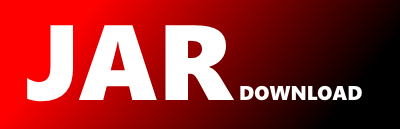
com.pulumi.vault.generic.kotlin.Endpoint.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.generic.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [Endpoint].
*/
@PulumiTagMarker
public class EndpointResourceBuilder internal constructor() {
public var name: String? = null
public var args: EndpointArgs = EndpointArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EndpointArgsBuilder.() -> Unit) {
val builder = EndpointArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Endpoint {
val builtJavaResource = com.pulumi.vault.generic.Endpoint(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Endpoint(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const userpass = new vault.AuthBackend("userpass", {type: "userpass"});
* const u1 = new vault.generic.Endpoint("u1", {
* path: "auth/userpass/users/u1",
* ignoreAbsentFields: true,
* dataJson: `{
* "policies": ["p1"],
* "password": "changeme"
* }
* `,
* }, {
* dependsOn: [userpass],
* });
* const u1Token = new vault.generic.Endpoint("u1_token", {
* path: "auth/userpass/login/u1",
* disableRead: true,
* disableDelete: true,
* dataJson: `{
* "password": "changeme"
* }
* `,
* }, {
* dependsOn: [u1],
* });
* const u1Entity = new vault.generic.Endpoint("u1_entity", {
* disableRead: true,
* disableDelete: true,
* path: "identity/lookup/entity",
* ignoreAbsentFields: true,
* writeFields: ["id"],
* dataJson: `{
* "alias_name": "u1",
* "alias_mount_accessor": vault_auth_backend.userpass.accessor
* }
* `,
* }, {
* dependsOn: [u1Token],
* });
* export const u1Id = u1Entity.writeData.id;
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* userpass = vault.AuthBackend("userpass", type="userpass")
* u1 = vault.generic.Endpoint("u1",
* path="auth/userpass/users/u1",
* ignore_absent_fields=True,
* data_json="""{
* "policies": ["p1"],
* "password": "changeme"
* }
* """,
* opts = pulumi.ResourceOptions(depends_on=[userpass]))
* u1_token = vault.generic.Endpoint("u1_token",
* path="auth/userpass/login/u1",
* disable_read=True,
* disable_delete=True,
* data_json="""{
* "password": "changeme"
* }
* """,
* opts = pulumi.ResourceOptions(depends_on=[u1]))
* u1_entity = vault.generic.Endpoint("u1_entity",
* disable_read=True,
* disable_delete=True,
* path="identity/lookup/entity",
* ignore_absent_fields=True,
* write_fields=["id"],
* data_json="""{
* "alias_name": "u1",
* "alias_mount_accessor": vault_auth_backend.userpass.accessor
* }
* """,
* opts = pulumi.ResourceOptions(depends_on=[u1_token]))
* pulumi.export("u1Id", u1_entity.write_data["id"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var userpass = new Vault.AuthBackend("userpass", new()
* {
* Type = "userpass",
* });
* var u1 = new Vault.Generic.Endpoint("u1", new()
* {
* Path = "auth/userpass/users/u1",
* IgnoreAbsentFields = true,
* DataJson = @"{
* ""policies"": [""p1""],
* ""password"": ""changeme""
* }
* ",
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* userpass,
* },
* });
* var u1Token = new Vault.Generic.Endpoint("u1_token", new()
* {
* Path = "auth/userpass/login/u1",
* DisableRead = true,
* DisableDelete = true,
* DataJson = @"{
* ""password"": ""changeme""
* }
* ",
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* u1,
* },
* });
* var u1Entity = new Vault.Generic.Endpoint("u1_entity", new()
* {
* DisableRead = true,
* DisableDelete = true,
* Path = "identity/lookup/entity",
* IgnoreAbsentFields = true,
* WriteFields = new[]
* {
* "id",
* },
* DataJson = @"{
* ""alias_name"": ""u1"",
* ""alias_mount_accessor"": vault_auth_backend.userpass.accessor
* }
* ",
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* u1Token,
* },
* });
* return new Dictionary
* {
* ["u1Id"] = u1Entity.WriteData.Apply(writeData => writeData.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/generic"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* userpass, err := vault.NewAuthBackend(ctx, "userpass", &vault.AuthBackendArgs{
* Type: pulumi.String("userpass"),
* })
* if err != nil {
* return err
* }
* u1, err := generic.NewEndpoint(ctx, "u1", &generic.EndpointArgs{
* Path: pulumi.String("auth/userpass/users/u1"),
* IgnoreAbsentFields: pulumi.Bool(true),
* DataJson: pulumi.String("{\n \"policies\": [\"p1\"],\n \"password\": \"changeme\"\n}\n"),
* }, pulumi.DependsOn([]pulumi.Resource{
* userpass,
* }))
* if err != nil {
* return err
* }
* u1Token, err := generic.NewEndpoint(ctx, "u1_token", &generic.EndpointArgs{
* Path: pulumi.String("auth/userpass/login/u1"),
* DisableRead: pulumi.Bool(true),
* DisableDelete: pulumi.Bool(true),
* DataJson: pulumi.String("{\n \"password\": \"changeme\"\n}\n"),
* }, pulumi.DependsOn([]pulumi.Resource{
* u1,
* }))
* if err != nil {
* return err
* }
* u1Entity, err := generic.NewEndpoint(ctx, "u1_entity", &generic.EndpointArgs{
* DisableRead: pulumi.Bool(true),
* DisableDelete: pulumi.Bool(true),
* Path: pulumi.String("identity/lookup/entity"),
* IgnoreAbsentFields: pulumi.Bool(true),
* WriteFields: pulumi.StringArray{
* pulumi.String("id"),
* },
* DataJson: pulumi.String("{\n \"alias_name\": \"u1\",\n \"alias_mount_accessor\": vault_auth_backend.userpass.accessor\n}\n"),
* }, pulumi.DependsOn([]pulumi.Resource{
* u1Token,
* }))
* if err != nil {
* return err
* }
* ctx.Export("u1Id", u1Entity.WriteData.ApplyT(func(writeData map[string]string) (string, error) {
* return writeData.Id, nil
* }).(pulumi.StringOutput))
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.generic.Endpoint;
* import com.pulumi.vault.generic.EndpointArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var userpass = new AuthBackend("userpass", AuthBackendArgs.builder()
* .type("userpass")
* .build());
* var u1 = new Endpoint("u1", EndpointArgs.builder()
* .path("auth/userpass/users/u1")
* .ignoreAbsentFields(true)
* .dataJson("""
* {
* "policies": ["p1"],
* "password": "changeme"
* }
* """)
* .build(), CustomResourceOptions.builder()
* .dependsOn(userpass)
* .build());
* var u1Token = new Endpoint("u1Token", EndpointArgs.builder()
* .path("auth/userpass/login/u1")
* .disableRead(true)
* .disableDelete(true)
* .dataJson("""
* {
* "password": "changeme"
* }
* """)
* .build(), CustomResourceOptions.builder()
* .dependsOn(u1)
* .build());
* var u1Entity = new Endpoint("u1Entity", EndpointArgs.builder()
* .disableRead(true)
* .disableDelete(true)
* .path("identity/lookup/entity")
* .ignoreAbsentFields(true)
* .writeFields("id")
* .dataJson("""
* {
* "alias_name": "u1",
* "alias_mount_accessor": vault_auth_backend.userpass.accessor
* }
* """)
* .build(), CustomResourceOptions.builder()
* .dependsOn(u1Token)
* .build());
* ctx.export("u1Id", u1Entity.writeData().applyValue(writeData -> writeData.id()));
* }
* }
* ```
* ```yaml
* resources:
* userpass:
* type: vault:AuthBackend
* properties:
* type: userpass
* u1:
* type: vault:generic:Endpoint
* properties:
* path: auth/userpass/users/u1
* ignoreAbsentFields: true
* dataJson: |
* {
* "policies": ["p1"],
* "password": "changeme"
* }
* options:
* dependson:
* - ${userpass}
* u1Token:
* type: vault:generic:Endpoint
* name: u1_token
* properties:
* path: auth/userpass/login/u1
* disableRead: true
* disableDelete: true
* dataJson: |
* {
* "password": "changeme"
* }
* options:
* dependson:
* - ${u1}
* u1Entity:
* type: vault:generic:Endpoint
* name: u1_entity
* properties:
* disableRead: true
* disableDelete: true
* path: identity/lookup/entity
* ignoreAbsentFields: true
* writeFields:
* - id
* dataJson: |
* {
* "alias_name": "u1",
* "alias_mount_accessor": vault_auth_backend.userpass.accessor
* }
* options:
* dependson:
* - ${u1Token}
* outputs:
* u1Id: ${u1Entity.writeData.id}
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `create` or `update` capability
* (depending on whether the resource already exists) on the given path. If
* `disable_delete` is false, the `delete` capability is also required. If
* `disable_read` is false, the `read` capability is required.
* ## Import
* Import is not supported for this resource.
*/
public class Endpoint internal constructor(
override val javaResource: com.pulumi.vault.generic.Endpoint,
) : KotlinCustomResource(javaResource, EndpointMapper) {
/**
* String containing a JSON-encoded object that will be
* written to the given path as the secret data.
*/
public val dataJson: Output
get() = javaResource.dataJson().applyValue({ args0 -> args0 })
/**
* - (Optional) True/false. Set this to true if your
* vault authentication is not able to delete the data or if the endpoint
* does not support the `DELETE` method. Defaults to false.
*/
public val disableDelete: Output?
get() = javaResource.disableDelete().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* True/false. Set this to true if your vault
* authentication is not able to read the data or if the endpoint does
* not support the `GET` method. Setting this to `true` will break drift
* detection. You should set this to `true` for endpoints that are
* write-only. Defaults to false.
*/
public val disableRead: Output?
get() = javaResource.disableRead().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* - (Optional) True/false. If set to true,
* ignore any fields present when the endpoint is read but that were not
* in `data_json`. Also, if a field that was written is not returned when
* the endpoint is read, treat that field as being up to date. You should
* set this to `true` when writing to endpoint that, when read, returns a
* different set of fields from the ones you wrote, as is common with
* many configuration endpoints. Defaults to false.
*/
public val ignoreAbsentFields: Output?
get() = javaResource.ignoreAbsentFields().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The full logical path at which to write the given
* data. Consult each backend's documentation to see which endpoints
* support the `PUT` methods and to determine whether they also support
* `DELETE` and `GET`.
*/
public val path: Output
get() = javaResource.path().applyValue({ args0 -> args0 })
/**
* - A map whose keys are the top-level data keys
* returned from Vault by the write operation and whose values are the
* corresponding values. This map can only represent string data, so
* any non-string values returned from Vault are serialized as JSON.
* Only fields set in `write_fields` are present in the JSON data.
*/
public val writeData: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy