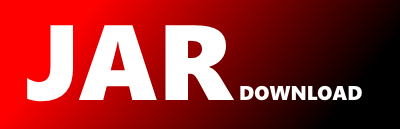
com.pulumi.vault.gcp.kotlin.SecretStaticAccountArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.gcp.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.gcp.SecretStaticAccountArgs.builder
import com.pulumi.vault.gcp.kotlin.inputs.SecretStaticAccountBindingArgs
import com.pulumi.vault.gcp.kotlin.inputs.SecretStaticAccountBindingArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Creates a Static Account in the [GCP Secrets Engine](https://www.vaultproject.io/docs/secrets/gcp/index.html) for Vault.
* Each [static account](https://www.vaultproject.io/docs/secrets/gcp/index.html#static-accounts) is tied to a separately managed
* Service Account, and can have one or more [bindings](https://www.vaultproject.io/docs/secrets/gcp/index.html#bindings) associated with it.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as google from "@pulumi/google";
* import * as std from "@pulumi/std";
* import * as vault from "@pulumi/vault";
* const _this = new google.index.ServiceAccount("this", {accountId: "my-awesome-account"});
* const gcp = new vault.gcp.SecretBackend("gcp", {
* path: "gcp",
* credentials: std.file({
* input: "credentials.json",
* }).then(invoke => invoke.result),
* });
* const staticAccount = new vault.gcp.SecretStaticAccount("static_account", {
* backend: gcp.path,
* staticAccount: "project_viewer",
* secretType: "access_token",
* tokenScopes: ["https://www.googleapis.com/auth/cloud-platform"],
* serviceAccountEmail: _this.email,
* bindings: [{
* resource: `//cloudresourcemanager.googleapis.com/projects/${_this.project}`,
* roles: ["roles/viewer"],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_google as google
* import pulumi_std as std
* import pulumi_vault as vault
* this = google.index.ServiceAccount("this", account_id=my-awesome-account)
* gcp = vault.gcp.SecretBackend("gcp",
* path="gcp",
* credentials=std.file(input="credentials.json").result)
* static_account = vault.gcp.SecretStaticAccount("static_account",
* backend=gcp.path,
* static_account="project_viewer",
* secret_type="access_token",
* token_scopes=["https://www.googleapis.com/auth/cloud-platform"],
* service_account_email=this["email"],
* bindings=[vault.gcp.SecretStaticAccountBindingArgs(
* resource=f"//cloudresourcemanager.googleapis.com/projects/{this['project']}",
* roles=["roles/viewer"],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Google = Pulumi.Google;
* using Std = Pulumi.Std;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var @this = new Google.Index.ServiceAccount("this", new()
* {
* AccountId = "my-awesome-account",
* });
* var gcp = new Vault.Gcp.SecretBackend("gcp", new()
* {
* Path = "gcp",
* Credentials = Std.File.Invoke(new()
* {
* Input = "credentials.json",
* }).Apply(invoke => invoke.Result),
* });
* var staticAccount = new Vault.Gcp.SecretStaticAccount("static_account", new()
* {
* Backend = gcp.Path,
* StaticAccount = "project_viewer",
* SecretType = "access_token",
* TokenScopes = new[]
* {
* "https://www.googleapis.com/auth/cloud-platform",
* },
* ServiceAccountEmail = @this.Email,
* Bindings = new[]
* {
* new Vault.Gcp.Inputs.SecretStaticAccountBindingArgs
* {
* Resource = $"//cloudresourcemanager.googleapis.com/projects/{@this.Project}",
* Roles = new[]
* {
* "roles/viewer",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-google/sdk/go/google"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/gcp"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* this, err := google.NewServiceAccount(ctx, "this", &google.ServiceAccountArgs{
* AccountId: "my-awesome-account",
* })
* if err != nil {
* return err
* }
* invokeFile, err := std.File(ctx, &std.FileArgs{
* Input: "credentials.json",
* }, nil)
* if err != nil {
* return err
* }
* gcp, err := gcp.NewSecretBackend(ctx, "gcp", &gcp.SecretBackendArgs{
* Path: pulumi.String("gcp"),
* Credentials: pulumi.String(invokeFile.Result),
* })
* if err != nil {
* return err
* }
* _, err = gcp.NewSecretStaticAccount(ctx, "static_account", &gcp.SecretStaticAccountArgs{
* Backend: gcp.Path,
* StaticAccount: pulumi.String("project_viewer"),
* SecretType: pulumi.String("access_token"),
* TokenScopes: pulumi.StringArray{
* pulumi.String("https://www.googleapis.com/auth/cloud-platform"),
* },
* ServiceAccountEmail: this.Email,
* Bindings: gcp.SecretStaticAccountBindingArray{
* &gcp.SecretStaticAccountBindingArgs{
* Resource: pulumi.String(fmt.Sprintf("//cloudresourcemanager.googleapis.com/projects/%v", this.Project)),
* Roles: pulumi.StringArray{
* pulumi.String("roles/viewer"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.google.serviceAccount;
* import com.pulumi.google.ServiceAccountArgs;
* import com.pulumi.vault.gcp.SecretBackend;
* import com.pulumi.vault.gcp.SecretBackendArgs;
* import com.pulumi.vault.gcp.SecretStaticAccount;
* import com.pulumi.vault.gcp.SecretStaticAccountArgs;
* import com.pulumi.vault.gcp.inputs.SecretStaticAccountBindingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var this_ = new ServiceAccount("this", ServiceAccountArgs.builder()
* .accountId("my-awesome-account")
* .build());
* var gcp = new SecretBackend("gcp", SecretBackendArgs.builder()
* .path("gcp")
* .credentials(StdFunctions.file(FileArgs.builder()
* .input("credentials.json")
* .build()).result())
* .build());
* var staticAccount = new SecretStaticAccount("staticAccount", SecretStaticAccountArgs.builder()
* .backend(gcp.path())
* .staticAccount("project_viewer")
* .secretType("access_token")
* .tokenScopes("https://www.googleapis.com/auth/cloud-platform")
* .serviceAccountEmail(this_.email())
* .bindings(SecretStaticAccountBindingArgs.builder()
* .resource(String.format("//cloudresourcemanager.googleapis.com/projects/%s", this_.project()))
* .roles("roles/viewer")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* this:
* type: google:serviceAccount
* properties:
* accountId: my-awesome-account
* gcp:
* type: vault:gcp:SecretBackend
* properties:
* path: gcp
* credentials:
* fn::invoke:
* Function: std:file
* Arguments:
* input: credentials.json
* Return: result
* staticAccount:
* type: vault:gcp:SecretStaticAccount
* name: static_account
* properties:
* backend: ${gcp.path}
* staticAccount: project_viewer
* secretType: access_token
* tokenScopes:
* - https://www.googleapis.com/auth/cloud-platform
* serviceAccountEmail: ${this.email}
* bindings:
* - resource: //cloudresourcemanager.googleapis.com/projects/${this.project}
* roles:
* - roles/viewer
* ```
*
* ## Import
* A static account can be imported using its Vault Path. For example, referencing the example above,
* ```sh
* $ pulumi import vault:gcp/secretStaticAccount:SecretStaticAccount static_account gcp/static-account/project_viewer
* ```
* @property backend Path where the GCP Secrets Engine is mounted
* @property bindings Bindings to create for this static account. This can be specified multiple times for multiple bindings. Structure is documented below.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property secretType Type of secret generated for this static account. Accepted values: `access_token`, `service_account_key`. Defaults to `access_token`.
* @property serviceAccountEmail Email of the GCP service account to manage.
* @property staticAccount Name of the Static Account to create
* @property tokenScopes List of OAuth scopes to assign to `access_token` secrets generated under this static account (`access_token` static accounts only).
*/
public data class SecretStaticAccountArgs(
public val backend: Output? = null,
public val bindings: Output>? = null,
public val namespace: Output? = null,
public val secretType: Output? = null,
public val serviceAccountEmail: Output? = null,
public val staticAccount: Output? = null,
public val tokenScopes: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.gcp.SecretStaticAccountArgs =
com.pulumi.vault.gcp.SecretStaticAccountArgs.builder()
.backend(backend?.applyValue({ args0 -> args0 }))
.bindings(
bindings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.namespace(namespace?.applyValue({ args0 -> args0 }))
.secretType(secretType?.applyValue({ args0 -> args0 }))
.serviceAccountEmail(serviceAccountEmail?.applyValue({ args0 -> args0 }))
.staticAccount(staticAccount?.applyValue({ args0 -> args0 }))
.tokenScopes(tokenScopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [SecretStaticAccountArgs].
*/
@PulumiTagMarker
public class SecretStaticAccountArgsBuilder internal constructor() {
private var backend: Output? = null
private var bindings: Output>? = null
private var namespace: Output? = null
private var secretType: Output? = null
private var serviceAccountEmail: Output? = null
private var staticAccount: Output? = null
private var tokenScopes: Output>? = null
/**
* @param value Path where the GCP Secrets Engine is mounted
*/
@JvmName("lfnnpnbajimmdnpr")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value Bindings to create for this static account. This can be specified multiple times for multiple bindings. Structure is documented below.
*/
@JvmName("qibekeoyepifdfii")
public suspend fun bindings(`value`: Output>) {
this.bindings = value
}
@JvmName("mdrwgqkxryfqijud")
public suspend fun bindings(vararg values: Output) {
this.bindings = Output.all(values.asList())
}
/**
* @param values Bindings to create for this static account. This can be specified multiple times for multiple bindings. Structure is documented below.
*/
@JvmName("upcykqixeefpveba")
public suspend fun bindings(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy