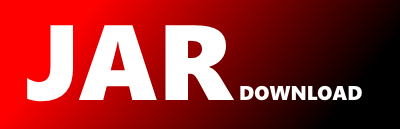
com.pulumi.vault.generic.kotlin.inputs.GetSecretPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.generic.kotlin.inputs
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.generic.inputs.GetSecretPlainArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getSecret.
* @property namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path The full logical path from which to request data.
* To read data from the "generic" secret backend mounted in Vault by
* default, this should be prefixed with `secret/`. Reading from other backends
* with this data source is possible; consult each backend's documentation
* to see which endpoints support the `GET` method.
* @property version The version of the secret to read. This is used by the
* Vault KV secrets engine - version 2 to indicate which version of the secret
* to read.
* @property withLeaseStartTime If set to true, stores `lease_start_time` in the TF state.
* Note that storing the `lease_start_time` in the TF state will cause a persistent drift
* on every `pulumi preview` and will require a `pulumi up`.
*/
public data class GetSecretPlainArgs(
public val namespace: String? = null,
public val path: String,
public val version: Int? = null,
public val withLeaseStartTime: Boolean? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.generic.inputs.GetSecretPlainArgs =
com.pulumi.vault.generic.inputs.GetSecretPlainArgs.builder()
.namespace(namespace?.let({ args0 -> args0 }))
.path(path.let({ args0 -> args0 }))
.version(version?.let({ args0 -> args0 }))
.withLeaseStartTime(withLeaseStartTime?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetSecretPlainArgs].
*/
@PulumiTagMarker
public class GetSecretPlainArgsBuilder internal constructor() {
private var namespace: String? = null
private var path: String? = null
private var version: Int? = null
private var withLeaseStartTime: Boolean? = null
/**
* @param value The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("ovjsynfptlqhyqsw")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.namespace = mapped
}
/**
* @param value The full logical path from which to request data.
* To read data from the "generic" secret backend mounted in Vault by
* default, this should be prefixed with `secret/`. Reading from other backends
* with this data source is possible; consult each backend's documentation
* to see which endpoints support the `GET` method.
*/
@JvmName("smqwxgvtprgnyyhn")
public suspend fun path(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.path = mapped
}
/**
* @param value The version of the secret to read. This is used by the
* Vault KV secrets engine - version 2 to indicate which version of the secret
* to read.
*/
@JvmName("uyvhhxamebbpkmft")
public suspend fun version(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.version = mapped
}
/**
* @param value If set to true, stores `lease_start_time` in the TF state.
* Note that storing the `lease_start_time` in the TF state will cause a persistent drift
* on every `pulumi preview` and will require a `pulumi up`.
*/
@JvmName("amdvjnpvfxtvuawm")
public suspend fun withLeaseStartTime(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.withLeaseStartTime = mapped
}
internal fun build(): GetSecretPlainArgs = GetSecretPlainArgs(
namespace = namespace,
path = path ?: throw PulumiNullFieldException("path"),
version = version,
withLeaseStartTime = withLeaseStartTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy