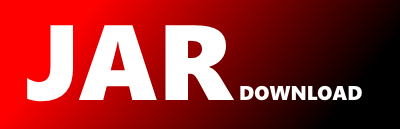
com.pulumi.vault.kotlin.RaftAutopilotArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.RaftAutopilotArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Autopilot enables automated workflows for managing Raft clusters. The
* current feature set includes 3 main features: Server Stabilization, Dead
* Server Cleanup and State API. **These three features are introduced in
* Vault 1.7.**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const autopilot = new vault.RaftAutopilot("autopilot", {
* cleanupDeadServers: true,
* deadServerLastContactThreshold: "24h0m0s",
* lastContactThreshold: "10s",
* maxTrailingLogs: 1000,
* minQuorum: 3,
* serverStabilizationTime: "10s",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* autopilot = vault.RaftAutopilot("autopilot",
* cleanup_dead_servers=True,
* dead_server_last_contact_threshold="24h0m0s",
* last_contact_threshold="10s",
* max_trailing_logs=1000,
* min_quorum=3,
* server_stabilization_time="10s")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var autopilot = new Vault.RaftAutopilot("autopilot", new()
* {
* CleanupDeadServers = true,
* DeadServerLastContactThreshold = "24h0m0s",
* LastContactThreshold = "10s",
* MaxTrailingLogs = 1000,
* MinQuorum = 3,
* ServerStabilizationTime = "10s",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vault.NewRaftAutopilot(ctx, "autopilot", &vault.RaftAutopilotArgs{
* CleanupDeadServers: pulumi.Bool(true),
* DeadServerLastContactThreshold: pulumi.String("24h0m0s"),
* LastContactThreshold: pulumi.String("10s"),
* MaxTrailingLogs: pulumi.Int(1000),
* MinQuorum: pulumi.Int(3),
* ServerStabilizationTime: pulumi.String("10s"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.RaftAutopilot;
* import com.pulumi.vault.RaftAutopilotArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var autopilot = new RaftAutopilot("autopilot", RaftAutopilotArgs.builder()
* .cleanupDeadServers(true)
* .deadServerLastContactThreshold("24h0m0s")
* .lastContactThreshold("10s")
* .maxTrailingLogs(1000)
* .minQuorum(3)
* .serverStabilizationTime("10s")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* autopilot:
* type: vault:RaftAutopilot
* properties:
* cleanupDeadServers: true
* deadServerLastContactThreshold: 24h0m0s
* lastContactThreshold: 10s
* maxTrailingLogs: 1000
* minQuorum: 3
* serverStabilizationTime: 10s
* ```
*
* ## Import
* Raft Autopilot config can be imported using the ID, e.g.
* ```sh
* $ pulumi import vault:index/raftAutopilot:RaftAutopilot autopilot sys/storage/raft/autopilot/configuration
* ```
* @property cleanupDeadServers Specifies whether to remove dead server nodes
* periodically or when a new server joins. This requires that `min-quorum` is also set.
* @property deadServerLastContactThreshold Limit the amount of time a
* server can go without leader contact before being considered failed. This only takes
* effect when `cleanup_dead_servers` is set.
* @property disableUpgradeMigration Disables automatically upgrading Vault using autopilot. (Enterprise-only)
* @property lastContactThreshold Limit the amount of time a server can go
* without leader contact before being considered unhealthy.
* @property maxTrailingLogs Maximum number of log entries in the Raft log
* that a server can be behind its leader before being considered unhealthy.
* @property minQuorum Minimum number of servers allowed in a cluster before
* autopilot can prune dead servers. This should at least be 3. Applicable only for
* voting nodes.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property serverStabilizationTime Minimum amount of time a server must be
* stable in the 'healthy' state before being added to the cluster.
*/
public data class RaftAutopilotArgs(
public val cleanupDeadServers: Output? = null,
public val deadServerLastContactThreshold: Output? = null,
public val disableUpgradeMigration: Output? = null,
public val lastContactThreshold: Output? = null,
public val maxTrailingLogs: Output? = null,
public val minQuorum: Output? = null,
public val namespace: Output? = null,
public val serverStabilizationTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.RaftAutopilotArgs =
com.pulumi.vault.RaftAutopilotArgs.builder()
.cleanupDeadServers(cleanupDeadServers?.applyValue({ args0 -> args0 }))
.deadServerLastContactThreshold(deadServerLastContactThreshold?.applyValue({ args0 -> args0 }))
.disableUpgradeMigration(disableUpgradeMigration?.applyValue({ args0 -> args0 }))
.lastContactThreshold(lastContactThreshold?.applyValue({ args0 -> args0 }))
.maxTrailingLogs(maxTrailingLogs?.applyValue({ args0 -> args0 }))
.minQuorum(minQuorum?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.serverStabilizationTime(serverStabilizationTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RaftAutopilotArgs].
*/
@PulumiTagMarker
public class RaftAutopilotArgsBuilder internal constructor() {
private var cleanupDeadServers: Output? = null
private var deadServerLastContactThreshold: Output? = null
private var disableUpgradeMigration: Output? = null
private var lastContactThreshold: Output? = null
private var maxTrailingLogs: Output? = null
private var minQuorum: Output? = null
private var namespace: Output? = null
private var serverStabilizationTime: Output? = null
/**
* @param value Specifies whether to remove dead server nodes
* periodically or when a new server joins. This requires that `min-quorum` is also set.
*/
@JvmName("bfrajgqogbsbfylc")
public suspend fun cleanupDeadServers(`value`: Output) {
this.cleanupDeadServers = value
}
/**
* @param value Limit the amount of time a
* server can go without leader contact before being considered failed. This only takes
* effect when `cleanup_dead_servers` is set.
*/
@JvmName("yjywqcwtprjtkrvo")
public suspend fun deadServerLastContactThreshold(`value`: Output) {
this.deadServerLastContactThreshold = value
}
/**
* @param value Disables automatically upgrading Vault using autopilot. (Enterprise-only)
*/
@JvmName("eurpblfbotcedswo")
public suspend fun disableUpgradeMigration(`value`: Output) {
this.disableUpgradeMigration = value
}
/**
* @param value Limit the amount of time a server can go
* without leader contact before being considered unhealthy.
*/
@JvmName("wquxlcxijopgnump")
public suspend fun lastContactThreshold(`value`: Output) {
this.lastContactThreshold = value
}
/**
* @param value Maximum number of log entries in the Raft log
* that a server can be behind its leader before being considered unhealthy.
*/
@JvmName("hsonhqavpdcvovtp")
public suspend fun maxTrailingLogs(`value`: Output) {
this.maxTrailingLogs = value
}
/**
* @param value Minimum number of servers allowed in a cluster before
* autopilot can prune dead servers. This should at least be 3. Applicable only for
* voting nodes.
*/
@JvmName("dfawbrrfvfxflvvp")
public suspend fun minQuorum(`value`: Output) {
this.minQuorum = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("ylnufopxoglkrxii")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Minimum amount of time a server must be
* stable in the 'healthy' state before being added to the cluster.
*/
@JvmName("lixivpihxiusstsp")
public suspend fun serverStabilizationTime(`value`: Output) {
this.serverStabilizationTime = value
}
/**
* @param value Specifies whether to remove dead server nodes
* periodically or when a new server joins. This requires that `min-quorum` is also set.
*/
@JvmName("dltlhxmuekwylgvc")
public suspend fun cleanupDeadServers(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cleanupDeadServers = mapped
}
/**
* @param value Limit the amount of time a
* server can go without leader contact before being considered failed. This only takes
* effect when `cleanup_dead_servers` is set.
*/
@JvmName("lqbcphbuombcrpmq")
public suspend fun deadServerLastContactThreshold(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadServerLastContactThreshold = mapped
}
/**
* @param value Disables automatically upgrading Vault using autopilot. (Enterprise-only)
*/
@JvmName("dfrkjtbyofahpnft")
public suspend fun disableUpgradeMigration(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableUpgradeMigration = mapped
}
/**
* @param value Limit the amount of time a server can go
* without leader contact before being considered unhealthy.
*/
@JvmName("rcctuoqrrwllgnne")
public suspend fun lastContactThreshold(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastContactThreshold = mapped
}
/**
* @param value Maximum number of log entries in the Raft log
* that a server can be behind its leader before being considered unhealthy.
*/
@JvmName("cupseqxipchrinsw")
public suspend fun maxTrailingLogs(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxTrailingLogs = mapped
}
/**
* @param value Minimum number of servers allowed in a cluster before
* autopilot can prune dead servers. This should at least be 3. Applicable only for
* voting nodes.
*/
@JvmName("ugvcefgomypyajmx")
public suspend fun minQuorum(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minQuorum = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("eclsbveojswncbpw")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Minimum amount of time a server must be
* stable in the 'healthy' state before being added to the cluster.
*/
@JvmName("giyhjgxjfbwlciyi")
public suspend fun serverStabilizationTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serverStabilizationTime = mapped
}
internal fun build(): RaftAutopilotArgs = RaftAutopilotArgs(
cleanupDeadServers = cleanupDeadServers,
deadServerLastContactThreshold = deadServerLastContactThreshold,
disableUpgradeMigration = disableUpgradeMigration,
lastContactThreshold = lastContactThreshold,
maxTrailingLogs = maxTrailingLogs,
minQuorum = minQuorum,
namespace = namespace,
serverStabilizationTime = serverStabilizationTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy