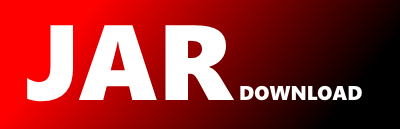
com.pulumi.vault.kotlin.ProviderArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.ProviderArgs.builder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginAwsArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginAwsArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginAzureArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginAzureArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginCertArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginCertArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginGcpArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginGcpArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginJwtArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginJwtArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginKerberosArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginKerberosArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginOciArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginOciArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginOidcArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginOidcArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginRadiusArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginRadiusArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginTokenFileArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginTokenFileArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginUserpassArgs
import com.pulumi.vault.kotlin.inputs.ProviderAuthLoginUserpassArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderClientAuthArgs
import com.pulumi.vault.kotlin.inputs.ProviderClientAuthArgsBuilder
import com.pulumi.vault.kotlin.inputs.ProviderHeaderArgs
import com.pulumi.vault.kotlin.inputs.ProviderHeaderArgsBuilder
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The provider type for the vault package. By default, resources use package-wide configuration
* settings, however an explicit `Provider` instance may be created and passed during resource
* construction to achieve fine-grained programmatic control over provider settings. See the
* [documentation](https://www.pulumi.com/docs/reference/programming-model/#providers) for more information.
* @property addAddressToEnv
* @property address URL of the root of the target Vault server.
* @property authLogin Login to vault with an existing auth method using auth//login
* @property authLoginAws Login to vault using the AWS method
* @property authLoginAzure Login to vault using the azure method
* @property authLoginCert Login to vault using the cert method
* @property authLoginGcp Login to vault using the gcp method
* @property authLoginJwt Login to vault using the jwt method
* @property authLoginKerberos Login to vault using the kerberos method
* @property authLoginOci Login to vault using the OCI method
* @property authLoginOidc Login to vault using the oidc method
* @property authLoginRadius Login to vault using the radius method
* @property authLoginTokenFile Login to vault using
* @property authLoginUserpass Login to vault using the userpass method
* @property caCertDir Path to directory containing CA certificate files to validate the server's certificate.
* @property caCertFile Path to a CA certificate file to validate the server's certificate.
* @property clientAuth Client authentication credentials.
* @property headers The headers to send with each Vault request.
* @property maxLeaseTtlSeconds Maximum TTL for secret leases requested by this provider.
* @property maxRetries Maximum number of retries when a 5xx error code is encountered.
* @property maxRetriesCcc Maximum number of retries for Client Controlled Consistency related operations
* @property namespace The namespace to use. Available only for Vault Enterprise.
* @property setNamespaceFromToken In the case where the Vault token is for a specific namespace and the provider namespace is not configured, use the
* token namespace as the root namespace for all resources.
* @property skipChildToken Set this to true to prevent the creation of ephemeral child token used by this provider.
* @property skipGetVaultVersion Skip the dynamic fetching of the Vault server version.
* @property skipTlsVerify Set this to true only if the target Vault server is an insecure development instance.
* @property tlsServerName Name to use as the SNI host when connecting via TLS.
* @property token Token to use to authenticate to Vault.
* @property tokenName Token name to use for creating the Vault child token.
* @property vaultVersionOverride Override the Vault server version, which is normally determined dynamically from the target Vault server
*/
public data class ProviderArgs(
public val addAddressToEnv: Output? = null,
public val address: Output? = null,
public val authLogin: Output? = null,
public val authLoginAws: Output? = null,
public val authLoginAzure: Output? = null,
public val authLoginCert: Output? = null,
public val authLoginGcp: Output? = null,
public val authLoginJwt: Output? = null,
public val authLoginKerberos: Output? = null,
public val authLoginOci: Output? = null,
public val authLoginOidc: Output? = null,
public val authLoginRadius: Output? = null,
public val authLoginTokenFile: Output? = null,
public val authLoginUserpass: Output? = null,
public val caCertDir: Output? = null,
public val caCertFile: Output? = null,
@Deprecated(
message = """
Use auth_login_cert instead
""",
)
public val clientAuth: Output? = null,
public val headers: Output>? = null,
public val maxLeaseTtlSeconds: Output? = null,
public val maxRetries: Output? = null,
public val maxRetriesCcc: Output? = null,
public val namespace: Output? = null,
public val setNamespaceFromToken: Output? = null,
public val skipChildToken: Output? = null,
public val skipGetVaultVersion: Output? = null,
public val skipTlsVerify: Output? = null,
public val tlsServerName: Output? = null,
public val token: Output? = null,
public val tokenName: Output? = null,
public val vaultVersionOverride: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.ProviderArgs = com.pulumi.vault.ProviderArgs.builder()
.addAddressToEnv(addAddressToEnv?.applyValue({ args0 -> args0 }))
.address(address?.applyValue({ args0 -> args0 }))
.authLogin(authLogin?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginAws(authLoginAws?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginAzure(authLoginAzure?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginCert(authLoginCert?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginGcp(authLoginGcp?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginJwt(authLoginJwt?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginKerberos(authLoginKerberos?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginOci(authLoginOci?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginOidc(authLoginOidc?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginRadius(authLoginRadius?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authLoginTokenFile(
authLoginTokenFile?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.authLoginUserpass(authLoginUserpass?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.caCertDir(caCertDir?.applyValue({ args0 -> args0 }))
.caCertFile(caCertFile?.applyValue({ args0 -> args0 }))
.clientAuth(clientAuth?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.headers(
headers?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.maxLeaseTtlSeconds(maxLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.maxRetries(maxRetries?.applyValue({ args0 -> args0 }))
.maxRetriesCcc(maxRetriesCcc?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.setNamespaceFromToken(setNamespaceFromToken?.applyValue({ args0 -> args0 }))
.skipChildToken(skipChildToken?.applyValue({ args0 -> args0 }))
.skipGetVaultVersion(skipGetVaultVersion?.applyValue({ args0 -> args0 }))
.skipTlsVerify(skipTlsVerify?.applyValue({ args0 -> args0 }))
.tlsServerName(tlsServerName?.applyValue({ args0 -> args0 }))
.token(token?.applyValue({ args0 -> args0 }))
.tokenName(tokenName?.applyValue({ args0 -> args0 }))
.vaultVersionOverride(vaultVersionOverride?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProviderArgs].
*/
@PulumiTagMarker
public class ProviderArgsBuilder internal constructor() {
private var addAddressToEnv: Output? = null
private var address: Output? = null
private var authLogin: Output? = null
private var authLoginAws: Output? = null
private var authLoginAzure: Output? = null
private var authLoginCert: Output? = null
private var authLoginGcp: Output? = null
private var authLoginJwt: Output? = null
private var authLoginKerberos: Output? = null
private var authLoginOci: Output? = null
private var authLoginOidc: Output? = null
private var authLoginRadius: Output? = null
private var authLoginTokenFile: Output? = null
private var authLoginUserpass: Output? = null
private var caCertDir: Output? = null
private var caCertFile: Output? = null
private var clientAuth: Output? = null
private var headers: Output>? = null
private var maxLeaseTtlSeconds: Output? = null
private var maxRetries: Output? = null
private var maxRetriesCcc: Output? = null
private var namespace: Output? = null
private var setNamespaceFromToken: Output? = null
private var skipChildToken: Output? = null
private var skipGetVaultVersion: Output? = null
private var skipTlsVerify: Output? = null
private var tlsServerName: Output? = null
private var token: Output? = null
private var tokenName: Output? = null
private var vaultVersionOverride: Output? = null
/**
* @param value
*/
@JvmName("vqmhaxqcuuwkmwxx")
public suspend fun addAddressToEnv(`value`: Output) {
this.addAddressToEnv = value
}
/**
* @param value URL of the root of the target Vault server.
*/
@JvmName("ubvqsapplsnblitj")
public suspend fun address(`value`: Output) {
this.address = value
}
/**
* @param value Login to vault with an existing auth method using auth//login
*/
@JvmName("ckyqppqbggrvvnha")
public suspend fun authLogin(`value`: Output) {
this.authLogin = value
}
/**
* @param value Login to vault using the AWS method
*/
@JvmName("rwbcnlpooxsphvcl")
public suspend fun authLoginAws(`value`: Output) {
this.authLoginAws = value
}
/**
* @param value Login to vault using the azure method
*/
@JvmName("hrqvlmuwkmaltuwy")
public suspend fun authLoginAzure(`value`: Output) {
this.authLoginAzure = value
}
/**
* @param value Login to vault using the cert method
*/
@JvmName("slyunixturptlrxs")
public suspend fun authLoginCert(`value`: Output) {
this.authLoginCert = value
}
/**
* @param value Login to vault using the gcp method
*/
@JvmName("yxiccxshwjvjuohr")
public suspend fun authLoginGcp(`value`: Output) {
this.authLoginGcp = value
}
/**
* @param value Login to vault using the jwt method
*/
@JvmName("jqkoatbmoilefchu")
public suspend fun authLoginJwt(`value`: Output) {
this.authLoginJwt = value
}
/**
* @param value Login to vault using the kerberos method
*/
@JvmName("puoekwonsbidvcpc")
public suspend fun authLoginKerberos(`value`: Output) {
this.authLoginKerberos = value
}
/**
* @param value Login to vault using the OCI method
*/
@JvmName("vvixrceqjgltosrp")
public suspend fun authLoginOci(`value`: Output) {
this.authLoginOci = value
}
/**
* @param value Login to vault using the oidc method
*/
@JvmName("luajhcwefxblodfw")
public suspend fun authLoginOidc(`value`: Output) {
this.authLoginOidc = value
}
/**
* @param value Login to vault using the radius method
*/
@JvmName("jnkqftjxfjcyiuuk")
public suspend fun authLoginRadius(`value`: Output) {
this.authLoginRadius = value
}
/**
* @param value Login to vault using
*/
@JvmName("iepmoqgyqwyekwhr")
public suspend fun authLoginTokenFile(`value`: Output) {
this.authLoginTokenFile = value
}
/**
* @param value Login to vault using the userpass method
*/
@JvmName("rsxdxhsnakmogyry")
public suspend fun authLoginUserpass(`value`: Output) {
this.authLoginUserpass = value
}
/**
* @param value Path to directory containing CA certificate files to validate the server's certificate.
*/
@JvmName("pascvcamjoajlxkg")
public suspend fun caCertDir(`value`: Output) {
this.caCertDir = value
}
/**
* @param value Path to a CA certificate file to validate the server's certificate.
*/
@JvmName("ngcfcnqthfbidfwc")
public suspend fun caCertFile(`value`: Output) {
this.caCertFile = value
}
/**
* @param value Client authentication credentials.
*/
@Deprecated(
message = """
Use auth_login_cert instead
""",
)
@JvmName("efcmsnxlpxobchbf")
public suspend fun clientAuth(`value`: Output) {
this.clientAuth = value
}
/**
* @param value The headers to send with each Vault request.
*/
@JvmName("sxcfuasptojelcuh")
public suspend fun headers(`value`: Output>) {
this.headers = value
}
@JvmName("sfetwgxivpjrfigj")
public suspend fun headers(vararg values: Output) {
this.headers = Output.all(values.asList())
}
/**
* @param values The headers to send with each Vault request.
*/
@JvmName("frodifodjqcgsugj")
public suspend fun headers(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy