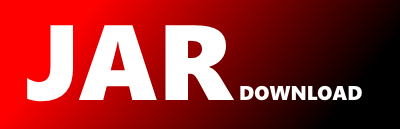
com.pulumi.vault.kotlin.inputs.GetPolicyDocumentRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kotlin.inputs
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.inputs.GetPolicyDocumentRule.builder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property allowedParameters Whitelists a list of keys and values that are permitted on the given path. See Parameters below.
* @property capabilities A list of capabilities that this rule apply to `path`. For example, ["read", "write"].
* @property deniedParameters Blacklists a list of parameter and values. Any values specified here take precedence over `allowed_parameter`. See Parameters below.
* @property description Description of the rule. Will be added as a comment to rendered rule.
* @property maxWrappingTtl The maximum allowed TTL that clients can specify for a wrapped response.
* @property minWrappingTtl The minimum allowed TTL that clients can specify for a wrapped response.
* @property path A path in Vault that this rule applies to.
* @property requiredParameters A list of parameters that must be specified.
*/
public data class GetPolicyDocumentRule(
public val allowedParameters: List? = null,
public val capabilities: List,
public val deniedParameters: List? = null,
public val description: String? = null,
public val maxWrappingTtl: String? = null,
public val minWrappingTtl: String? = null,
public val path: String,
public val requiredParameters: List? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.inputs.GetPolicyDocumentRule =
com.pulumi.vault.inputs.GetPolicyDocumentRule.builder()
.allowedParameters(
allowedParameters?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.capabilities(capabilities.let({ args0 -> args0.map({ args0 -> args0 }) }))
.deniedParameters(
deniedParameters?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.description(description?.let({ args0 -> args0 }))
.maxWrappingTtl(maxWrappingTtl?.let({ args0 -> args0 }))
.minWrappingTtl(minWrappingTtl?.let({ args0 -> args0 }))
.path(path.let({ args0 -> args0 }))
.requiredParameters(requiredParameters?.let({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [GetPolicyDocumentRule].
*/
@PulumiTagMarker
public class GetPolicyDocumentRuleBuilder internal constructor() {
private var allowedParameters: List? = null
private var capabilities: List? = null
private var deniedParameters: List? = null
private var description: String? = null
private var maxWrappingTtl: String? = null
private var minWrappingTtl: String? = null
private var path: String? = null
private var requiredParameters: List? = null
/**
* @param value Whitelists a list of keys and values that are permitted on the given path. See Parameters below.
*/
@JvmName("mgobwuvjtoupvkqf")
public suspend fun allowedParameters(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.allowedParameters = mapped
}
/**
* @param argument Whitelists a list of keys and values that are permitted on the given path. See Parameters below.
*/
@JvmName("scrgrxumcecmyoja")
public suspend fun allowedParameters(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetPolicyDocumentRuleAllowedParameterBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.allowedParameters = mapped
}
/**
* @param argument Whitelists a list of keys and values that are permitted on the given path. See Parameters below.
*/
@JvmName("hxrmmgvkpgicgyye")
public suspend fun allowedParameters(vararg argument: suspend GetPolicyDocumentRuleAllowedParameterBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetPolicyDocumentRuleAllowedParameterBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.allowedParameters = mapped
}
/**
* @param argument Whitelists a list of keys and values that are permitted on the given path. See Parameters below.
*/
@JvmName("ghtfdsvolwanjhys")
public suspend fun allowedParameters(argument: suspend GetPolicyDocumentRuleAllowedParameterBuilder.() -> Unit) {
val toBeMapped = listOf(
GetPolicyDocumentRuleAllowedParameterBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.allowedParameters = mapped
}
/**
* @param values Whitelists a list of keys and values that are permitted on the given path. See Parameters below.
*/
@JvmName("gffiaodiulcbwcdx")
public suspend fun allowedParameters(vararg values: GetPolicyDocumentRuleAllowedParameter) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.allowedParameters = mapped
}
/**
* @param value A list of capabilities that this rule apply to `path`. For example, ["read", "write"].
*/
@JvmName("rnowrmepmcwnhrgb")
public suspend fun capabilities(`value`: List) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.capabilities = mapped
}
/**
* @param values A list of capabilities that this rule apply to `path`. For example, ["read", "write"].
*/
@JvmName("xrexivfxoajasbqa")
public suspend fun capabilities(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.capabilities = mapped
}
/**
* @param value Blacklists a list of parameter and values. Any values specified here take precedence over `allowed_parameter`. See Parameters below.
*/
@JvmName("bdomlgggxjtsayay")
public suspend fun deniedParameters(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.deniedParameters = mapped
}
/**
* @param argument Blacklists a list of parameter and values. Any values specified here take precedence over `allowed_parameter`. See Parameters below.
*/
@JvmName("qjmuvhveupnmrrgo")
public suspend fun deniedParameters(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetPolicyDocumentRuleDeniedParameterBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.deniedParameters = mapped
}
/**
* @param argument Blacklists a list of parameter and values. Any values specified here take precedence over `allowed_parameter`. See Parameters below.
*/
@JvmName("cebheiirbipxfxtk")
public suspend fun deniedParameters(vararg argument: suspend GetPolicyDocumentRuleDeniedParameterBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetPolicyDocumentRuleDeniedParameterBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.deniedParameters = mapped
}
/**
* @param argument Blacklists a list of parameter and values. Any values specified here take precedence over `allowed_parameter`. See Parameters below.
*/
@JvmName("tipsqddntpdjjkjd")
public suspend fun deniedParameters(argument: suspend GetPolicyDocumentRuleDeniedParameterBuilder.() -> Unit) {
val toBeMapped = listOf(
GetPolicyDocumentRuleDeniedParameterBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.deniedParameters = mapped
}
/**
* @param values Blacklists a list of parameter and values. Any values specified here take precedence over `allowed_parameter`. See Parameters below.
*/
@JvmName("jvmllqmfpjhedakq")
public suspend fun deniedParameters(vararg values: GetPolicyDocumentRuleDeniedParameter) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.deniedParameters = mapped
}
/**
* @param value Description of the rule. Will be added as a comment to rendered rule.
*/
@JvmName("dqblakksolwfpwsx")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.description = mapped
}
/**
* @param value The maximum allowed TTL that clients can specify for a wrapped response.
*/
@JvmName("olfhrkdekjmgnpjd")
public suspend fun maxWrappingTtl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.maxWrappingTtl = mapped
}
/**
* @param value The minimum allowed TTL that clients can specify for a wrapped response.
*/
@JvmName("ihctqxtettpkehai")
public suspend fun minWrappingTtl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.minWrappingTtl = mapped
}
/**
* @param value A path in Vault that this rule applies to.
*/
@JvmName("llslvjeyahfadyxo")
public suspend fun path(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.path = mapped
}
/**
* @param value A list of parameters that must be specified.
*/
@JvmName("seudwasqjvmtwdxd")
public suspend fun requiredParameters(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.requiredParameters = mapped
}
/**
* @param values A list of parameters that must be specified.
*/
@JvmName("xllnfdimaqetdxmm")
public suspend fun requiredParameters(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.requiredParameters = mapped
}
internal fun build(): GetPolicyDocumentRule = GetPolicyDocumentRule(
allowedParameters = allowedParameters,
capabilities = capabilities ?: throw PulumiNullFieldException("capabilities"),
deniedParameters = deniedParameters,
description = description,
maxWrappingTtl = maxWrappingTtl,
minWrappingTtl = minWrappingTtl,
path = path ?: throw PulumiNullFieldException("path"),
requiredParameters = requiredParameters,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy