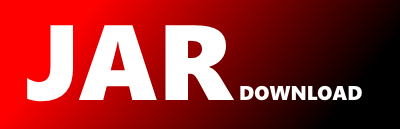
com.pulumi.vault.identity.kotlin.inputs.GetGroupPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.identity.kotlin.inputs
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.identity.inputs.GetGroupPlainArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getGroup.
* @property aliasId ID of the alias.
* @property aliasMountAccessor Accessor of the mount to which the alias belongs to.
* This should be supplied in conjunction with `alias_name`.
* The lookup criteria can be `group_name`, `group_id`, `alias_id`, or a combination of
* `alias_name` and `alias_mount_accessor`.
* @property aliasName Name of the alias. This should be supplied in conjunction with
* `alias_mount_accessor`.
* @property groupId ID of the group.
* @property groupName Name of the group.
* @property namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public data class GetGroupPlainArgs(
public val aliasId: String? = null,
public val aliasMountAccessor: String? = null,
public val aliasName: String? = null,
public val groupId: String? = null,
public val groupName: String? = null,
public val namespace: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.identity.inputs.GetGroupPlainArgs =
com.pulumi.vault.identity.inputs.GetGroupPlainArgs.builder()
.aliasId(aliasId?.let({ args0 -> args0 }))
.aliasMountAccessor(aliasMountAccessor?.let({ args0 -> args0 }))
.aliasName(aliasName?.let({ args0 -> args0 }))
.groupId(groupId?.let({ args0 -> args0 }))
.groupName(groupName?.let({ args0 -> args0 }))
.namespace(namespace?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetGroupPlainArgs].
*/
@PulumiTagMarker
public class GetGroupPlainArgsBuilder internal constructor() {
private var aliasId: String? = null
private var aliasMountAccessor: String? = null
private var aliasName: String? = null
private var groupId: String? = null
private var groupName: String? = null
private var namespace: String? = null
/**
* @param value ID of the alias.
*/
@JvmName("smjeauqftdjdghfp")
public suspend fun aliasId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.aliasId = mapped
}
/**
* @param value Accessor of the mount to which the alias belongs to.
* This should be supplied in conjunction with `alias_name`.
* The lookup criteria can be `group_name`, `group_id`, `alias_id`, or a combination of
* `alias_name` and `alias_mount_accessor`.
*/
@JvmName("qkxrdbeyoktdvlsh")
public suspend fun aliasMountAccessor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.aliasMountAccessor = mapped
}
/**
* @param value Name of the alias. This should be supplied in conjunction with
* `alias_mount_accessor`.
*/
@JvmName("rwermoclktacjbya")
public suspend fun aliasName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.aliasName = mapped
}
/**
* @param value ID of the group.
*/
@JvmName("nnvxxyhwsqkiyjbd")
public suspend fun groupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.groupId = mapped
}
/**
* @param value Name of the group.
*/
@JvmName("jcxcxvqwkmtyobri")
public suspend fun groupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.groupName = mapped
}
/**
* @param value The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("puhtanrrwcxofmqq")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.namespace = mapped
}
internal fun build(): GetGroupPlainArgs = GetGroupPlainArgs(
aliasId = aliasId,
aliasMountAccessor = aliasMountAccessor,
aliasName = aliasName,
groupId = groupId,
groupName = groupName,
namespace = namespace,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy