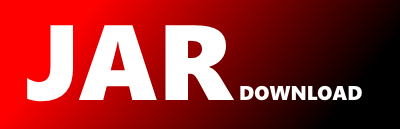
com.pulumi.vault.identity.kotlin.OidcClientArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.identity.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.identity.OidcClientArgs.builder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages OIDC Clients in a Vault server. See the [Vault documentation](https://www.vaultproject.io/api-docs/secret/identity/oidc-provider#create-or-update-an-assignment)
* for more information.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const test = new vault.identity.OidcAssignment("test", {
* name: "my-assignment",
* entityIds: ["ascbascas-2231a-sdfaa"],
* groupIds: ["sajkdsad-32414-sfsada"],
* });
* const testOidcClient = new vault.identity.OidcClient("test", {
* name: "my-app",
* redirectUris: [
* "http://127.0.0.1:9200/v1/auth-methods/oidc:authenticate:callback",
* "http://127.0.0.1:8251/callback",
* "http://127.0.0.1:8080/callback",
* ],
* assignments: [test.name],
* idTokenTtl: 2400,
* accessTokenTtl: 7200,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* test = vault.identity.OidcAssignment("test",
* name="my-assignment",
* entity_ids=["ascbascas-2231a-sdfaa"],
* group_ids=["sajkdsad-32414-sfsada"])
* test_oidc_client = vault.identity.OidcClient("test",
* name="my-app",
* redirect_uris=[
* "http://127.0.0.1:9200/v1/auth-methods/oidc:authenticate:callback",
* "http://127.0.0.1:8251/callback",
* "http://127.0.0.1:8080/callback",
* ],
* assignments=[test.name],
* id_token_ttl=2400,
* access_token_ttl=7200)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var test = new Vault.Identity.OidcAssignment("test", new()
* {
* Name = "my-assignment",
* EntityIds = new[]
* {
* "ascbascas-2231a-sdfaa",
* },
* GroupIds = new[]
* {
* "sajkdsad-32414-sfsada",
* },
* });
* var testOidcClient = new Vault.Identity.OidcClient("test", new()
* {
* Name = "my-app",
* RedirectUris = new[]
* {
* "http://127.0.0.1:9200/v1/auth-methods/oidc:authenticate:callback",
* "http://127.0.0.1:8251/callback",
* "http://127.0.0.1:8080/callback",
* },
* Assignments = new[]
* {
* test.Name,
* },
* IdTokenTtl = 2400,
* AccessTokenTtl = 7200,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/identity"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := identity.NewOidcAssignment(ctx, "test", &identity.OidcAssignmentArgs{
* Name: pulumi.String("my-assignment"),
* EntityIds: pulumi.StringArray{
* pulumi.String("ascbascas-2231a-sdfaa"),
* },
* GroupIds: pulumi.StringArray{
* pulumi.String("sajkdsad-32414-sfsada"),
* },
* })
* if err != nil {
* return err
* }
* _, err = identity.NewOidcClient(ctx, "test", &identity.OidcClientArgs{
* Name: pulumi.String("my-app"),
* RedirectUris: pulumi.StringArray{
* pulumi.String("http://127.0.0.1:9200/v1/auth-methods/oidc:authenticate:callback"),
* pulumi.String("http://127.0.0.1:8251/callback"),
* pulumi.String("http://127.0.0.1:8080/callback"),
* },
* Assignments: pulumi.StringArray{
* test.Name,
* },
* IdTokenTtl: pulumi.Int(2400),
* AccessTokenTtl: pulumi.Int(7200),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.identity.OidcAssignment;
* import com.pulumi.vault.identity.OidcAssignmentArgs;
* import com.pulumi.vault.identity.OidcClient;
* import com.pulumi.vault.identity.OidcClientArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new OidcAssignment("test", OidcAssignmentArgs.builder()
* .name("my-assignment")
* .entityIds("ascbascas-2231a-sdfaa")
* .groupIds("sajkdsad-32414-sfsada")
* .build());
* var testOidcClient = new OidcClient("testOidcClient", OidcClientArgs.builder()
* .name("my-app")
* .redirectUris(
* "http://127.0.0.1:9200/v1/auth-methods/oidc:authenticate:callback",
* "http://127.0.0.1:8251/callback",
* "http://127.0.0.1:8080/callback")
* .assignments(test.name())
* .idTokenTtl(2400)
* .accessTokenTtl(7200)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: vault:identity:OidcAssignment
* properties:
* name: my-assignment
* entityIds:
* - ascbascas-2231a-sdfaa
* groupIds:
* - sajkdsad-32414-sfsada
* testOidcClient:
* type: vault:identity:OidcClient
* name: test
* properties:
* name: my-app
* redirectUris:
* - http://127.0.0.1:9200/v1/auth-methods/oidc:authenticate:callback
* - http://127.0.0.1:8251/callback
* - http://127.0.0.1:8080/callback
* assignments:
* - ${test.name}
* idTokenTtl: 2400
* accessTokenTtl: 7200
* ```
*
* ## Import
* OIDC Clients can be imported using the `name`, e.g.
* ```sh
* $ pulumi import vault:identity/oidcClient:OidcClient test my-app
* ```
* @property accessTokenTtl The time-to-live for access tokens obtained by the client.
* @property assignments A list of assignment resources associated with the client.
* @property clientType The client type based on its ability to maintain confidentiality of credentials.
* The following client types are supported: `confidential`, `public`. Defaults to `confidential`.
* @property idTokenTtl The time-to-live for ID tokens obtained by the client.
* The value should be less than the `verification_ttl` on the key.
* @property key A reference to a named key resource in Vault.
* This cannot be modified after creation. If not provided, the `default`
* key is used.
* @property name The name of the client.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property redirectUris Redirection URI values used by the client.
* One of these values must exactly match the `redirect_uri` parameter value
* used in each authentication request.
*/
public data class OidcClientArgs(
public val accessTokenTtl: Output? = null,
public val assignments: Output>? = null,
public val clientType: Output? = null,
public val idTokenTtl: Output? = null,
public val key: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val redirectUris: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.identity.OidcClientArgs =
com.pulumi.vault.identity.OidcClientArgs.builder()
.accessTokenTtl(accessTokenTtl?.applyValue({ args0 -> args0 }))
.assignments(assignments?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.clientType(clientType?.applyValue({ args0 -> args0 }))
.idTokenTtl(idTokenTtl?.applyValue({ args0 -> args0 }))
.key(key?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.redirectUris(redirectUris?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [OidcClientArgs].
*/
@PulumiTagMarker
public class OidcClientArgsBuilder internal constructor() {
private var accessTokenTtl: Output? = null
private var assignments: Output>? = null
private var clientType: Output? = null
private var idTokenTtl: Output? = null
private var key: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var redirectUris: Output>? = null
/**
* @param value The time-to-live for access tokens obtained by the client.
*/
@JvmName("njleuliljppmtbsu")
public suspend fun accessTokenTtl(`value`: Output) {
this.accessTokenTtl = value
}
/**
* @param value A list of assignment resources associated with the client.
*/
@JvmName("wrtrrovauvtorfcf")
public suspend fun assignments(`value`: Output>) {
this.assignments = value
}
@JvmName("ypdgbcwrgikjlkma")
public suspend fun assignments(vararg values: Output) {
this.assignments = Output.all(values.asList())
}
/**
* @param values A list of assignment resources associated with the client.
*/
@JvmName("yqixshkyghkspqjc")
public suspend fun assignments(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy