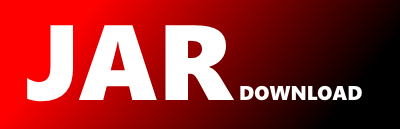
com.pulumi.vault.gcp.kotlin.GcpFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.gcp.kotlin
import com.pulumi.vault.gcp.GcpFunctions.getAuthBackendRolePlain
import com.pulumi.vault.gcp.kotlin.inputs.GetAuthBackendRolePlainArgs
import com.pulumi.vault.gcp.kotlin.inputs.GetAuthBackendRolePlainArgsBuilder
import com.pulumi.vault.gcp.kotlin.outputs.GetAuthBackendRoleResult
import com.pulumi.vault.gcp.kotlin.outputs.GetAuthBackendRoleResult.Companion.toKotlin
import kotlinx.coroutines.future.await
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
public object GcpFunctions {
/**
* Reads a GCP auth role from a Vault server.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* export = async () => {
* const role = await vault.gcp.getAuthBackendRole({
* backend: "my-gcp-backend",
* roleName: "my-role",
* });
* return {
* "role-id": role.roleId,
* };
* }
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* role = vault.gcp.get_auth_backend_role(backend="my-gcp-backend",
* role_name="my-role")
* pulumi.export("role-id", role.role_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var role = Vault.Gcp.GetAuthBackendRole.Invoke(new()
* {
* Backend = "my-gcp-backend",
* RoleName = "my-role",
* });
* return new Dictionary
* {
* ["role-id"] = role.Apply(getAuthBackendRoleResult => getAuthBackendRoleResult.RoleId),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/gcp"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* role, err := gcp.LookupAuthBackendRole(ctx, &gcp.LookupAuthBackendRoleArgs{
* Backend: pulumi.StringRef("my-gcp-backend"),
* RoleName: "my-role",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("role-id", role.RoleId)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.gcp.GcpFunctions;
* import com.pulumi.vault.gcp.inputs.GetAuthBackendRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var role = GcpFunctions.getAuthBackendRole(GetAuthBackendRoleArgs.builder()
* .backend("my-gcp-backend")
* .roleName("my-role")
* .build());
* ctx.export("role-id", role.applyValue(getAuthBackendRoleResult -> getAuthBackendRoleResult.roleId()));
* }
* }
* ```
* ```yaml
* variables:
* role:
* fn::invoke:
* Function: vault:gcp:getAuthBackendRole
* Arguments:
* backend: my-gcp-backend
* roleName: my-role
* outputs:
* role-id: ${role.roleId}
* ```
*
* @param argument A collection of arguments for invoking getAuthBackendRole.
* @return A collection of values returned by getAuthBackendRole.
*/
public suspend fun getAuthBackendRole(argument: GetAuthBackendRolePlainArgs): GetAuthBackendRoleResult = toKotlin(getAuthBackendRolePlain(argument.toJava()).await())
/**
* @see [getAuthBackendRole].
* @param backend The unique name for the GCP backend from which to fetch the role. Defaults to "gcp".
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured namespace.
* *Available only for Vault Enterprise*.
* @param roleName The name of the role to retrieve the Role ID for.
* @param tokenBoundCidrs List of CIDR blocks; if set, specifies blocks of IP
* addresses which can authenticate successfully, and ties the resulting token to these blocks
* as well.
* @param tokenExplicitMaxTtl If set, will encode an
* [explicit max TTL](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls)
* onto the token in number of seconds. This is a hard cap even if `token_ttl` and
* `token_max_ttl` would otherwise allow a renewal.
* @param tokenMaxTtl The maximum lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @param tokenNoDefaultPolicy If set, the default policy will not be set on
* generated tokens; otherwise it will be added to the policies set in token_policies.
* @param tokenNumUses The
* [period](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls),
* if any, in number of seconds to set on the token.
* @param tokenPeriod (Optional) If set, indicates that the
* token generated using this role should never expire. The token should be renewed within the
* duration specified by this value. At each renewal, the token's TTL will be set to the
* value of this field. Specified in seconds.
* @param tokenPolicies List of policies to encode onto generated tokens. Depending
* on the auth method, this list may be supplemented by user/group/other values.
* @param tokenTtl The incremental lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @param tokenType The type of token that should be generated. Can be `service`,
* `batch`, or `default` to use the mount's tuned default (which unless changed will be
* `service` tokens). For token store roles, there are two additional possibilities:
* `default-service` and `default-batch` which specify the type to return unless the client
* requests a different type at generation time.
* @return A collection of values returned by getAuthBackendRole.
*/
public suspend fun getAuthBackendRole(
backend: String? = null,
namespace: String? = null,
roleName: String,
tokenBoundCidrs: List? = null,
tokenExplicitMaxTtl: Int? = null,
tokenMaxTtl: Int? = null,
tokenNoDefaultPolicy: Boolean? = null,
tokenNumUses: Int? = null,
tokenPeriod: Int? = null,
tokenPolicies: List? = null,
tokenTtl: Int? = null,
tokenType: String? = null,
): GetAuthBackendRoleResult {
val argument = GetAuthBackendRolePlainArgs(
backend = backend,
namespace = namespace,
roleName = roleName,
tokenBoundCidrs = tokenBoundCidrs,
tokenExplicitMaxTtl = tokenExplicitMaxTtl,
tokenMaxTtl = tokenMaxTtl,
tokenNoDefaultPolicy = tokenNoDefaultPolicy,
tokenNumUses = tokenNumUses,
tokenPeriod = tokenPeriod,
tokenPolicies = tokenPolicies,
tokenTtl = tokenTtl,
tokenType = tokenType,
)
return toKotlin(getAuthBackendRolePlain(argument.toJava()).await())
}
/**
* @see [getAuthBackendRole].
* @param argument Builder for [com.pulumi.vault.gcp.kotlin.inputs.GetAuthBackendRolePlainArgs].
* @return A collection of values returned by getAuthBackendRole.
*/
public suspend fun getAuthBackendRole(argument: suspend GetAuthBackendRolePlainArgsBuilder.() -> Unit): GetAuthBackendRoleResult {
val builder = GetAuthBackendRolePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return toKotlin(getAuthBackendRolePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy