for a secret-less configuration:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const gcp = new vault.gcp.AuthBackend("gcp", {
* identityTokenKey: "example-key",
* identityTokenTtl: 1800,
* identityTokenAudience: "",
* serviceAccountEmail: "",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* gcp = vault.gcp.AuthBackend("gcp",
* identity_token_key="example-key",
* identity_token_ttl=1800,
* identity_token_audience="",
* service_account_email="")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var gcp = new Vault.Gcp.AuthBackend("gcp", new()
* {
* IdentityTokenKey = "example-key",
* IdentityTokenTtl = 1800,
* IdentityTokenAudience = "",
* ServiceAccountEmail = "",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/gcp"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gcp.NewAuthBackend(ctx, "gcp", &gcp.AuthBackendArgs{
* IdentityTokenKey: pulumi.String("example-key"),
* IdentityTokenTtl: pulumi.Int(1800),
* IdentityTokenAudience: pulumi.String(""),
* ServiceAccountEmail: pulumi.String(""),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.gcp.AuthBackend;
* import com.pulumi.vault.gcp.AuthBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var gcp = new AuthBackend("gcp", AuthBackendArgs.builder()
* .identityTokenKey("example-key")
* .identityTokenTtl(1800)
* .identityTokenAudience("")
* .serviceAccountEmail("")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* gcp:
* type: vault:gcp:AuthBackend
* properties:
* identityTokenKey: example-key
* identityTokenTtl: 1800
* identityTokenAudience:
* serviceAccountEmail:
* ```
*
* ## Import
* GCP authentication backends can be imported using the backend name, e.g.
* ```sh
* $ pulumi import vault:gcp/authBackend:AuthBackend gcp gcp
* ```
*/
public class AuthBackend internal constructor(
override val javaResource: com.pulumi.vault.gcp.AuthBackend,
) : KotlinCustomResource(javaResource, AuthBackendMapper) {
/**
* The mount accessor related to the auth mount. It is useful for integration with [Identity Secrets Engine](https://www.vaultproject.io/docs/secrets/identity/index.html).
*/
public val accessor: Output
get() = javaResource.accessor().applyValue({ args0 -> args0 })
/**
* The clients email associated with the credentials
*/
public val clientEmail: Output
get() = javaResource.clientEmail().applyValue({ args0 -> args0 })
/**
* The Client ID of the credentials
*/
public val clientId: Output
get() = javaResource.clientId().applyValue({ args0 -> args0 })
/**
* A JSON string containing the contents of a GCP credentials file. If this value is empty, Vault will try to use Application Default Credentials from the machine on which the Vault server is running.
*/
public val credentials: Output?
get() = javaResource.credentials().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies overrides to
* [service endpoints](https://cloud.google.com/apis/design/glossary#api_service_endpoint)
* used when making API requests. This allows specific requests made during authentication
* to target alternative service endpoints for use in [Private Google Access](https://cloud.google.com/vpc/docs/configure-private-google-access)
* environments. Requires Vault 1.11+.
* Overrides are set at the subdomain level using the following keys:
*/
public val customEndpoint: Output?
get() = javaResource.customEndpoint().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> authBackendCustomEndpointToKotlin(args0) })
}).orElse(null)
})
/**
* A description of the auth method.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
public val disableRemount: Output?
get() = javaResource.disableRemount().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The audience claim value for plugin identity
* tokens. Must match an allowed audience configured for the target [Workload Identity Pool](https://cloud.google.com/iam/docs/workload-identity-federation-with-other-providers#prepare).
* Mutually exclusive with `credentials`. Requires Vault 1.17+. *Available only for Vault Enterprise*.
*/
public val identityTokenAudience: Output?
get() = javaResource.identityTokenAudience().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The key to use for signing plugin identity
* tokens. Requires Vault 1.17+. *Available only for Vault Enterprise*.
*/
public val identityTokenKey: Output?
get() = javaResource.identityTokenKey().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The TTL of generated tokens.
*/
public val identityTokenTtl: Output?
get() = javaResource.identityTokenTtl().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies if the auth method is local only.
*/
public val local: Output?
get() = javaResource.local().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The path to mount the auth method — this defaults to 'gcp'.
*/
public val path: Output?
get() = javaResource.path().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The ID of the private key from the credentials
*/
public val privateKeyId: Output
get() = javaResource.privateKeyId().applyValue({ args0 -> args0 })
/**
* The GCP Project ID
*/
public val projectId: Output
get() = javaResource.projectId().applyValue({ args0 -> args0 })
/**
* Service Account to impersonate for plugin workload identity federation.
* Required with `identity_token_audience`. Requires Vault 1.17+. *Available only for Vault Enterprise*.
*/
public val serviceAccountEmail: Output?
get() = javaResource.serviceAccountEmail().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Extra configuration block. Structure is documented below.
* The `tune` block is used to tune the auth backend:
*/
public val tune: Output
get() = javaResource.tune().applyValue({ args0 ->
args0.let({ args0 ->
authBackendTuneToKotlin(args0)
})
})
}
public object AuthBackendMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.gcp.AuthBackend::class == javaResource::class
override fun map(javaResource: Resource): AuthBackend = AuthBackend(
javaResource as
com.pulumi.vault.gcp.AuthBackend,
)
}
/**
* @see [AuthBackend].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AuthBackend].
*/
public suspend fun authBackend(name: String, block: suspend AuthBackendResourceBuilder.() -> Unit): AuthBackend {
val builder = AuthBackendResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AuthBackend].
* @param name The _unique_ name of the resulting resource.
*/
public fun authBackend(name: String): AuthBackend {
val builder = AuthBackendResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy