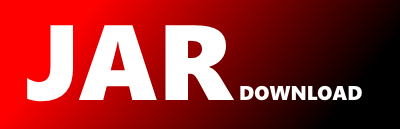
com.pulumi.vault.kotlin.NomadSecretBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.NomadSecretBackendArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const config = new vault.NomadSecretBackend("config", {
* backend: "nomad",
* description: "test description",
* defaultLeaseTtlSeconds: 3600,
* maxLeaseTtlSeconds: 7200,
* maxTtl: 240,
* address: "https://127.0.0.1:4646",
* token: "ae20ceaa-...",
* ttl: 120,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* config = vault.NomadSecretBackend("config",
* backend="nomad",
* description="test description",
* default_lease_ttl_seconds=3600,
* max_lease_ttl_seconds=7200,
* max_ttl=240,
* address="https://127.0.0.1:4646",
* token="ae20ceaa-...",
* ttl=120)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var config = new Vault.NomadSecretBackend("config", new()
* {
* Backend = "nomad",
* Description = "test description",
* DefaultLeaseTtlSeconds = 3600,
* MaxLeaseTtlSeconds = 7200,
* MaxTtl = 240,
* Address = "https://127.0.0.1:4646",
* Token = "ae20ceaa-...",
* Ttl = 120,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vault.NewNomadSecretBackend(ctx, "config", &vault.NomadSecretBackendArgs{
* Backend: pulumi.String("nomad"),
* Description: pulumi.String("test description"),
* DefaultLeaseTtlSeconds: pulumi.Int(3600),
* MaxLeaseTtlSeconds: pulumi.Int(7200),
* MaxTtl: pulumi.Int(240),
* Address: pulumi.String("https://127.0.0.1:4646"),
* Token: pulumi.String("ae20ceaa-..."),
* Ttl: pulumi.Int(120),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.NomadSecretBackend;
* import com.pulumi.vault.NomadSecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var config = new NomadSecretBackend("config", NomadSecretBackendArgs.builder()
* .backend("nomad")
* .description("test description")
* .defaultLeaseTtlSeconds("3600")
* .maxLeaseTtlSeconds("7200")
* .maxTtl("240")
* .address("https://127.0.0.1:4646")
* .token("ae20ceaa-...")
* .ttl("120")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* config:
* type: vault:NomadSecretBackend
* properties:
* backend: nomad
* description: test description
* defaultLeaseTtlSeconds: '3600'
* maxLeaseTtlSeconds: '7200'
* maxTtl: '240'
* address: https://127.0.0.1:4646
* token: ae20ceaa-...
* ttl: '120'
* ```
*
* ## Import
* Nomad secret backend can be imported using the `backend`, e.g.
* ```sh
* $ pulumi import vault:index/nomadSecretBackend:NomadSecretBackend nomad nomad
* ```
* @property address Specifies the address of the Nomad instance, provided
* as "protocol://host:port" like "http://127.0.0.1:4646".
* @property backend The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `nomad`.
* @property caCert CA certificate to use when verifying the Nomad server certificate, must be
* x509 PEM encoded.
* @property clientCert Client certificate to provide to the Nomad server, must be x509 PEM encoded.
* @property clientKey Client certificate key to provide to the Nomad server, must be x509 PEM encoded.
* @property defaultLeaseTtlSeconds Default lease duration for secrets in seconds.
* @property description Human-friendly description of the mount for the Active Directory backend.
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property local Mark the secrets engine as local-only. Local engines are not replicated or removed by
* replication.Tolerance duration to use when checking the last rotation time.
* @property maxLeaseTtlSeconds Maximum possible lease duration for secrets in seconds.
* @property maxTokenNameLength Specifies the maximum length to use for the name of the Nomad token
* generated with Generate Credential. If omitted, 0 is used and ignored, defaulting to the max value allowed
* by the Nomad version.
* @property maxTtl Maximum possible lease duration for secrets in seconds.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property token Specifies the Nomad Management token to use.
* @property ttl Specifies the ttl of the lease for the generated token.
*/
public data class NomadSecretBackendArgs(
public val address: Output? = null,
public val backend: Output? = null,
public val caCert: Output? = null,
public val clientCert: Output? = null,
public val clientKey: Output? = null,
public val defaultLeaseTtlSeconds: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val local: Output? = null,
public val maxLeaseTtlSeconds: Output? = null,
public val maxTokenNameLength: Output? = null,
public val maxTtl: Output? = null,
public val namespace: Output? = null,
public val token: Output? = null,
public val ttl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.NomadSecretBackendArgs =
com.pulumi.vault.NomadSecretBackendArgs.builder()
.address(address?.applyValue({ args0 -> args0 }))
.backend(backend?.applyValue({ args0 -> args0 }))
.caCert(caCert?.applyValue({ args0 -> args0 }))
.clientCert(clientCert?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.defaultLeaseTtlSeconds(defaultLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.local(local?.applyValue({ args0 -> args0 }))
.maxLeaseTtlSeconds(maxLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.maxTokenNameLength(maxTokenNameLength?.applyValue({ args0 -> args0 }))
.maxTtl(maxTtl?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.token(token?.applyValue({ args0 -> args0 }))
.ttl(ttl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NomadSecretBackendArgs].
*/
@PulumiTagMarker
public class NomadSecretBackendArgsBuilder internal constructor() {
private var address: Output? = null
private var backend: Output? = null
private var caCert: Output? = null
private var clientCert: Output? = null
private var clientKey: Output? = null
private var defaultLeaseTtlSeconds: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var local: Output? = null
private var maxLeaseTtlSeconds: Output? = null
private var maxTokenNameLength: Output? = null
private var maxTtl: Output? = null
private var namespace: Output? = null
private var token: Output? = null
private var ttl: Output? = null
/**
* @param value Specifies the address of the Nomad instance, provided
* as "protocol://host:port" like "http://127.0.0.1:4646".
*/
@JvmName("lrofciftsvufkacu")
public suspend fun address(`value`: Output) {
this.address = value
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `nomad`.
*/
@JvmName("vqwjvsvvprvtlcsh")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value CA certificate to use when verifying the Nomad server certificate, must be
* x509 PEM encoded.
*/
@JvmName("vdehvnrbwfcnffqq")
public suspend fun caCert(`value`: Output) {
this.caCert = value
}
/**
* @param value Client certificate to provide to the Nomad server, must be x509 PEM encoded.
*/
@JvmName("fdshorcknqyiyfum")
public suspend fun clientCert(`value`: Output) {
this.clientCert = value
}
/**
* @param value Client certificate key to provide to the Nomad server, must be x509 PEM encoded.
*/
@JvmName("ekttnvygialpnnid")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value Default lease duration for secrets in seconds.
*/
@JvmName("hggraibiuifbgwlj")
public suspend fun defaultLeaseTtlSeconds(`value`: Output) {
this.defaultLeaseTtlSeconds = value
}
/**
* @param value Human-friendly description of the mount for the Active Directory backend.
*/
@JvmName("ldwewhnbbtcqhtqb")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("sclqtexxjesghkpo")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value Mark the secrets engine as local-only. Local engines are not replicated or removed by
* replication.Tolerance duration to use when checking the last rotation time.
*/
@JvmName("skmgrbhtgtlvjned")
public suspend fun local(`value`: Output) {
this.local = value
}
/**
* @param value Maximum possible lease duration for secrets in seconds.
*/
@JvmName("cmfblhaswgfsrmmb")
public suspend fun maxLeaseTtlSeconds(`value`: Output) {
this.maxLeaseTtlSeconds = value
}
/**
* @param value Specifies the maximum length to use for the name of the Nomad token
* generated with Generate Credential. If omitted, 0 is used and ignored, defaulting to the max value allowed
* by the Nomad version.
*/
@JvmName("tsyhsbjhkexovgal")
public suspend fun maxTokenNameLength(`value`: Output) {
this.maxTokenNameLength = value
}
/**
* @param value Maximum possible lease duration for secrets in seconds.
*/
@JvmName("pevijdhgjyoyhhvb")
public suspend fun maxTtl(`value`: Output) {
this.maxTtl = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("qrkshljqggfhklld")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Specifies the Nomad Management token to use.
*/
@JvmName("racaxxbvopfuxngx")
public suspend fun token(`value`: Output) {
this.token = value
}
/**
* @param value Specifies the ttl of the lease for the generated token.
*/
@JvmName("uutjkwamhiuruown")
public suspend fun ttl(`value`: Output) {
this.ttl = value
}
/**
* @param value Specifies the address of the Nomad instance, provided
* as "protocol://host:port" like "http://127.0.0.1:4646".
*/
@JvmName("iquaaormerahqaom")
public suspend fun address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address = mapped
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `nomad`.
*/
@JvmName("otxijdeqxefhiubi")
public suspend fun backend(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backend = mapped
}
/**
* @param value CA certificate to use when verifying the Nomad server certificate, must be
* x509 PEM encoded.
*/
@JvmName("xuwbbkauldjkstmo")
public suspend fun caCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCert = mapped
}
/**
* @param value Client certificate to provide to the Nomad server, must be x509 PEM encoded.
*/
@JvmName("xqiwwqnxpkflqeam")
public suspend fun clientCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCert = mapped
}
/**
* @param value Client certificate key to provide to the Nomad server, must be x509 PEM encoded.
*/
@JvmName("sgirwswwcwwnqybh")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value Default lease duration for secrets in seconds.
*/
@JvmName("gytcpltolpdnjleb")
public suspend fun defaultLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultLeaseTtlSeconds = mapped
}
/**
* @param value Human-friendly description of the mount for the Active Directory backend.
*/
@JvmName("vamopjkneeqgfyyw")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("ofbxcfqlvoeugyhx")
public suspend fun disableRemount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableRemount = mapped
}
/**
* @param value Mark the secrets engine as local-only. Local engines are not replicated or removed by
* replication.Tolerance duration to use when checking the last rotation time.
*/
@JvmName("khekoaigcsgxrxro")
public suspend fun local(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.local = mapped
}
/**
* @param value Maximum possible lease duration for secrets in seconds.
*/
@JvmName("myrfgqdgtojmvupe")
public suspend fun maxLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxLeaseTtlSeconds = mapped
}
/**
* @param value Specifies the maximum length to use for the name of the Nomad token
* generated with Generate Credential. If omitted, 0 is used and ignored, defaulting to the max value allowed
* by the Nomad version.
*/
@JvmName("qcxrxbbqtotvvnop")
public suspend fun maxTokenNameLength(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxTokenNameLength = mapped
}
/**
* @param value Maximum possible lease duration for secrets in seconds.
*/
@JvmName("vwoxgohebankfjij")
public suspend fun maxTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxTtl = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("qaktnlmnojxgpfsi")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Specifies the Nomad Management token to use.
*/
@JvmName("jmfhdthgttjqewwd")
public suspend fun token(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.token = mapped
}
/**
* @param value Specifies the ttl of the lease for the generated token.
*/
@JvmName("hngrwujgsrpnobpy")
public suspend fun ttl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ttl = mapped
}
internal fun build(): NomadSecretBackendArgs = NomadSecretBackendArgs(
address = address,
backend = backend,
caCert = caCert,
clientCert = clientCert,
clientKey = clientKey,
defaultLeaseTtlSeconds = defaultLeaseTtlSeconds,
description = description,
disableRemount = disableRemount,
local = local,
maxLeaseTtlSeconds = maxLeaseTtlSeconds,
maxTokenNameLength = maxTokenNameLength,
maxTtl = maxTtl,
namespace = namespace,
token = token,
ttl = ttl,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy