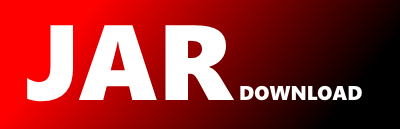
com.pulumi.vault.kotlin.QuotaLeaseCount.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [QuotaLeaseCount].
*/
@PulumiTagMarker
public class QuotaLeaseCountResourceBuilder internal constructor() {
public var name: String? = null
public var args: QuotaLeaseCountArgs = QuotaLeaseCountArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend QuotaLeaseCountArgsBuilder.() -> Unit) {
val builder = QuotaLeaseCountArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): QuotaLeaseCount {
val builtJavaResource = com.pulumi.vault.QuotaLeaseCount(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return QuotaLeaseCount(builtJavaResource)
}
}
/**
* Manage lease count quotas which enforce the number of leases that can be created.
* A lease count quota can be created at the root level or defined on a namespace or mount by
* specifying a path when creating the quota.
* See [Vault's Documentation](https://www.vaultproject.io/docs/enterprise/lease-count-quotas) for more
* information.
* **Note** this feature is available only with Vault Enterprise.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const global = new vault.QuotaLeaseCount("global", {
* name: "global",
* path: "",
* maxLeases: 100,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* global_ = vault.QuotaLeaseCount("global",
* name="global",
* path="",
* max_leases=100)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var @global = new Vault.QuotaLeaseCount("global", new()
* {
* Name = "global",
* Path = "",
* MaxLeases = 100,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vault.NewQuotaLeaseCount(ctx, "global", &vault.QuotaLeaseCountArgs{
* Name: pulumi.String("global"),
* Path: pulumi.String(""),
* MaxLeases: pulumi.Int(100),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.QuotaLeaseCount;
* import com.pulumi.vault.QuotaLeaseCountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var global = new QuotaLeaseCount("global", QuotaLeaseCountArgs.builder()
* .name("global")
* .path("")
* .maxLeases(100)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* global:
* type: vault:QuotaLeaseCount
* properties:
* name: global
* path:
* maxLeases: 100
* ```
*
* ## Import
* Lease count quotas can be imported using their names
* ```sh
* $ pulumi import vault:index/quotaLeaseCount:QuotaLeaseCount global global
* ```
*/
public class QuotaLeaseCount internal constructor(
override val javaResource: com.pulumi.vault.QuotaLeaseCount,
) : KotlinCustomResource(javaResource, QuotaLeaseCountMapper) {
/**
* If set to `true` on a quota where path is set to a namespace, the same quota will be cumulatively applied to all child namespace. The inheritable parameter cannot be set to `true` if the path does not specify a namespace. Only the quotas associated with the root namespace are inheritable by default. Requires Vault 1.15+.
*/
public val inheritable: Output?
get() = javaResource.inheritable().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum number of leases to be allowed by the quota
* rule. The `max_leases` must be positive.
*/
public val maxLeases: Output
get() = javaResource.maxLeases().applyValue({ args0 -> args0 })
/**
* Name of the rate limit quota
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured namespace.
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Path of the mount or namespace to apply the quota. A blank path configures a
* global rate limit quota. For example `namespace1/` adds a quota to a full namespace,
* `namespace1/auth/userpass` adds a `quota` to `userpass` in `namespace1`.
* Updating this field on an existing quota can have "moving" effects. For example, updating
* `auth/userpass` to `namespace1/auth/userpass` moves this quota from being a global mount quota to
* a namespace specific mount quota. **Note, namespaces are supported in Enterprise only.**
*/
public val path: Output?
get() = javaResource.path().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* If set on a quota where `path` is set to an auth mount with a concept of roles (such as /auth/approle/), this will make the quota restrict login requests to that mount that are made with the specified role.
*/
public val role: Output?
get() = javaResource.role().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object QuotaLeaseCountMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.QuotaLeaseCount::class == javaResource::class
override fun map(javaResource: Resource): QuotaLeaseCount = QuotaLeaseCount(
javaResource as
com.pulumi.vault.QuotaLeaseCount,
)
}
/**
* @see [QuotaLeaseCount].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [QuotaLeaseCount].
*/
public suspend fun quotaLeaseCount(
name: String,
block: suspend QuotaLeaseCountResourceBuilder.() -> Unit,
): QuotaLeaseCount {
val builder = QuotaLeaseCountResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [QuotaLeaseCount].
* @param name The _unique_ name of the resulting resource.
*/
public fun quotaLeaseCount(name: String): QuotaLeaseCount {
val builder = QuotaLeaseCountResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy