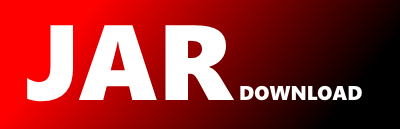
com.pulumi.vault.kotlin.NamespaceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.NamespaceArgs.builder
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* ## Import
* Namespaces can be imported using its `name` as accessor id
* ```sh
* $ pulumi import vault:index/namespace:Namespace example
* ```
* If the declared resource is imported and intends to support namespaces using a provider alias, then the name is relative to the namespace path.
* hcl
* provider "vault" {
* # Configuration options
* namespace = "example"
* alias = "example"
* }
* resource "vault_namespace" "example2" {
* provider = vault.example
* path = "example2"
* }
* ```sh
* $ pulumi import vault:index/namespace:Namespace example2 example2
* ```
* $ terraform state show vault_namespace.example2
* vault_namespace.example2:
* resource "vault_namespace" "example2" {
* id = "example/example2/"
* namespace_id =
* path = "example2"
* path_fq = "example2"
* }
* @property customMetadata Custom metadata describing this namespace. Value type
* is `map[string]string`. Requires Vault version 1.12+.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path The path of the namespace. Must not have a trailing `/`.
* @property pathFq The fully qualified path to the namespace. Useful when provisioning resources in a child `namespace`.
* The path is relative to the provider's `namespace` argument.
*/
public data class NamespaceArgs(
public val customMetadata: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy