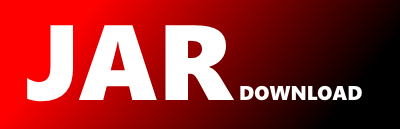
com.pulumi.vault.generic.kotlin.GenericFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.generic.kotlin
import com.pulumi.vault.generic.GenericFunctions.getSecretPlain
import com.pulumi.vault.generic.kotlin.inputs.GetSecretPlainArgs
import com.pulumi.vault.generic.kotlin.inputs.GetSecretPlainArgsBuilder
import com.pulumi.vault.generic.kotlin.outputs.GetSecretResult
import com.pulumi.vault.generic.kotlin.outputs.GetSecretResult.Companion.toKotlin
import kotlinx.coroutines.future.await
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
public object GenericFunctions {
/**
* ## Example Usage
* ### Generic secret
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const rundeckAuth = vault.generic.getSecret({
* path: "secret/rundeck_auth",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* rundeck_auth = vault.generic.get_secret(path="secret/rundeck_auth")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var rundeckAuth = Vault.Generic.GetSecret.Invoke(new()
* {
* Path = "secret/rundeck_auth",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/generic"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := generic.LookupSecret(ctx, &generic.LookupSecretArgs{
* Path: "secret/rundeck_auth",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.generic.GenericFunctions;
* import com.pulumi.vault.generic.inputs.GetSecretArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var rundeckAuth = GenericFunctions.getSecret(GetSecretArgs.builder()
* .path("secret/rundeck_auth")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* rundeckAuth:
* fn::invoke:
* Function: vault:generic:getSecret
* Arguments:
* path: secret/rundeck_auth
* ```
*
* ### KV
* For this example, consider `example` as a path for a KV engine.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* function notImplemented(message: string) {
* throw new Error(message);
* }
* const exampleCreds = vault.generic.getSecret({
* path: "example/creds",
* });
* const exampleTemplate = notImplemented("The template_file data resource is not yet supported.");
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* def not_implemented(msg):
* raise NotImplementedError(msg)
* example_creds = vault.generic.get_secret(path="example/creds")
* example_template = not_implemented("The template_file data resource is not yet supported.")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* object NotImplemented(string errorMessage)
* {
* throw new System.NotImplementedException(errorMessage);
* }
* return await Deployment.RunAsync(() =>
* {
* var exampleCreds = Vault.Generic.GetSecret.Invoke(new()
* {
* Path = "example/creds",
* });
* var exampleTemplate = NotImplemented("The template_file data resource is not yet supported.");
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/generic"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func notImplemented(message string) pulumi.AnyOutput {
* panic(message)
* }
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := generic.LookupSecret(ctx, &generic.LookupSecretArgs{
* Path: "example/creds",
* }, nil)
* if err != nil {
* return err
* }
* _ = notImplemented("The template_file data resource is not yet supported.")
* return nil
* })
* }
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `read` capability on the given path.
* @param argument A collection of arguments for invoking getSecret.
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(argument: GetSecretPlainArgs): GetSecretResult =
toKotlin(getSecretPlain(argument.toJava()).await())
/**
* @see [getSecret].
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param path The full logical path from which to request data.
* To read data from the "generic" secret backend mounted in Vault by
* default, this should be prefixed with `secret/`. Reading from other backends
* with this data source is possible; consult each backend's documentation
* to see which endpoints support the `GET` method.
* @param version The version of the secret to read. This is used by the
* Vault KV secrets engine - version 2 to indicate which version of the secret
* to read.
* @param withLeaseStartTime If set to true, stores `lease_start_time` in the TF state.
* Note that storing the `lease_start_time` in the TF state will cause a persistent drift
* on every `pulumi preview` and will require a `pulumi up`.
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(
namespace: String? = null,
path: String,
version: Int? = null,
withLeaseStartTime: Boolean? = null,
): GetSecretResult {
val argument = GetSecretPlainArgs(
namespace = namespace,
path = path,
version = version,
withLeaseStartTime = withLeaseStartTime,
)
return toKotlin(getSecretPlain(argument.toJava()).await())
}
/**
* @see [getSecret].
* @param argument Builder for [com.pulumi.vault.generic.kotlin.inputs.GetSecretPlainArgs].
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(argument: suspend GetSecretPlainArgsBuilder.() -> Unit): GetSecretResult {
val builder = GetSecretPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return toKotlin(getSecretPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy