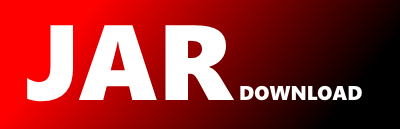
com.pulumi.vault.kotlin.MfaDuoArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.MfaDuoArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a resource to manage [Duo MFA](https://www.vaultproject.io/docs/enterprise/mfa/mfa-duo.html).
* **Note** this feature is available only with Vault Enterprise.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const userpass = new vault.AuthBackend("userpass", {
* type: "userpass",
* path: "userpass",
* });
* const myDuo = new vault.MfaDuo("my_duo", {
* name: "my_duo",
* mountAccessor: userpass.accessor,
* secretKey: "8C7THtrIigh2rPZQMbguugt8IUftWhMRCOBzbuyz",
* integrationKey: "BIACEUEAXI20BNWTEYXT",
* apiHostname: "api-2b5c39f5.duosecurity.com",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* userpass = vault.AuthBackend("userpass",
* type="userpass",
* path="userpass")
* my_duo = vault.MfaDuo("my_duo",
* name="my_duo",
* mount_accessor=userpass.accessor,
* secret_key="8C7THtrIigh2rPZQMbguugt8IUftWhMRCOBzbuyz",
* integration_key="BIACEUEAXI20BNWTEYXT",
* api_hostname="api-2b5c39f5.duosecurity.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var userpass = new Vault.AuthBackend("userpass", new()
* {
* Type = "userpass",
* Path = "userpass",
* });
* var myDuo = new Vault.MfaDuo("my_duo", new()
* {
* Name = "my_duo",
* MountAccessor = userpass.Accessor,
* SecretKey = "8C7THtrIigh2rPZQMbguugt8IUftWhMRCOBzbuyz",
* IntegrationKey = "BIACEUEAXI20BNWTEYXT",
* ApiHostname = "api-2b5c39f5.duosecurity.com",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* userpass, err := vault.NewAuthBackend(ctx, "userpass", &vault.AuthBackendArgs{
* Type: pulumi.String("userpass"),
* Path: pulumi.String("userpass"),
* })
* if err != nil {
* return err
* }
* _, err = vault.NewMfaDuo(ctx, "my_duo", &vault.MfaDuoArgs{
* Name: pulumi.String("my_duo"),
* MountAccessor: userpass.Accessor,
* SecretKey: pulumi.String("8C7THtrIigh2rPZQMbguugt8IUftWhMRCOBzbuyz"),
* IntegrationKey: pulumi.String("BIACEUEAXI20BNWTEYXT"),
* ApiHostname: pulumi.String("api-2b5c39f5.duosecurity.com"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.MfaDuo;
* import com.pulumi.vault.MfaDuoArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var userpass = new AuthBackend("userpass", AuthBackendArgs.builder()
* .type("userpass")
* .path("userpass")
* .build());
* var myDuo = new MfaDuo("myDuo", MfaDuoArgs.builder()
* .name("my_duo")
* .mountAccessor(userpass.accessor())
* .secretKey("8C7THtrIigh2rPZQMbguugt8IUftWhMRCOBzbuyz")
* .integrationKey("BIACEUEAXI20BNWTEYXT")
* .apiHostname("api-2b5c39f5.duosecurity.com")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* userpass:
* type: vault:AuthBackend
* properties:
* type: userpass
* path: userpass
* myDuo:
* type: vault:MfaDuo
* name: my_duo
* properties:
* name: my_duo
* mountAccessor: ${userpass.accessor}
* secretKey: 8C7THtrIigh2rPZQMbguugt8IUftWhMRCOBzbuyz
* integrationKey: BIACEUEAXI20BNWTEYXT
* apiHostname: api-2b5c39f5.duosecurity.com
* ```
*
* ## Import
* Mounts can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:index/mfaDuo:MfaDuo my_duo my_duo
* ```
* @property apiHostname `(string: )` - API hostname for Duo.
* @property integrationKey `(string: )` - Integration key for Duo.
* @property mountAccessor `(string: )` - The mount to tie this method to for use in automatic mappings. The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
* @property name `(string: )` – Name of the MFA method.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property pushInfo `(string)` - Push information for Duo.
* @property secretKey `(string: )` - Secret key for Duo.
* @property usernameFormat `(string)` - A format string for mapping Identity names to MFA method names. Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`. If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
public data class MfaDuoArgs(
public val apiHostname: Output? = null,
public val integrationKey: Output? = null,
public val mountAccessor: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val pushInfo: Output? = null,
public val secretKey: Output? = null,
public val usernameFormat: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.MfaDuoArgs = com.pulumi.vault.MfaDuoArgs.builder()
.apiHostname(apiHostname?.applyValue({ args0 -> args0 }))
.integrationKey(integrationKey?.applyValue({ args0 -> args0 }))
.mountAccessor(mountAccessor?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.pushInfo(pushInfo?.applyValue({ args0 -> args0 }))
.secretKey(secretKey?.applyValue({ args0 -> args0 }))
.usernameFormat(usernameFormat?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MfaDuoArgs].
*/
@PulumiTagMarker
public class MfaDuoArgsBuilder internal constructor() {
private var apiHostname: Output? = null
private var integrationKey: Output? = null
private var mountAccessor: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var pushInfo: Output? = null
private var secretKey: Output? = null
private var usernameFormat: Output? = null
/**
* @param value `(string: )` - API hostname for Duo.
*/
@JvmName("ugijadeyggrkqpof")
public suspend fun apiHostname(`value`: Output) {
this.apiHostname = value
}
/**
* @param value `(string: )` - Integration key for Duo.
*/
@JvmName("nodtrqkgcjvsxjab")
public suspend fun integrationKey(`value`: Output) {
this.integrationKey = value
}
/**
* @param value `(string: )` - The mount to tie this method to for use in automatic mappings. The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*/
@JvmName("kydlxfafssujhodn")
public suspend fun mountAccessor(`value`: Output) {
this.mountAccessor = value
}
/**
* @param value `(string: )` – Name of the MFA method.
*/
@JvmName("smlenpeomdkksrvb")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("vqigrfucaqckpyxu")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value `(string)` - Push information for Duo.
*/
@JvmName("qaesdefuharwtico")
public suspend fun pushInfo(`value`: Output) {
this.pushInfo = value
}
/**
* @param value `(string: )` - Secret key for Duo.
*/
@JvmName("jddqjupbfapdkuiu")
public suspend fun secretKey(`value`: Output) {
this.secretKey = value
}
/**
* @param value `(string)` - A format string for mapping Identity names to MFA method names. Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`. If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
@JvmName("qljpvugfuktxsxex")
public suspend fun usernameFormat(`value`: Output) {
this.usernameFormat = value
}
/**
* @param value `(string: )` - API hostname for Duo.
*/
@JvmName("hbtdtifxohrvfgbg")
public suspend fun apiHostname(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiHostname = mapped
}
/**
* @param value `(string: )` - Integration key for Duo.
*/
@JvmName("qqnbpwngmokwtcav")
public suspend fun integrationKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integrationKey = mapped
}
/**
* @param value `(string: )` - The mount to tie this method to for use in automatic mappings. The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*/
@JvmName("vgwoofnmolxnoyyo")
public suspend fun mountAccessor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mountAccessor = mapped
}
/**
* @param value `(string: )` – Name of the MFA method.
*/
@JvmName("xibqakuiisdxsere")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("uspmnartgtivmgwh")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value `(string)` - Push information for Duo.
*/
@JvmName("bihitbgkwoiynfcb")
public suspend fun pushInfo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pushInfo = mapped
}
/**
* @param value `(string: )` - Secret key for Duo.
*/
@JvmName("pkbefnuedyxqjojw")
public suspend fun secretKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretKey = mapped
}
/**
* @param value `(string)` - A format string for mapping Identity names to MFA method names. Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`. If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
@JvmName("cjlcpvcoepxsfona")
public suspend fun usernameFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameFormat = mapped
}
internal fun build(): MfaDuoArgs = MfaDuoArgs(
apiHostname = apiHostname,
integrationKey = integrationKey,
mountAccessor = mountAccessor,
name = name,
namespace = namespace,
pushInfo = pushInfo,
secretKey = secretKey,
usernameFormat = usernameFormat,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy