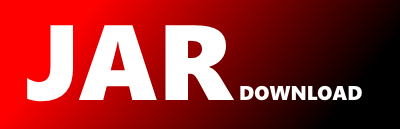
com.pulumi.vault.kotlin.Audit.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [Audit].
*/
@PulumiTagMarker
public class AuditResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuditArgs = AuditArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuditArgsBuilder.() -> Unit) {
val builder = AuditArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Audit {
val builtJavaResource = com.pulumi.vault.Audit(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Audit(builtJavaResource)
}
}
/**
* ## Example Usage
* ### File Audit Device)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const test = new vault.Audit("test", {
* type: "file",
* options: {
* file_path: "C:/temp/audit.txt",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* test = vault.Audit("test",
* type="file",
* options={
* "file_path": "C:/temp/audit.txt",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var test = new Vault.Audit("test", new()
* {
* Type = "file",
* Options =
* {
* { "file_path", "C:/temp/audit.txt" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vault.NewAudit(ctx, "test", &vault.AuditArgs{
* Type: pulumi.String("file"),
* Options: pulumi.StringMap{
* "file_path": pulumi.String("C:/temp/audit.txt"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Audit;
* import com.pulumi.vault.AuditArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new Audit("test", AuditArgs.builder()
* .type("file")
* .options(Map.of("file_path", "C:/temp/audit.txt"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: vault:Audit
* properties:
* type: file
* options:
* file_path: C:/temp/audit.txt
* ```
*
* ### Socket Audit Device)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const test = new vault.Audit("test", {
* type: "socket",
* path: "app_socket",
* local: false,
* options: {
* address: "127.0.0.1:8000",
* socket_type: "tcp",
* description: "application x socket",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* test = vault.Audit("test",
* type="socket",
* path="app_socket",
* local=False,
* options={
* "address": "127.0.0.1:8000",
* "socket_type": "tcp",
* "description": "application x socket",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var test = new Vault.Audit("test", new()
* {
* Type = "socket",
* Path = "app_socket",
* Local = false,
* Options =
* {
* { "address", "127.0.0.1:8000" },
* { "socket_type", "tcp" },
* { "description", "application x socket" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vault.NewAudit(ctx, "test", &vault.AuditArgs{
* Type: pulumi.String("socket"),
* Path: pulumi.String("app_socket"),
* Local: pulumi.Bool(false),
* Options: pulumi.StringMap{
* "address": pulumi.String("127.0.0.1:8000"),
* "socket_type": pulumi.String("tcp"),
* "description": pulumi.String("application x socket"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Audit;
* import com.pulumi.vault.AuditArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new Audit("test", AuditArgs.builder()
* .type("socket")
* .path("app_socket")
* .local(false)
* .options(Map.ofEntries(
* Map.entry("address", "127.0.0.1:8000"),
* Map.entry("socket_type", "tcp"),
* Map.entry("description", "application x socket")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: vault:Audit
* properties:
* type: socket
* path: app_socket
* local: false
* options:
* address: 127.0.0.1:8000
* socket_type: tcp
* description: application x socket
* ```
*
* ## Import
* Audit devices can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:index/audit:Audit test syslog
* ```
*/
public class Audit internal constructor(
override val javaResource: com.pulumi.vault.Audit,
) : KotlinCustomResource(javaResource, AuditMapper) {
/**
* Human-friendly description of the audit device.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies if the audit device is a local only. Local audit devices are not replicated nor (if a secondary) removed by replication.
*/
public val local: Output?
get() = javaResource.local().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Configuration options to pass to the audit device itself.
* For a reference of the device types and their options, consult the [Vault documentation.](https://www.vaultproject.io/docs/audit/index.html)
*/
public val options: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy