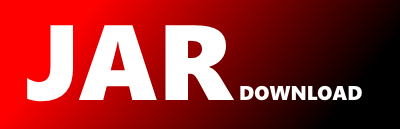
com.pulumi.vault.kotlin.MfaPingid.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [MfaPingid].
*/
@PulumiTagMarker
public class MfaPingidResourceBuilder internal constructor() {
public var name: String? = null
public var args: MfaPingidArgs = MfaPingidArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend MfaPingidArgsBuilder.() -> Unit) {
val builder = MfaPingidArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): MfaPingid {
val builtJavaResource = com.pulumi.vault.MfaPingid(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return MfaPingid(builtJavaResource)
}
}
/**
* Provides a resource to manage [PingID MFA](https://www.vaultproject.io/docs/enterprise/mfa/mfa-pingid).
* **Note** this feature is available only with Vault Enterprise.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const config = new pulumi.Config();
* const settingsFile = config.requireObject("settingsFile");
* const userpass = new vault.AuthBackend("userpass", {
* type: "userpass",
* path: "userpass",
* });
* const myPingid = new vault.MfaPingid("my_pingid", {
* name: "my_pingid",
* mountAccessor: userpass.accessor,
* usernameFormat: "[email protected]",
* settingsFileBase64: settingsFile,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* config = pulumi.Config()
* settings_file = config.require_object("settingsFile")
* userpass = vault.AuthBackend("userpass",
* type="userpass",
* path="userpass")
* my_pingid = vault.MfaPingid("my_pingid",
* name="my_pingid",
* mount_accessor=userpass.accessor,
* username_format="[email protected]",
* settings_file_base64=settings_file)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var config = new Config();
* var settingsFile = config.RequireObject("settingsFile");
* var userpass = new Vault.AuthBackend("userpass", new()
* {
* Type = "userpass",
* Path = "userpass",
* });
* var myPingid = new Vault.MfaPingid("my_pingid", new()
* {
* Name = "my_pingid",
* MountAccessor = userpass.Accessor,
* UsernameFormat = "[email protected]",
* SettingsFileBase64 = settingsFile,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi/config"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* cfg := config.New(ctx, "")
* settingsFile := cfg.RequireObject("settingsFile")
* userpass, err := vault.NewAuthBackend(ctx, "userpass", &vault.AuthBackendArgs{
* Type: pulumi.String("userpass"),
* Path: pulumi.String("userpass"),
* })
* if err != nil {
* return err
* }
* _, err = vault.NewMfaPingid(ctx, "my_pingid", &vault.MfaPingidArgs{
* Name: pulumi.String("my_pingid"),
* MountAccessor: userpass.Accessor,
* UsernameFormat: pulumi.String("[email protected]"),
* SettingsFileBase64: pulumi.Any(settingsFile),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.MfaPingid;
* import com.pulumi.vault.MfaPingidArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var settingsFile = config.get("settingsFile");
* var userpass = new AuthBackend("userpass", AuthBackendArgs.builder()
* .type("userpass")
* .path("userpass")
* .build());
* var myPingid = new MfaPingid("myPingid", MfaPingidArgs.builder()
* .name("my_pingid")
* .mountAccessor(userpass.accessor())
* .usernameFormat("[email protected]")
* .settingsFileBase64(settingsFile)
* .build());
* }
* }
* ```
* ```yaml
* configuration:
* settingsFile:
* type: dynamic
* resources:
* userpass:
* type: vault:AuthBackend
* properties:
* type: userpass
* path: userpass
* myPingid:
* type: vault:MfaPingid
* name: my_pingid
* properties:
* name: my_pingid
* mountAccessor: ${userpass.accessor}
* usernameFormat: [email protected]
* settingsFileBase64: ${settingsFile}
* ```
*
* ## Import
* Mounts can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:index/mfaPingid:MfaPingid my_pingid my_pingid
* ```
*/
public class MfaPingid internal constructor(
override val javaResource: com.pulumi.vault.MfaPingid,
) : KotlinCustomResource(javaResource, MfaPingidMapper) {
/**
* `(string)` – Admin URL computed by Vault
*/
public val adminUrl: Output
get() = javaResource.adminUrl().applyValue({ args0 -> args0 })
/**
* `(string)` – Authenticator URL computed by Vault
*/
public val authenticatorUrl: Output
get() = javaResource.authenticatorUrl().applyValue({ args0 -> args0 })
/**
* `(string)` – IDP URL computed by Vault
*/
public val idpUrl: Output
get() = javaResource.idpUrl().applyValue({ args0 -> args0 })
/**
* `(string: )` - The mount to tie this method to for use in automatic mappings.
* The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*/
public val mountAccessor: Output
get() = javaResource.mountAccessor().applyValue({ args0 -> args0 })
/**
* `(string: )` – Name of the MFA method.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* `(string)` – Namespace ID computed by Vault
*/
public val namespaceId: Output
get() = javaResource.namespaceId().applyValue({ args0 -> args0 })
/**
* `(string)` – Org Alias computed by Vault
*/
public val orgAlias: Output
get() = javaResource.orgAlias().applyValue({ args0 -> args0 })
/**
* `(string: )` - A base64-encoded third-party settings file retrieved
* from PingID's configuration page.
*/
public val settingsFileBase64: Output
get() = javaResource.settingsFileBase64().applyValue({ args0 -> args0 })
/**
* `(string)` – Type of configuration computed by Vault
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* `(string)` – If set to true, enables use of PingID signature. Computed by Vault
*/
public val useSignature: Output
get() = javaResource.useSignature().applyValue({ args0 -> args0 })
/**
* `(string)` - A format string for mapping Identity names to MFA method names.
* Values to substitute should be placed in `{{}}`. For example, `"{{alias.name}}@example.com"`.
* If blank, the Alias's Name field will be used as-is. Currently-supported mappings:
* - alias.name: The name returned by the mount configured via the `mount_accessor` parameter
* - entity.name: The name configured for the Entity
* - alias.metadata.``: The value of the Alias's metadata parameter
* - entity.metadata.``: The value of the Entity's metadata parameter
*/
public val usernameFormat: Output?
get() = javaResource.usernameFormat().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object MfaPingidMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.MfaPingid::class == javaResource::class
override fun map(javaResource: Resource): MfaPingid = MfaPingid(
javaResource as
com.pulumi.vault.MfaPingid,
)
}
/**
* @see [MfaPingid].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [MfaPingid].
*/
public suspend fun mfaPingid(name: String, block: suspend MfaPingidResourceBuilder.() -> Unit): MfaPingid {
val builder = MfaPingidResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [MfaPingid].
* @param name The _unique_ name of the resulting resource.
*/
public fun mfaPingid(name: String): MfaPingid {
val builder = MfaPingidResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy