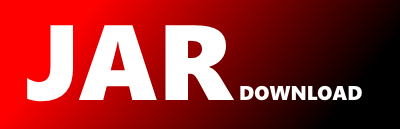
com.pulumi.vault.kotlin.MfaOktaArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.MfaOktaArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a resource to manage [Okta MFA](https://www.vaultproject.io/docs/enterprise/mfa/mfa-okta).
* **Note** this feature is available only with Vault Enterprise.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const userpass = new vault.AuthBackend("userpass", {
* type: "userpass",
* path: "userpass",
* });
* const myOkta = new vault.MfaOkta("my_okta", {
* name: "my_okta",
* mountAccessor: userpass.accessor,
* usernameFormat: "[email protected]",
* orgName: "hashicorp",
* apiToken: "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* userpass = vault.AuthBackend("userpass",
* type="userpass",
* path="userpass")
* my_okta = vault.MfaOkta("my_okta",
* name="my_okta",
* mount_accessor=userpass.accessor,
* username_format="[email protected]",
* org_name="hashicorp",
* api_token="eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var userpass = new Vault.AuthBackend("userpass", new()
* {
* Type = "userpass",
* Path = "userpass",
* });
* var myOkta = new Vault.MfaOkta("my_okta", new()
* {
* Name = "my_okta",
* MountAccessor = userpass.Accessor,
* UsernameFormat = "[email protected]",
* OrgName = "hashicorp",
* ApiToken = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* userpass, err := vault.NewAuthBackend(ctx, "userpass", &vault.AuthBackendArgs{
* Type: pulumi.String("userpass"),
* Path: pulumi.String("userpass"),
* })
* if err != nil {
* return err
* }
* _, err = vault.NewMfaOkta(ctx, "my_okta", &vault.MfaOktaArgs{
* Name: pulumi.String("my_okta"),
* MountAccessor: userpass.Accessor,
* UsernameFormat: pulumi.String("[email protected]"),
* OrgName: pulumi.String("hashicorp"),
* ApiToken: pulumi.String("eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.MfaOkta;
* import com.pulumi.vault.MfaOktaArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var userpass = new AuthBackend("userpass", AuthBackendArgs.builder()
* .type("userpass")
* .path("userpass")
* .build());
* var myOkta = new MfaOkta("myOkta", MfaOktaArgs.builder()
* .name("my_okta")
* .mountAccessor(userpass.accessor())
* .usernameFormat("[email protected]")
* .orgName("hashicorp")
* .apiToken("eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* userpass:
* type: vault:AuthBackend
* properties:
* type: userpass
* path: userpass
* myOkta:
* type: vault:MfaOkta
* name: my_okta
* properties:
* name: my_okta
* mountAccessor: ${userpass.accessor}
* usernameFormat: [email protected]
* orgName: hashicorp
* apiToken: eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9
* ```
*
* ## Import
* Mounts can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:index/mfaOkta:MfaOkta my_okta my_okta
* ```
* @property apiToken `(string: )` - Okta API key.
* @property baseUrl `(string)` - If set, will be used as the base domain for API requests. Examples are `okta.com`,
* `oktapreview.com`, and `okta-emea.com`.
* @property mountAccessor `(string: )` - The mount to tie this method to for use in automatic mappings.
* The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
* @property name `(string: )` – Name of the MFA method.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property orgName `(string: )` - Name of the organization to be used in the Okta API.
* @property primaryEmail `(string: )` - If set to true, the username will only match the
* primary email for the account.
* @property usernameFormat `(string)` - A format string for mapping Identity names to MFA method names.
* Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`.
* If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
public data class MfaOktaArgs(
public val apiToken: Output? = null,
public val baseUrl: Output? = null,
public val mountAccessor: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val orgName: Output? = null,
public val primaryEmail: Output? = null,
public val usernameFormat: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.MfaOktaArgs = com.pulumi.vault.MfaOktaArgs.builder()
.apiToken(apiToken?.applyValue({ args0 -> args0 }))
.baseUrl(baseUrl?.applyValue({ args0 -> args0 }))
.mountAccessor(mountAccessor?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.orgName(orgName?.applyValue({ args0 -> args0 }))
.primaryEmail(primaryEmail?.applyValue({ args0 -> args0 }))
.usernameFormat(usernameFormat?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MfaOktaArgs].
*/
@PulumiTagMarker
public class MfaOktaArgsBuilder internal constructor() {
private var apiToken: Output? = null
private var baseUrl: Output? = null
private var mountAccessor: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var orgName: Output? = null
private var primaryEmail: Output? = null
private var usernameFormat: Output? = null
/**
* @param value `(string: )` - Okta API key.
*/
@JvmName("gxxgjfbfilwiulao")
public suspend fun apiToken(`value`: Output) {
this.apiToken = value
}
/**
* @param value `(string)` - If set, will be used as the base domain for API requests. Examples are `okta.com`,
* `oktapreview.com`, and `okta-emea.com`.
*/
@JvmName("kqdcpkrmrfqtokky")
public suspend fun baseUrl(`value`: Output) {
this.baseUrl = value
}
/**
* @param value `(string: )` - The mount to tie this method to for use in automatic mappings.
* The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*/
@JvmName("avcgmkgeawmmxfto")
public suspend fun mountAccessor(`value`: Output) {
this.mountAccessor = value
}
/**
* @param value `(string: )` – Name of the MFA method.
*/
@JvmName("afpujiggjuojkedn")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("edineqmexyrcpsvj")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value `(string: )` - Name of the organization to be used in the Okta API.
*/
@JvmName("oelxaqchfnowdytd")
public suspend fun orgName(`value`: Output) {
this.orgName = value
}
/**
* @param value `(string: )` - If set to true, the username will only match the
* primary email for the account.
*/
@JvmName("lotobjntgbubfoxt")
public suspend fun primaryEmail(`value`: Output) {
this.primaryEmail = value
}
/**
* @param value `(string)` - A format string for mapping Identity names to MFA method names.
* Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`.
* If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
@JvmName("gpxcndpdcdjcpmsa")
public suspend fun usernameFormat(`value`: Output) {
this.usernameFormat = value
}
/**
* @param value `(string: )` - Okta API key.
*/
@JvmName("ssdkhiwfljcsfxwr")
public suspend fun apiToken(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiToken = mapped
}
/**
* @param value `(string)` - If set, will be used as the base domain for API requests. Examples are `okta.com`,
* `oktapreview.com`, and `okta-emea.com`.
*/
@JvmName("hfcmctenipbyjdfr")
public suspend fun baseUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseUrl = mapped
}
/**
* @param value `(string: )` - The mount to tie this method to for use in automatic mappings.
* The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*/
@JvmName("dlxiebjuewkwoopd")
public suspend fun mountAccessor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mountAccessor = mapped
}
/**
* @param value `(string: )` – Name of the MFA method.
*/
@JvmName("valklvkljudavyxu")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("ggsqgwfyvnccwwhe")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value `(string: )` - Name of the organization to be used in the Okta API.
*/
@JvmName("tataougctmosvuja")
public suspend fun orgName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.orgName = mapped
}
/**
* @param value `(string: )` - If set to true, the username will only match the
* primary email for the account.
*/
@JvmName("jxcchjycxtdnhosu")
public suspend fun primaryEmail(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryEmail = mapped
}
/**
* @param value `(string)` - A format string for mapping Identity names to MFA method names.
* Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`.
* If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
@JvmName("epidxpkiaahdgyaw")
public suspend fun usernameFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameFormat = mapped
}
internal fun build(): MfaOktaArgs = MfaOktaArgs(
apiToken = apiToken,
baseUrl = baseUrl,
mountAccessor = mountAccessor,
name = name,
namespace = namespace,
orgName = orgName,
primaryEmail = primaryEmail,
usernameFormat = usernameFormat,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy