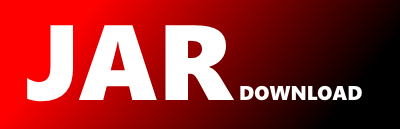
com.pulumi.vault.kubernetes.kotlin.KubernetesFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kubernetes.kotlin
import com.pulumi.vault.kubernetes.KubernetesFunctions.getAuthBackendConfigPlain
import com.pulumi.vault.kubernetes.KubernetesFunctions.getAuthBackendRolePlain
import com.pulumi.vault.kubernetes.KubernetesFunctions.getServiceAccountTokenPlain
import com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendConfigPlainArgs
import com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendConfigPlainArgsBuilder
import com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendRolePlainArgs
import com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendRolePlainArgsBuilder
import com.pulumi.vault.kubernetes.kotlin.inputs.GetServiceAccountTokenPlainArgs
import com.pulumi.vault.kubernetes.kotlin.inputs.GetServiceAccountTokenPlainArgsBuilder
import com.pulumi.vault.kubernetes.kotlin.outputs.GetAuthBackendConfigResult
import com.pulumi.vault.kubernetes.kotlin.outputs.GetAuthBackendRoleResult
import com.pulumi.vault.kubernetes.kotlin.outputs.GetServiceAccountTokenResult
import kotlinx.coroutines.future.await
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.vault.kubernetes.kotlin.outputs.GetAuthBackendConfigResult.Companion.toKotlin as getAuthBackendConfigResultToKotlin
import com.pulumi.vault.kubernetes.kotlin.outputs.GetAuthBackendRoleResult.Companion.toKotlin as getAuthBackendRoleResultToKotlin
import com.pulumi.vault.kubernetes.kotlin.outputs.GetServiceAccountTokenResult.Companion.toKotlin as getServiceAccountTokenResultToKotlin
public object KubernetesFunctions {
/**
* Reads the Role of an Kubernetes from a Vault server. See the [Vault
* documentation](https://www.vaultproject.io/api-docs/auth/kubernetes#read-config) for more
* information.
* @param argument A collection of arguments for invoking getAuthBackendConfig.
* @return A collection of values returned by getAuthBackendConfig.
*/
public suspend fun getAuthBackendConfig(argument: GetAuthBackendConfigPlainArgs): GetAuthBackendConfigResult =
getAuthBackendConfigResultToKotlin(getAuthBackendConfigPlain(argument.toJava()).await())
/**
* @see [getAuthBackendConfig].
* @param backend The unique name for the Kubernetes backend the config to
* retrieve Role attributes for resides in. Defaults to "kubernetes".
* @param disableIssValidation
* @param disableLocalCaJwt
* @param issuer Optional JWT issuer. If no issuer is specified, `kubernetes.io/serviceaccount` will be used as the default issuer.
* @param kubernetesCaCert PEM encoded CA cert for use by the TLS client used to talk with the Kubernetes API.
* @param kubernetesHost Host must be a host string, a host:port pair, or a URL to the base of the Kubernetes API server.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured namespace.
* *Available only for Vault Enterprise*.
* @param pemKeys Optional list of PEM-formatted public keys or certificates used to verify the signatures of Kubernetes service account JWTs. If a certificate is given, its public key will be extracted. Not every installation of Kubernetes exposes these keys.
* @return A collection of values returned by getAuthBackendConfig.
*/
public suspend fun getAuthBackendConfig(
backend: String? = null,
disableIssValidation: Boolean? = null,
disableLocalCaJwt: Boolean? = null,
issuer: String? = null,
kubernetesCaCert: String? = null,
kubernetesHost: String? = null,
namespace: String? = null,
pemKeys: List? = null,
): GetAuthBackendConfigResult {
val argument = GetAuthBackendConfigPlainArgs(
backend = backend,
disableIssValidation = disableIssValidation,
disableLocalCaJwt = disableLocalCaJwt,
issuer = issuer,
kubernetesCaCert = kubernetesCaCert,
kubernetesHost = kubernetesHost,
namespace = namespace,
pemKeys = pemKeys,
)
return getAuthBackendConfigResultToKotlin(getAuthBackendConfigPlain(argument.toJava()).await())
}
/**
* @see [getAuthBackendConfig].
* @param argument Builder for [com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendConfigPlainArgs].
* @return A collection of values returned by getAuthBackendConfig.
*/
public suspend fun getAuthBackendConfig(argument: suspend GetAuthBackendConfigPlainArgsBuilder.() -> Unit): GetAuthBackendConfigResult {
val builder = GetAuthBackendConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAuthBackendConfigResultToKotlin(getAuthBackendConfigPlain(builtArgument.toJava()).await())
}
/**
* Reads the Role of an Kubernetes from a Vault server. See the [Vault
* documentation](https://www.vaultproject.io/api-docs/auth/kubernetes#read-role) for more
* information.
* @param argument A collection of arguments for invoking getAuthBackendRole.
* @return A collection of values returned by getAuthBackendRole.
*/
public suspend fun getAuthBackendRole(argument: GetAuthBackendRolePlainArgs): GetAuthBackendRoleResult =
getAuthBackendRoleResultToKotlin(getAuthBackendRolePlain(argument.toJava()).await())
/**
* @see [getAuthBackendRole].
* @param audience Audience claim to verify in the JWT.
* @param backend The unique name for the Kubernetes backend the role to
* retrieve Role attributes for resides in. Defaults to "kubernetes".
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured namespace.
* *Available only for Vault Enterprise*.
* @param roleName The name of the role to retrieve the Role attributes for.
* @param tokenBoundCidrs List of CIDR blocks; if set, specifies blocks of IP
* addresses which can authenticate successfully, and ties the resulting token to these blocks
* as well.
* @param tokenExplicitMaxTtl If set, will encode an
* [explicit max TTL](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls)
* onto the token in number of seconds. This is a hard cap even if `token_ttl` and
* `token_max_ttl` would otherwise allow a renewal.
* @param tokenMaxTtl The maximum lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @param tokenNoDefaultPolicy If set, the default policy will not be set on
* generated tokens; otherwise it will be added to the policies set in token_policies.
* @param tokenNumUses The
* [period](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls),
* if any, in number of seconds to set on the token.
* @param tokenPeriod (Optional) If set, indicates that the
* token generated using this role should never expire. The token should be renewed within the
* duration specified by this value. At each renewal, the token's TTL will be set to the
* value of this field. Specified in seconds.
* @param tokenPolicies List of policies to encode onto generated tokens. Depending
* on the auth method, this list may be supplemented by user/group/other values.
* @param tokenTtl The incremental lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @param tokenType The type of token that should be generated. Can be `service`,
* `batch`, or `default` to use the mount's tuned default (which unless changed will be
* `service` tokens). For token store roles, there are two additional possibilities:
* `default-service` and `default-batch` which specify the type to return unless the client
* requests a different type at generation time.
* @return A collection of values returned by getAuthBackendRole.
*/
public suspend fun getAuthBackendRole(
audience: String? = null,
backend: String? = null,
namespace: String? = null,
roleName: String,
tokenBoundCidrs: List? = null,
tokenExplicitMaxTtl: Int? = null,
tokenMaxTtl: Int? = null,
tokenNoDefaultPolicy: Boolean? = null,
tokenNumUses: Int? = null,
tokenPeriod: Int? = null,
tokenPolicies: List? = null,
tokenTtl: Int? = null,
tokenType: String? = null,
): GetAuthBackendRoleResult {
val argument = GetAuthBackendRolePlainArgs(
audience = audience,
backend = backend,
namespace = namespace,
roleName = roleName,
tokenBoundCidrs = tokenBoundCidrs,
tokenExplicitMaxTtl = tokenExplicitMaxTtl,
tokenMaxTtl = tokenMaxTtl,
tokenNoDefaultPolicy = tokenNoDefaultPolicy,
tokenNumUses = tokenNumUses,
tokenPeriod = tokenPeriod,
tokenPolicies = tokenPolicies,
tokenTtl = tokenTtl,
tokenType = tokenType,
)
return getAuthBackendRoleResultToKotlin(getAuthBackendRolePlain(argument.toJava()).await())
}
/**
* @see [getAuthBackendRole].
* @param argument Builder for [com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendRolePlainArgs].
* @return A collection of values returned by getAuthBackendRole.
*/
public suspend fun getAuthBackendRole(argument: suspend GetAuthBackendRolePlainArgsBuilder.() -> Unit): GetAuthBackendRoleResult {
val builder = GetAuthBackendRolePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAuthBackendRoleResultToKotlin(getAuthBackendRolePlain(builtArgument.toJava()).await())
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as std from "@pulumi/std";
* import * as vault from "@pulumi/vault";
* const config = new vault.kubernetes.SecretBackend("config", {
* path: "kubernetes",
* description: "kubernetes secrets engine description",
* kubernetesHost: "https://127.0.0.1:61233",
* kubernetesCaCert: std.file({
* input: "/path/to/cert",
* }).then(invoke => invoke.result),
* serviceAccountJwt: std.file({
* input: "/path/to/token",
* }).then(invoke => invoke.result),
* disableLocalCaJwt: false,
* });
* const role = new vault.kubernetes.SecretBackendRole("role", {
* backend: config.path,
* name: "service-account-name-role",
* allowedKubernetesNamespaces: ["*"],
* tokenMaxTtl: 43200,
* tokenDefaultTtl: 21600,
* serviceAccountName: "test-service-account-with-generated-token",
* extraLabels: {
* id: "abc123",
* name: "some_name",
* },
* extraAnnotations: {
* env: "development",
* location: "earth",
* },
* });
* const token = vault.kubernetes.getServiceAccountTokenOutput({
* backend: config.path,
* role: role.name,
* kubernetesNamespace: "test",
* clusterRoleBinding: false,
* ttl: "1h",
* });
* ```
* ```python
* import pulumi
* import pulumi_std as std
* import pulumi_vault as vault
* config = vault.kubernetes.SecretBackend("config",
* path="kubernetes",
* description="kubernetes secrets engine description",
* kubernetes_host="https://127.0.0.1:61233",
* kubernetes_ca_cert=std.file(input="/path/to/cert").result,
* service_account_jwt=std.file(input="/path/to/token").result,
* disable_local_ca_jwt=False)
* role = vault.kubernetes.SecretBackendRole("role",
* backend=config.path,
* name="service-account-name-role",
* allowed_kubernetes_namespaces=["*"],
* token_max_ttl=43200,
* token_default_ttl=21600,
* service_account_name="test-service-account-with-generated-token",
* extra_labels={
* "id": "abc123",
* "name": "some_name",
* },
* extra_annotations={
* "env": "development",
* "location": "earth",
* })
* token = vault.kubernetes.get_service_account_token_output(backend=config.path,
* role=role.name,
* kubernetes_namespace="test",
* cluster_role_binding=False,
* ttl="1h")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Std = Pulumi.Std;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var config = new Vault.Kubernetes.SecretBackend("config", new()
* {
* Path = "kubernetes",
* Description = "kubernetes secrets engine description",
* KubernetesHost = "https://127.0.0.1:61233",
* KubernetesCaCert = Std.File.Invoke(new()
* {
* Input = "/path/to/cert",
* }).Apply(invoke => invoke.Result),
* ServiceAccountJwt = Std.File.Invoke(new()
* {
* Input = "/path/to/token",
* }).Apply(invoke => invoke.Result),
* DisableLocalCaJwt = false,
* });
* var role = new Vault.Kubernetes.SecretBackendRole("role", new()
* {
* Backend = config.Path,
* Name = "service-account-name-role",
* AllowedKubernetesNamespaces = new[]
* {
* "*",
* },
* TokenMaxTtl = 43200,
* TokenDefaultTtl = 21600,
* ServiceAccountName = "test-service-account-with-generated-token",
* ExtraLabels =
* {
* { "id", "abc123" },
* { "name", "some_name" },
* },
* ExtraAnnotations =
* {
* { "env", "development" },
* { "location", "earth" },
* },
* });
* var token = Vault.Kubernetes.GetServiceAccountToken.Invoke(new()
* {
* Backend = config.Path,
* Role = role.Name,
* KubernetesNamespace = "test",
* ClusterRoleBinding = false,
* Ttl = "1h",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kubernetes"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* invokeFile, err := std.File(ctx, &std.FileArgs{
* Input: "/path/to/cert",
* }, nil)
* if err != nil {
* return err
* }
* invokeFile1, err := std.File(ctx, &std.FileArgs{
* Input: "/path/to/token",
* }, nil)
* if err != nil {
* return err
* }
* config, err := kubernetes.NewSecretBackend(ctx, "config", &kubernetes.SecretBackendArgs{
* Path: pulumi.String("kubernetes"),
* Description: pulumi.String("kubernetes secrets engine description"),
* KubernetesHost: pulumi.String("https://127.0.0.1:61233"),
* KubernetesCaCert: pulumi.String(invokeFile.Result),
* ServiceAccountJwt: pulumi.String(invokeFile1.Result),
* DisableLocalCaJwt: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* role, err := kubernetes.NewSecretBackendRole(ctx, "role", &kubernetes.SecretBackendRoleArgs{
* Backend: config.Path,
* Name: pulumi.String("service-account-name-role"),
* AllowedKubernetesNamespaces: pulumi.StringArray{
* pulumi.String("*"),
* },
* TokenMaxTtl: pulumi.Int(43200),
* TokenDefaultTtl: pulumi.Int(21600),
* ServiceAccountName: pulumi.String("test-service-account-with-generated-token"),
* ExtraLabels: pulumi.StringMap{
* "id": pulumi.String("abc123"),
* "name": pulumi.String("some_name"),
* },
* ExtraAnnotations: pulumi.StringMap{
* "env": pulumi.String("development"),
* "location": pulumi.String("earth"),
* },
* })
* if err != nil {
* return err
* }
* _ = kubernetes.GetServiceAccountTokenOutput(ctx, kubernetes.GetServiceAccountTokenOutputArgs{
* Backend: config.Path,
* Role: role.Name,
* KubernetesNamespace: pulumi.String("test"),
* ClusterRoleBinding: pulumi.Bool(false),
* Ttl: pulumi.String("1h"),
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.kubernetes.SecretBackend;
* import com.pulumi.vault.kubernetes.SecretBackendArgs;
* import com.pulumi.vault.kubernetes.SecretBackendRole;
* import com.pulumi.vault.kubernetes.SecretBackendRoleArgs;
* import com.pulumi.vault.kubernetes.KubernetesFunctions;
* import com.pulumi.vault.kubernetes.inputs.GetServiceAccountTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var config = new SecretBackend("config", SecretBackendArgs.builder()
* .path("kubernetes")
* .description("kubernetes secrets engine description")
* .kubernetesHost("https://127.0.0.1:61233")
* .kubernetesCaCert(StdFunctions.file(FileArgs.builder()
* .input("/path/to/cert")
* .build()).result())
* .serviceAccountJwt(StdFunctions.file(FileArgs.builder()
* .input("/path/to/token")
* .build()).result())
* .disableLocalCaJwt(false)
* .build());
* var role = new SecretBackendRole("role", SecretBackendRoleArgs.builder()
* .backend(config.path())
* .name("service-account-name-role")
* .allowedKubernetesNamespaces("*")
* .tokenMaxTtl(43200)
* .tokenDefaultTtl(21600)
* .serviceAccountName("test-service-account-with-generated-token")
* .extraLabels(Map.ofEntries(
* Map.entry("id", "abc123"),
* Map.entry("name", "some_name")
* ))
* .extraAnnotations(Map.ofEntries(
* Map.entry("env", "development"),
* Map.entry("location", "earth")
* ))
* .build());
* final var token = KubernetesFunctions.getServiceAccountToken(GetServiceAccountTokenArgs.builder()
* .backend(config.path())
* .role(role.name())
* .kubernetesNamespace("test")
* .clusterRoleBinding(false)
* .ttl("1h")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* config:
* type: vault:kubernetes:SecretBackend
* properties:
* path: kubernetes
* description: kubernetes secrets engine description
* kubernetesHost: https://127.0.0.1:61233
* kubernetesCaCert:
* fn::invoke:
* Function: std:file
* Arguments:
* input: /path/to/cert
* Return: result
* serviceAccountJwt:
* fn::invoke:
* Function: std:file
* Arguments:
* input: /path/to/token
* Return: result
* disableLocalCaJwt: false
* role:
* type: vault:kubernetes:SecretBackendRole
* properties:
* backend: ${config.path}
* name: service-account-name-role
* allowedKubernetesNamespaces:
* - '*'
* tokenMaxTtl: 43200
* tokenDefaultTtl: 21600
* serviceAccountName: test-service-account-with-generated-token
* extraLabels:
* id: abc123
* name: some_name
* extraAnnotations:
* env: development
* location: earth
* variables:
* token:
* fn::invoke:
* Function: vault:kubernetes:getServiceAccountToken
* Arguments:
* backend: ${config.path}
* role: ${role.name}
* kubernetesNamespace: test
* clusterRoleBinding: false
* ttl: 1h
* ```
*
* @param argument A collection of arguments for invoking getServiceAccountToken.
* @return A collection of values returned by getServiceAccountToken.
*/
public suspend fun getServiceAccountToken(argument: GetServiceAccountTokenPlainArgs): GetServiceAccountTokenResult =
getServiceAccountTokenResultToKotlin(getServiceAccountTokenPlain(argument.toJava()).await())
/**
* @see [getServiceAccountToken].
* @param backend The Kubernetes secret backend to generate service account
* tokens from.
* @param clusterRoleBinding If true, generate a ClusterRoleBinding to grant
* permissions across the whole cluster instead of within a namespace.
* @param kubernetesNamespace The name of the Kubernetes namespace in which to
* generate the credentials.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param role The name of the Kubernetes secret backend role to generate service
* account tokens from.
* @param ttl The TTL of the generated Kubernetes service account token, specified in
* seconds or as a Go duration format string.
* @return A collection of values returned by getServiceAccountToken.
*/
public suspend fun getServiceAccountToken(
backend: String,
clusterRoleBinding: Boolean? = null,
kubernetesNamespace: String,
namespace: String? = null,
role: String,
ttl: String? = null,
): GetServiceAccountTokenResult {
val argument = GetServiceAccountTokenPlainArgs(
backend = backend,
clusterRoleBinding = clusterRoleBinding,
kubernetesNamespace = kubernetesNamespace,
namespace = namespace,
role = role,
ttl = ttl,
)
return getServiceAccountTokenResultToKotlin(getServiceAccountTokenPlain(argument.toJava()).await())
}
/**
* @see [getServiceAccountToken].
* @param argument Builder for [com.pulumi.vault.kubernetes.kotlin.inputs.GetServiceAccountTokenPlainArgs].
* @return A collection of values returned by getServiceAccountToken.
*/
public suspend fun getServiceAccountToken(argument: suspend GetServiceAccountTokenPlainArgsBuilder.() -> Unit): GetServiceAccountTokenResult {
val builder = GetServiceAccountTokenPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceAccountTokenResultToKotlin(getServiceAccountTokenPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy