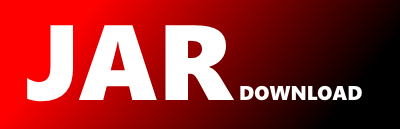
com.pulumi.vault.jwt.kotlin.AuthBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.jwt.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.jwt.AuthBackendArgs.builder
import com.pulumi.vault.jwt.kotlin.inputs.AuthBackendTuneArgs
import com.pulumi.vault.jwt.kotlin.inputs.AuthBackendTuneArgsBuilder
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a resource for managing an
* [JWT auth backend within Vault](https://www.vaultproject.io/docs/auth/jwt.html).
* ## Example Usage
* Manage JWT auth backend:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.jwt.AuthBackend("example", {
* description: "Demonstration of the Terraform JWT auth backend",
* path: "jwt",
* oidcDiscoveryUrl: "https://myco.auth0.com/",
* boundIssuer: "https://myco.auth0.com/",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.jwt.AuthBackend("example",
* description="Demonstration of the Terraform JWT auth backend",
* path="jwt",
* oidc_discovery_url="https://myco.auth0.com/",
* bound_issuer="https://myco.auth0.com/")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.Jwt.AuthBackend("example", new()
* {
* Description = "Demonstration of the Terraform JWT auth backend",
* Path = "jwt",
* OidcDiscoveryUrl = "https://myco.auth0.com/",
* BoundIssuer = "https://myco.auth0.com/",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/jwt"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := jwt.NewAuthBackend(ctx, "example", &jwt.AuthBackendArgs{
* Description: pulumi.String("Demonstration of the Terraform JWT auth backend"),
* Path: pulumi.String("jwt"),
* OidcDiscoveryUrl: pulumi.String("https://myco.auth0.com/"),
* BoundIssuer: pulumi.String("https://myco.auth0.com/"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.jwt.AuthBackend;
* import com.pulumi.vault.jwt.AuthBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AuthBackend("example", AuthBackendArgs.builder()
* .description("Demonstration of the Terraform JWT auth backend")
* .path("jwt")
* .oidcDiscoveryUrl("https://myco.auth0.com/")
* .boundIssuer("https://myco.auth0.com/")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:jwt:AuthBackend
* properties:
* description: Demonstration of the Terraform JWT auth backend
* path: jwt
* oidcDiscoveryUrl: https://myco.auth0.com/
* boundIssuer: https://myco.auth0.com/
* ```
*
* Manage OIDC auth backend:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.jwt.AuthBackend("example", {
* description: "Demonstration of the Terraform JWT auth backend",
* path: "oidc",
* type: "oidc",
* oidcDiscoveryUrl: "https://myco.auth0.com/",
* oidcClientId: "1234567890",
* oidcClientSecret: "secret123456",
* boundIssuer: "https://myco.auth0.com/",
* tune: {
* listingVisibility: "unauth",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.jwt.AuthBackend("example",
* description="Demonstration of the Terraform JWT auth backend",
* path="oidc",
* type="oidc",
* oidc_discovery_url="https://myco.auth0.com/",
* oidc_client_id="1234567890",
* oidc_client_secret="secret123456",
* bound_issuer="https://myco.auth0.com/",
* tune=vault.jwt.AuthBackendTuneArgs(
* listing_visibility="unauth",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.Jwt.AuthBackend("example", new()
* {
* Description = "Demonstration of the Terraform JWT auth backend",
* Path = "oidc",
* Type = "oidc",
* OidcDiscoveryUrl = "https://myco.auth0.com/",
* OidcClientId = "1234567890",
* OidcClientSecret = "secret123456",
* BoundIssuer = "https://myco.auth0.com/",
* Tune = new Vault.Jwt.Inputs.AuthBackendTuneArgs
* {
* ListingVisibility = "unauth",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/jwt"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := jwt.NewAuthBackend(ctx, "example", &jwt.AuthBackendArgs{
* Description: pulumi.String("Demonstration of the Terraform JWT auth backend"),
* Path: pulumi.String("oidc"),
* Type: pulumi.String("oidc"),
* OidcDiscoveryUrl: pulumi.String("https://myco.auth0.com/"),
* OidcClientId: pulumi.String("1234567890"),
* OidcClientSecret: pulumi.String("secret123456"),
* BoundIssuer: pulumi.String("https://myco.auth0.com/"),
* Tune: &jwt.AuthBackendTuneArgs{
* ListingVisibility: pulumi.String("unauth"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.jwt.AuthBackend;
* import com.pulumi.vault.jwt.AuthBackendArgs;
* import com.pulumi.vault.jwt.inputs.AuthBackendTuneArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AuthBackend("example", AuthBackendArgs.builder()
* .description("Demonstration of the Terraform JWT auth backend")
* .path("oidc")
* .type("oidc")
* .oidcDiscoveryUrl("https://myco.auth0.com/")
* .oidcClientId("1234567890")
* .oidcClientSecret("secret123456")
* .boundIssuer("https://myco.auth0.com/")
* .tune(AuthBackendTuneArgs.builder()
* .listingVisibility("unauth")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:jwt:AuthBackend
* properties:
* description: Demonstration of the Terraform JWT auth backend
* path: oidc
* type: oidc
* oidcDiscoveryUrl: https://myco.auth0.com/
* oidcClientId: '1234567890'
* oidcClientSecret: secret123456
* boundIssuer: https://myco.auth0.com/
* tune:
* listingVisibility: unauth
* ```
*
* Configuring the auth backend with a `provider_config:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const gsuite = new vault.jwt.AuthBackend("gsuite", {
* description: "OIDC backend",
* oidcDiscoveryUrl: "https://accounts.google.com",
* path: "oidc",
* type: "oidc",
* providerConfig: {
* provider: "gsuite",
* fetch_groups: "true",
* fetch_user_info: "true",
* groups_recurse_max_depth: "1",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* gsuite = vault.jwt.AuthBackend("gsuite",
* description="OIDC backend",
* oidc_discovery_url="https://accounts.google.com",
* path="oidc",
* type="oidc",
* provider_config={
* "provider": "gsuite",
* "fetch_groups": "true",
* "fetch_user_info": "true",
* "groups_recurse_max_depth": "1",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var gsuite = new Vault.Jwt.AuthBackend("gsuite", new()
* {
* Description = "OIDC backend",
* OidcDiscoveryUrl = "https://accounts.google.com",
* Path = "oidc",
* Type = "oidc",
* ProviderConfig =
* {
* { "provider", "gsuite" },
* { "fetch_groups", "true" },
* { "fetch_user_info", "true" },
* { "groups_recurse_max_depth", "1" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/jwt"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := jwt.NewAuthBackend(ctx, "gsuite", &jwt.AuthBackendArgs{
* Description: pulumi.String("OIDC backend"),
* OidcDiscoveryUrl: pulumi.String("https://accounts.google.com"),
* Path: pulumi.String("oidc"),
* Type: pulumi.String("oidc"),
* ProviderConfig: pulumi.StringMap{
* "provider": pulumi.String("gsuite"),
* "fetch_groups": pulumi.String("true"),
* "fetch_user_info": pulumi.String("true"),
* "groups_recurse_max_depth": pulumi.String("1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.jwt.AuthBackend;
* import com.pulumi.vault.jwt.AuthBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var gsuite = new AuthBackend("gsuite", AuthBackendArgs.builder()
* .description("OIDC backend")
* .oidcDiscoveryUrl("https://accounts.google.com")
* .path("oidc")
* .type("oidc")
* .providerConfig(Map.ofEntries(
* Map.entry("provider", "gsuite"),
* Map.entry("fetch_groups", true),
* Map.entry("fetch_user_info", true),
* Map.entry("groups_recurse_max_depth", 1)
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* gsuite:
* type: vault:jwt:AuthBackend
* properties:
* description: OIDC backend
* oidcDiscoveryUrl: https://accounts.google.com
* path: oidc
* type: oidc
* providerConfig:
* provider: gsuite
* fetch_groups: true
* fetch_user_info: true
* groups_recurse_max_depth: 1
* ```
*
* ## Import
* JWT auth backend can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:jwt/authBackend:AuthBackend oidc oidc
* ```
* or
* ```sh
* $ pulumi import vault:jwt/authBackend:AuthBackend jwt jwt
* ```
* @property boundIssuer The value against which to match the iss claim in a JWT
* @property defaultRole The default role to use if none is provided during login
* @property description The description of the auth backend
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property jwksCaPem The CA certificate or chain of certificates, in PEM format, to use to validate connections to the JWKS URL. If not set, system certificates are used.
* @property jwksUrl JWKS URL to use to authenticate signatures. Cannot be used with "oidc_discovery_url" or "jwt_validation_pubkeys".
* @property jwtSupportedAlgs A list of supported signing algorithms. Vault 1.1.0 defaults to [RS256] but future or past versions of Vault may differ
* @property jwtValidationPubkeys A list of PEM-encoded public keys to use to authenticate signatures locally. Cannot be used in combination with `oidc_discovery_url`
* @property local Specifies if the auth method is local only.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property namespaceInState Pass namespace in the OIDC state parameter instead of as a separate query parameter. With this setting, the allowed redirect URL(s) in Vault and on the provider side should not contain a namespace query parameter. This means only one redirect URL entry needs to be maintained on the OIDC provider side for all vault namespaces that will be authenticating against it. Defaults to true for new configs
* * tune - (Optional) Extra configuration block. Structure is documented below.
* The `tune` block is used to tune the auth backend:
* @property oidcClientId Client ID used for OIDC backends
* @property oidcClientSecret Client Secret used for OIDC backends
* @property oidcDiscoveryCaPem The CA certificate or chain of certificates, in PEM format, to use to validate connections to the OIDC Discovery URL. If not set, system certificates are used
* @property oidcDiscoveryUrl The OIDC Discovery URL, without any .well-known component (base path). Cannot be used in combination with `jwt_validation_pubkeys`
* @property oidcResponseMode The response mode to be used in the OAuth2 request. Allowed values are `query` and `form_post`. Defaults to `query`. If using Vault namespaces, and `oidc_response_mode` is `form_post`, then `namespace_in_state` should be set to `false`.
* @property oidcResponseTypes List of response types to request. Allowed values are 'code' and 'id_token'. Defaults to `["code"]`. Note: `id_token` may only be used if `oidc_response_mode` is set to `form_post`.
* @property path Path to mount the JWT/OIDC auth backend
* @property providerConfig Provider specific handling configuration. All values may be strings, and the provider will convert to the appropriate type when configuring Vault.
* @property tune
* @property type Type of auth backend. Should be one of `jwt` or `oidc`. Default - `jwt`
*/
public data class AuthBackendArgs(
public val boundIssuer: Output? = null,
public val defaultRole: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val jwksCaPem: Output? = null,
public val jwksUrl: Output? = null,
public val jwtSupportedAlgs: Output>? = null,
public val jwtValidationPubkeys: Output>? = null,
public val local: Output? = null,
public val namespace: Output? = null,
public val namespaceInState: Output? = null,
public val oidcClientId: Output? = null,
public val oidcClientSecret: Output? = null,
public val oidcDiscoveryCaPem: Output? = null,
public val oidcDiscoveryUrl: Output? = null,
public val oidcResponseMode: Output? = null,
public val oidcResponseTypes: Output>? = null,
public val path: Output? = null,
public val providerConfig: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy