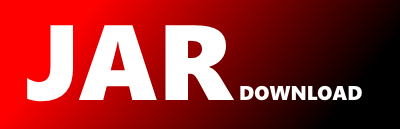
com.pulumi.vault.gcp.kotlin.outputs.GetAuthBackendRoleResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.gcp.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getAuthBackendRole.
* @property backend
* @property boundInstanceGroups GCP regions bound to the role. Returned when `type` is `gce`.
* @property boundLabels GCP labels bound to the role. Returned when `type` is `gce`.
* @property boundProjects GCP projects bound to the role.
* @property boundRegions GCP regions bound to the role. Returned when `type` is `gce`.
* @property boundServiceAccounts GCP service accounts bound to the role. Returned when `type` is `iam`.
* @property boundZones GCP zones bound to the role. Returned when `type` is `gce`.
* @property id The provider-assigned unique ID for this managed resource.
* @property namespace
* @property roleId The RoleID of the GCP role.
* @property roleName
* @property tokenBoundCidrs List of CIDR blocks; if set, specifies blocks of IP
* addresses which can authenticate successfully, and ties the resulting token to these blocks
* as well.
* @property tokenExplicitMaxTtl If set, will encode an
* [explicit max TTL](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls)
* onto the token in number of seconds. This is a hard cap even if `token_ttl` and
* `token_max_ttl` would otherwise allow a renewal.
* @property tokenMaxTtl The maximum lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @property tokenNoDefaultPolicy If set, the default policy will not be set on
* generated tokens; otherwise it will be added to the policies set in token_policies.
* @property tokenNumUses The
* [period](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls),
* if any, in number of seconds to set on the token.
* @property tokenPeriod (Optional) If set, indicates that the
* token generated using this role should never expire. The token should be renewed within the
* duration specified by this value. At each renewal, the token's TTL will be set to the
* value of this field. Specified in seconds.
* @property tokenPolicies List of policies to encode onto generated tokens. Depending
* on the auth method, this list may be supplemented by user/group/other values.
* @property tokenTtl The incremental lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @property tokenType The type of token that should be generated. Can be `service`,
* `batch`, or `default` to use the mount's tuned default (which unless changed will be
* `service` tokens). For token store roles, there are two additional possibilities:
* `default-service` and `default-batch` which specify the type to return unless the client
* requests a different type at generation time.
* @property type Type of GCP role. Expected values are `iam` or `gce`.
*/
public data class GetAuthBackendRoleResult(
public val backend: String? = null,
public val boundInstanceGroups: List,
public val boundLabels: List,
public val boundProjects: List,
public val boundRegions: List,
public val boundServiceAccounts: List,
public val boundZones: List,
public val id: String,
public val namespace: String? = null,
public val roleId: String,
public val roleName: String,
public val tokenBoundCidrs: List? = null,
public val tokenExplicitMaxTtl: Int? = null,
public val tokenMaxTtl: Int? = null,
public val tokenNoDefaultPolicy: Boolean? = null,
public val tokenNumUses: Int? = null,
public val tokenPeriod: Int? = null,
public val tokenPolicies: List? = null,
public val tokenTtl: Int? = null,
public val tokenType: String? = null,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.vault.gcp.outputs.GetAuthBackendRoleResult): GetAuthBackendRoleResult = GetAuthBackendRoleResult(
backend = javaType.backend().map({ args0 -> args0 }).orElse(null),
boundInstanceGroups = javaType.boundInstanceGroups().map({ args0 -> args0 }),
boundLabels = javaType.boundLabels().map({ args0 -> args0 }),
boundProjects = javaType.boundProjects().map({ args0 -> args0 }),
boundRegions = javaType.boundRegions().map({ args0 -> args0 }),
boundServiceAccounts = javaType.boundServiceAccounts().map({ args0 -> args0 }),
boundZones = javaType.boundZones().map({ args0 -> args0 }),
id = javaType.id(),
namespace = javaType.namespace().map({ args0 -> args0 }).orElse(null),
roleId = javaType.roleId(),
roleName = javaType.roleName(),
tokenBoundCidrs = javaType.tokenBoundCidrs().map({ args0 -> args0 }),
tokenExplicitMaxTtl = javaType.tokenExplicitMaxTtl().map({ args0 -> args0 }).orElse(null),
tokenMaxTtl = javaType.tokenMaxTtl().map({ args0 -> args0 }).orElse(null),
tokenNoDefaultPolicy = javaType.tokenNoDefaultPolicy().map({ args0 -> args0 }).orElse(null),
tokenNumUses = javaType.tokenNumUses().map({ args0 -> args0 }).orElse(null),
tokenPeriod = javaType.tokenPeriod().map({ args0 -> args0 }).orElse(null),
tokenPolicies = javaType.tokenPolicies().map({ args0 -> args0 }),
tokenTtl = javaType.tokenTtl().map({ args0 -> args0 }).orElse(null),
tokenType = javaType.tokenType().map({ args0 -> args0 }).orElse(null),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy