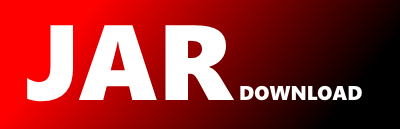
com.pulumi.vault.kmip.kotlin.SecretBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kmip.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.kmip.SecretBackendArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages KMIP Secret backends in a Vault server. This feature requires
* Vault Enterprise. See the [Vault documentation](https://www.vaultproject.io/docs/secrets/kmip)
* for more information.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const _default = new vault.kmip.SecretBackend("default", {
* path: "kmip",
* description: "Vault KMIP backend",
* listenAddrs: [
* "127.0.0.1:5696",
* "127.0.0.1:8080",
* ],
* tlsCaKeyType: "rsa",
* tlsCaKeyBits: 4096,
* defaultTlsClientKeyType: "rsa",
* defaultTlsClientKeyBits: 4096,
* defaultTlsClientTtl: 86400,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* default = vault.kmip.SecretBackend("default",
* path="kmip",
* description="Vault KMIP backend",
* listen_addrs=[
* "127.0.0.1:5696",
* "127.0.0.1:8080",
* ],
* tls_ca_key_type="rsa",
* tls_ca_key_bits=4096,
* default_tls_client_key_type="rsa",
* default_tls_client_key_bits=4096,
* default_tls_client_ttl=86400)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Vault.Kmip.SecretBackend("default", new()
* {
* Path = "kmip",
* Description = "Vault KMIP backend",
* ListenAddrs = new[]
* {
* "127.0.0.1:5696",
* "127.0.0.1:8080",
* },
* TlsCaKeyType = "rsa",
* TlsCaKeyBits = 4096,
* DefaultTlsClientKeyType = "rsa",
* DefaultTlsClientKeyBits = 4096,
* DefaultTlsClientTtl = 86400,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kmip"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kmip.NewSecretBackend(ctx, "default", &kmip.SecretBackendArgs{
* Path: pulumi.String("kmip"),
* Description: pulumi.String("Vault KMIP backend"),
* ListenAddrs: pulumi.StringArray{
* pulumi.String("127.0.0.1:5696"),
* pulumi.String("127.0.0.1:8080"),
* },
* TlsCaKeyType: pulumi.String("rsa"),
* TlsCaKeyBits: pulumi.Int(4096),
* DefaultTlsClientKeyType: pulumi.String("rsa"),
* DefaultTlsClientKeyBits: pulumi.Int(4096),
* DefaultTlsClientTtl: pulumi.Int(86400),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.kmip.SecretBackend;
* import com.pulumi.vault.kmip.SecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new SecretBackend("default", SecretBackendArgs.builder()
* .path("kmip")
* .description("Vault KMIP backend")
* .listenAddrs(
* "127.0.0.1:5696",
* "127.0.0.1:8080")
* .tlsCaKeyType("rsa")
* .tlsCaKeyBits(4096)
* .defaultTlsClientKeyType("rsa")
* .defaultTlsClientKeyBits(4096)
* .defaultTlsClientTtl(86400)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: vault:kmip:SecretBackend
* properties:
* path: kmip
* description: Vault KMIP backend
* listenAddrs:
* - 127.0.0.1:5696
* - 127.0.0.1:8080
* tlsCaKeyType: rsa
* tlsCaKeyBits: 4096
* defaultTlsClientKeyType: rsa
* defaultTlsClientKeyBits: 4096
* defaultTlsClientTtl: 86400
* ```
*
* ## Import
* KMIP Secret backend can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:kmip/secretBackend:SecretBackend default kmip
* ```
* @property defaultTlsClientKeyBits Client certificate key bits, valid values depend on key type.
* @property defaultTlsClientKeyType Client certificate key type, `rsa` or `ec`.
* @property defaultTlsClientTtl Client certificate TTL in seconds
* @property description A human-friendly description for this backend.
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property listenAddrs Addresses the KMIP server should listen on (`host:port`).
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `kmip`.
* @property serverHostnames Hostnames to include in the server's TLS certificate as SAN DNS names. The first will be used as the common name (CN).
* @property serverIps IPs to include in the server's TLS certificate as SAN IP addresses.
* @property tlsCaKeyBits CA key bits, valid values depend on key type.
* @property tlsCaKeyType CA key type, rsa or ec.
* @property tlsMinVersion Minimum TLS version to accept.
*/
public data class SecretBackendArgs(
public val defaultTlsClientKeyBits: Output? = null,
public val defaultTlsClientKeyType: Output? = null,
public val defaultTlsClientTtl: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val listenAddrs: Output>? = null,
public val namespace: Output? = null,
public val path: Output? = null,
public val serverHostnames: Output>? = null,
public val serverIps: Output>? = null,
public val tlsCaKeyBits: Output? = null,
public val tlsCaKeyType: Output? = null,
public val tlsMinVersion: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.kmip.SecretBackendArgs =
com.pulumi.vault.kmip.SecretBackendArgs.builder()
.defaultTlsClientKeyBits(defaultTlsClientKeyBits?.applyValue({ args0 -> args0 }))
.defaultTlsClientKeyType(defaultTlsClientKeyType?.applyValue({ args0 -> args0 }))
.defaultTlsClientTtl(defaultTlsClientTtl?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.listenAddrs(listenAddrs?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.serverHostnames(serverHostnames?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.serverIps(serverIps?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tlsCaKeyBits(tlsCaKeyBits?.applyValue({ args0 -> args0 }))
.tlsCaKeyType(tlsCaKeyType?.applyValue({ args0 -> args0 }))
.tlsMinVersion(tlsMinVersion?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendArgs].
*/
@PulumiTagMarker
public class SecretBackendArgsBuilder internal constructor() {
private var defaultTlsClientKeyBits: Output? = null
private var defaultTlsClientKeyType: Output? = null
private var defaultTlsClientTtl: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var listenAddrs: Output>? = null
private var namespace: Output? = null
private var path: Output? = null
private var serverHostnames: Output>? = null
private var serverIps: Output>? = null
private var tlsCaKeyBits: Output? = null
private var tlsCaKeyType: Output? = null
private var tlsMinVersion: Output? = null
/**
* @param value Client certificate key bits, valid values depend on key type.
*/
@JvmName("pxvcxhhykraqekiu")
public suspend fun defaultTlsClientKeyBits(`value`: Output) {
this.defaultTlsClientKeyBits = value
}
/**
* @param value Client certificate key type, `rsa` or `ec`.
*/
@JvmName("agxuqnxrhpeowlrf")
public suspend fun defaultTlsClientKeyType(`value`: Output) {
this.defaultTlsClientKeyType = value
}
/**
* @param value Client certificate TTL in seconds
*/
@JvmName("dciyjuqefebskqvi")
public suspend fun defaultTlsClientTtl(`value`: Output) {
this.defaultTlsClientTtl = value
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("nxfmcbokiitrfuto")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("iemdmyaupkdjesma")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value Addresses the KMIP server should listen on (`host:port`).
*/
@JvmName("bpvigamugvupntlt")
public suspend fun listenAddrs(`value`: Output>) {
this.listenAddrs = value
}
@JvmName("assfkneqdhjfxqxg")
public suspend fun listenAddrs(vararg values: Output) {
this.listenAddrs = Output.all(values.asList())
}
/**
* @param values Addresses the KMIP server should listen on (`host:port`).
*/
@JvmName("lnsmmtpkebfpqlms")
public suspend fun listenAddrs(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy