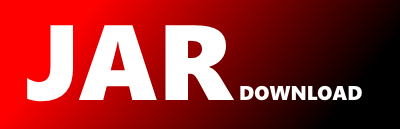
com.pulumi.vault.identity.kotlin.MfaPingidArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.identity.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.identity.MfaPingidArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource for configuring the pingid MFA method.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.identity.MfaPingid("example", {settingsFileBase64: "CnVzZV9iYXNlNjR[...]HBtCg=="});
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.identity.MfaPingid("example", settings_file_base64="CnVzZV9iYXNlNjR[...]HBtCg==")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.Identity.MfaPingid("example", new()
* {
* SettingsFileBase64 = "CnVzZV9iYXNlNjR[...]HBtCg==",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/identity"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := identity.NewMfaPingid(ctx, "example", &identity.MfaPingidArgs{
* SettingsFileBase64: pulumi.String("CnVzZV9iYXNlNjR[...]HBtCg=="),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.identity.MfaPingid;
* import com.pulumi.vault.identity.MfaPingidArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new MfaPingid("example", MfaPingidArgs.builder()
* .settingsFileBase64("CnVzZV9iYXNlNjR[...]HBtCg==")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:identity:MfaPingid
* properties:
* settingsFileBase64: CnVzZV9iYXNlNjR[...]HBtCg==
* ```
*
* ## Import
* Resource can be imported using its `uuid` field, e.g.
* ```sh
* $ pulumi import vault:identity/mfaPingid:MfaPingid example 0d89c36a-4ff5-4d70-8749-bb6a5598aeec
* ```
* @property namespace Target namespace. (requires Enterprise)
* @property settingsFileBase64 A base64-encoded third-party settings contents as retrieved from PingID's configuration page.
* @property usernameFormat A template string for mapping Identity names to MFA methods.
*/
public data class MfaPingidArgs(
public val namespace: Output? = null,
public val settingsFileBase64: Output? = null,
public val usernameFormat: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.identity.MfaPingidArgs =
com.pulumi.vault.identity.MfaPingidArgs.builder()
.namespace(namespace?.applyValue({ args0 -> args0 }))
.settingsFileBase64(settingsFileBase64?.applyValue({ args0 -> args0 }))
.usernameFormat(usernameFormat?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MfaPingidArgs].
*/
@PulumiTagMarker
public class MfaPingidArgsBuilder internal constructor() {
private var namespace: Output? = null
private var settingsFileBase64: Output? = null
private var usernameFormat: Output? = null
/**
* @param value Target namespace. (requires Enterprise)
*/
@JvmName("isjklrmyoshokuys")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value A base64-encoded third-party settings contents as retrieved from PingID's configuration page.
*/
@JvmName("aivxtepsvfifpfxt")
public suspend fun settingsFileBase64(`value`: Output) {
this.settingsFileBase64 = value
}
/**
* @param value A template string for mapping Identity names to MFA methods.
*/
@JvmName("ifhhugopupgtjgyq")
public suspend fun usernameFormat(`value`: Output) {
this.usernameFormat = value
}
/**
* @param value Target namespace. (requires Enterprise)
*/
@JvmName("ptifuipreumfejsd")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value A base64-encoded third-party settings contents as retrieved from PingID's configuration page.
*/
@JvmName("geixdbgnlotgamyd")
public suspend fun settingsFileBase64(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.settingsFileBase64 = mapped
}
/**
* @param value A template string for mapping Identity names to MFA methods.
*/
@JvmName("teheckoeeaeulinp")
public suspend fun usernameFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameFormat = mapped
}
internal fun build(): MfaPingidArgs = MfaPingidArgs(
namespace = namespace,
settingsFileBase64 = settingsFileBase64,
usernameFormat = usernameFormat,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy