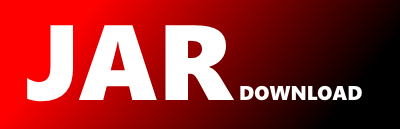
com.pulumi.vault.identity.kotlin.OidcKeyAllowedClientIDArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.identity.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.identity.OidcKeyAllowedClientIDArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const key = new vault.identity.OidcKey("key", {
* name: "key",
* algorithm: "RS256",
* });
* const role = new vault.identity.OidcRole("role", {
* name: "role",
* key: key.name,
* });
* const roleOidcKeyAllowedClientID = new vault.identity.OidcKeyAllowedClientID("role", {
* keyName: key.name,
* allowedClientId: role.clientId,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* key = vault.identity.OidcKey("key",
* name="key",
* algorithm="RS256")
* role = vault.identity.OidcRole("role",
* name="role",
* key=key.name)
* role_oidc_key_allowed_client_id = vault.identity.OidcKeyAllowedClientID("role",
* key_name=key.name,
* allowed_client_id=role.client_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var key = new Vault.Identity.OidcKey("key", new()
* {
* Name = "key",
* Algorithm = "RS256",
* });
* var role = new Vault.Identity.OidcRole("role", new()
* {
* Name = "role",
* Key = key.Name,
* });
* var roleOidcKeyAllowedClientID = new Vault.Identity.OidcKeyAllowedClientID("role", new()
* {
* KeyName = key.Name,
* AllowedClientId = role.ClientId,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/identity"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* key, err := identity.NewOidcKey(ctx, "key", &identity.OidcKeyArgs{
* Name: pulumi.String("key"),
* Algorithm: pulumi.String("RS256"),
* })
* if err != nil {
* return err
* }
* role, err := identity.NewOidcRole(ctx, "role", &identity.OidcRoleArgs{
* Name: pulumi.String("role"),
* Key: key.Name,
* })
* if err != nil {
* return err
* }
* _, err = identity.NewOidcKeyAllowedClientID(ctx, "role", &identity.OidcKeyAllowedClientIDArgs{
* KeyName: key.Name,
* AllowedClientId: role.ClientId,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.identity.OidcKey;
* import com.pulumi.vault.identity.OidcKeyArgs;
* import com.pulumi.vault.identity.OidcRole;
* import com.pulumi.vault.identity.OidcRoleArgs;
* import com.pulumi.vault.identity.OidcKeyAllowedClientID;
* import com.pulumi.vault.identity.OidcKeyAllowedClientIDArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var key = new OidcKey("key", OidcKeyArgs.builder()
* .name("key")
* .algorithm("RS256")
* .build());
* var role = new OidcRole("role", OidcRoleArgs.builder()
* .name("role")
* .key(key.name())
* .build());
* var roleOidcKeyAllowedClientID = new OidcKeyAllowedClientID("roleOidcKeyAllowedClientID", OidcKeyAllowedClientIDArgs.builder()
* .keyName(key.name())
* .allowedClientId(role.clientId())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* key:
* type: vault:identity:OidcKey
* properties:
* name: key
* algorithm: RS256
* role:
* type: vault:identity:OidcRole
* properties:
* name: role
* key: ${key.name}
* roleOidcKeyAllowedClientID:
* type: vault:identity:OidcKeyAllowedClientID
* name: role
* properties:
* keyName: ${key.name}
* allowedClientId: ${role.clientId}
* ```
*
* @property allowedClientId Client ID to allow usage with the OIDC named key
* @property keyName Name of the OIDC Key allow the Client ID.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public data class OidcKeyAllowedClientIDArgs(
public val allowedClientId: Output? = null,
public val keyName: Output? = null,
public val namespace: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.identity.OidcKeyAllowedClientIDArgs =
com.pulumi.vault.identity.OidcKeyAllowedClientIDArgs.builder()
.allowedClientId(allowedClientId?.applyValue({ args0 -> args0 }))
.keyName(keyName?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [OidcKeyAllowedClientIDArgs].
*/
@PulumiTagMarker
public class OidcKeyAllowedClientIDArgsBuilder internal constructor() {
private var allowedClientId: Output? = null
private var keyName: Output? = null
private var namespace: Output? = null
/**
* @param value Client ID to allow usage with the OIDC named key
*/
@JvmName("ebjgsedfsdyofpyv")
public suspend fun allowedClientId(`value`: Output) {
this.allowedClientId = value
}
/**
* @param value Name of the OIDC Key allow the Client ID.
*/
@JvmName("dyxqrvydlqiamcgg")
public suspend fun keyName(`value`: Output) {
this.keyName = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("wgoskauysklduryu")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Client ID to allow usage with the OIDC named key
*/
@JvmName("mcwsrgjdqjpvqufs")
public suspend fun allowedClientId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowedClientId = mapped
}
/**
* @param value Name of the OIDC Key allow the Client ID.
*/
@JvmName("voddblmsxwtsymft")
public suspend fun keyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyName = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("bxauiwgmpcuwtewj")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
internal fun build(): OidcKeyAllowedClientIDArgs = OidcKeyAllowedClientIDArgs(
allowedClientId = allowedClientId,
keyName = keyName,
namespace = namespace,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy