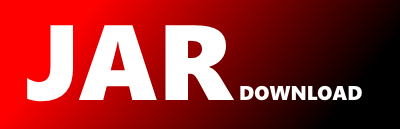
com.pulumi.vault.gcp.kotlin.AuthBackendRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.gcp.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.gcp.AuthBackendRoleArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a resource to create a role in an [GCP auth backend within Vault](https://www.vaultproject.io/docs/auth/gcp.html).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const gcp = new vault.AuthBackend("gcp", {
* path: "gcp",
* type: "gcp",
* });
* const test = new vault.gcp.AuthBackendRole("test", {
* backend: gcp.path,
* role: "test",
* type: "iam",
* boundServiceAccounts: ["test"],
* boundProjects: ["test"],
* tokenTtl: 300,
* tokenMaxTtl: 600,
* tokenPolicies: [
* "policy_a",
* "policy_b",
* ],
* addGroupAliases: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* gcp = vault.AuthBackend("gcp",
* path="gcp",
* type="gcp")
* test = vault.gcp.AuthBackendRole("test",
* backend=gcp.path,
* role="test",
* type="iam",
* bound_service_accounts=["test"],
* bound_projects=["test"],
* token_ttl=300,
* token_max_ttl=600,
* token_policies=[
* "policy_a",
* "policy_b",
* ],
* add_group_aliases=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var gcp = new Vault.AuthBackend("gcp", new()
* {
* Path = "gcp",
* Type = "gcp",
* });
* var test = new Vault.Gcp.AuthBackendRole("test", new()
* {
* Backend = gcp.Path,
* Role = "test",
* Type = "iam",
* BoundServiceAccounts = new[]
* {
* "test",
* },
* BoundProjects = new[]
* {
* "test",
* },
* TokenTtl = 300,
* TokenMaxTtl = 600,
* TokenPolicies = new[]
* {
* "policy_a",
* "policy_b",
* },
* AddGroupAliases = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/gcp"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* gcp, err := vault.NewAuthBackend(ctx, "gcp", &vault.AuthBackendArgs{
* Path: pulumi.String("gcp"),
* Type: pulumi.String("gcp"),
* })
* if err != nil {
* return err
* }
* _, err = gcp.NewAuthBackendRole(ctx, "test", &gcp.AuthBackendRoleArgs{
* Backend: gcp.Path,
* Role: pulumi.String("test"),
* Type: pulumi.String("iam"),
* BoundServiceAccounts: pulumi.StringArray{
* pulumi.String("test"),
* },
* BoundProjects: pulumi.StringArray{
* pulumi.String("test"),
* },
* TokenTtl: pulumi.Int(300),
* TokenMaxTtl: pulumi.Int(600),
* TokenPolicies: pulumi.StringArray{
* pulumi.String("policy_a"),
* pulumi.String("policy_b"),
* },
* AddGroupAliases: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.gcp.AuthBackendRole;
* import com.pulumi.vault.gcp.AuthBackendRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var gcp = new AuthBackend("gcp", AuthBackendArgs.builder()
* .path("gcp")
* .type("gcp")
* .build());
* var test = new AuthBackendRole("test", AuthBackendRoleArgs.builder()
* .backend(gcp.path())
* .role("test")
* .type("iam")
* .boundServiceAccounts("test")
* .boundProjects("test")
* .tokenTtl(300)
* .tokenMaxTtl(600)
* .tokenPolicies(
* "policy_a",
* "policy_b")
* .addGroupAliases(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* gcp:
* type: vault:AuthBackend
* properties:
* path: gcp
* type: gcp
* test:
* type: vault:gcp:AuthBackendRole
* properties:
* backend: ${gcp.path}
* role: test
* type: iam
* boundServiceAccounts:
* - test
* boundProjects:
* - test
* tokenTtl: 300
* tokenMaxTtl: 600
* tokenPolicies:
* - policy_a
* - policy_b
* addGroupAliases: true
* ```
*
* ## Import
* GCP authentication roles can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:gcp/authBackendRole:AuthBackendRole my_role auth/gcp/role/my_role
* ```
* @property addGroupAliases
* @property allowGceInference
* @property backend Path to the mounted GCP auth backend
* @property boundInstanceGroups
* @property boundLabels
* @property boundProjects An array of GCP project IDs. Only entities belonging to this project can authenticate under the role.
* @property boundRegions
* @property boundServiceAccounts GCP Service Accounts allowed to issue tokens under this role. (Note: **Required** if role is `iam`)
* @property boundZones
* @property maxJwtExp
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property role Name of the GCP role
* @property tokenBoundCidrs Specifies the blocks of IP addresses which are allowed to use the generated token
* @property tokenExplicitMaxTtl Generated Token's Explicit Maximum TTL in seconds
* @property tokenMaxTtl The maximum lifetime of the generated token
* @property tokenNoDefaultPolicy If true, the 'default' policy will not automatically be added to generated tokens
* @property tokenNumUses The maximum number of times a token may be used, a value of zero means unlimited
* @property tokenPeriod Generated Token's Period
* @property tokenPolicies Generated Token's Policies
* @property tokenTtl The initial ttl of the token to generate in seconds
* @property tokenType The type of token to generate, service or batch
* @property type Type of GCP authentication role (either `gce` or `iam`)
*/
public data class AuthBackendRoleArgs(
public val addGroupAliases: Output? = null,
public val allowGceInference: Output? = null,
public val backend: Output? = null,
public val boundInstanceGroups: Output>? = null,
public val boundLabels: Output>? = null,
public val boundProjects: Output>? = null,
public val boundRegions: Output>? = null,
public val boundServiceAccounts: Output>? = null,
public val boundZones: Output>? = null,
public val maxJwtExp: Output? = null,
public val namespace: Output? = null,
public val role: Output? = null,
public val tokenBoundCidrs: Output>? = null,
public val tokenExplicitMaxTtl: Output? = null,
public val tokenMaxTtl: Output? = null,
public val tokenNoDefaultPolicy: Output? = null,
public val tokenNumUses: Output? = null,
public val tokenPeriod: Output? = null,
public val tokenPolicies: Output>? = null,
public val tokenTtl: Output? = null,
public val tokenType: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.gcp.AuthBackendRoleArgs =
com.pulumi.vault.gcp.AuthBackendRoleArgs.builder()
.addGroupAliases(addGroupAliases?.applyValue({ args0 -> args0 }))
.allowGceInference(allowGceInference?.applyValue({ args0 -> args0 }))
.backend(backend?.applyValue({ args0 -> args0 }))
.boundInstanceGroups(boundInstanceGroups?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.boundLabels(boundLabels?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.boundProjects(boundProjects?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.boundRegions(boundRegions?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.boundServiceAccounts(boundServiceAccounts?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.boundZones(boundZones?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.maxJwtExp(maxJwtExp?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.role(role?.applyValue({ args0 -> args0 }))
.tokenBoundCidrs(tokenBoundCidrs?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenExplicitMaxTtl(tokenExplicitMaxTtl?.applyValue({ args0 -> args0 }))
.tokenMaxTtl(tokenMaxTtl?.applyValue({ args0 -> args0 }))
.tokenNoDefaultPolicy(tokenNoDefaultPolicy?.applyValue({ args0 -> args0 }))
.tokenNumUses(tokenNumUses?.applyValue({ args0 -> args0 }))
.tokenPeriod(tokenPeriod?.applyValue({ args0 -> args0 }))
.tokenPolicies(tokenPolicies?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenTtl(tokenTtl?.applyValue({ args0 -> args0 }))
.tokenType(tokenType?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AuthBackendRoleArgs].
*/
@PulumiTagMarker
public class AuthBackendRoleArgsBuilder internal constructor() {
private var addGroupAliases: Output? = null
private var allowGceInference: Output? = null
private var backend: Output? = null
private var boundInstanceGroups: Output>? = null
private var boundLabels: Output>? = null
private var boundProjects: Output>? = null
private var boundRegions: Output>? = null
private var boundServiceAccounts: Output>? = null
private var boundZones: Output>? = null
private var maxJwtExp: Output? = null
private var namespace: Output? = null
private var role: Output? = null
private var tokenBoundCidrs: Output>? = null
private var tokenExplicitMaxTtl: Output? = null
private var tokenMaxTtl: Output? = null
private var tokenNoDefaultPolicy: Output? = null
private var tokenNumUses: Output? = null
private var tokenPeriod: Output? = null
private var tokenPolicies: Output>? = null
private var tokenTtl: Output? = null
private var tokenType: Output? = null
private var type: Output? = null
/**
* @param value
*/
@JvmName("rbttowlxrjrmibit")
public suspend fun addGroupAliases(`value`: Output) {
this.addGroupAliases = value
}
/**
* @param value
*/
@JvmName("otteybcxqdspxhtn")
public suspend fun allowGceInference(`value`: Output) {
this.allowGceInference = value
}
/**
* @param value Path to the mounted GCP auth backend
*/
@JvmName("cdphxtmqqgmoqnfo")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value
*/
@JvmName("iqophntnkuhmvtrj")
public suspend fun boundInstanceGroups(`value`: Output>) {
this.boundInstanceGroups = value
}
@JvmName("ymdoieicobwqolce")
public suspend fun boundInstanceGroups(vararg values: Output) {
this.boundInstanceGroups = Output.all(values.asList())
}
/**
* @param values
*/
@JvmName("pcoquwvqurgtbvpj")
public suspend fun boundInstanceGroups(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy