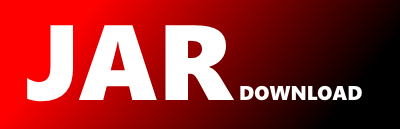
com.pulumi.vault.kubernetes.kotlin.inputs.GetAuthBackendRolePlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kubernetes.kotlin.inputs
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.kubernetes.inputs.GetAuthBackendRolePlainArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getAuthBackendRole.
* @property audience Audience claim to verify in the JWT.
* @property backend The unique name for the Kubernetes backend the role to
* retrieve Role attributes for resides in. Defaults to "kubernetes".
* @property namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured namespace.
* *Available only for Vault Enterprise*.
* @property roleName The name of the role to retrieve the Role attributes for.
* @property tokenBoundCidrs List of CIDR blocks; if set, specifies blocks of IP
* addresses which can authenticate successfully, and ties the resulting token to these blocks
* as well.
* @property tokenExplicitMaxTtl If set, will encode an
* [explicit max TTL](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls)
* onto the token in number of seconds. This is a hard cap even if `token_ttl` and
* `token_max_ttl` would otherwise allow a renewal.
* @property tokenMaxTtl The maximum lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @property tokenNoDefaultPolicy If set, the default policy will not be set on
* generated tokens; otherwise it will be added to the policies set in token_policies.
* @property tokenNumUses The
* [period](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls),
* if any, in number of seconds to set on the token.
* @property tokenPeriod (Optional) If set, indicates that the
* token generated using this role should never expire. The token should be renewed within the
* duration specified by this value. At each renewal, the token's TTL will be set to the
* value of this field. Specified in seconds.
* @property tokenPolicies List of policies to encode onto generated tokens. Depending
* on the auth method, this list may be supplemented by user/group/other values.
* @property tokenTtl The incremental lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
* @property tokenType The type of token that should be generated. Can be `service`,
* `batch`, or `default` to use the mount's tuned default (which unless changed will be
* `service` tokens). For token store roles, there are two additional possibilities:
* `default-service` and `default-batch` which specify the type to return unless the client
* requests a different type at generation time.
*/
public data class GetAuthBackendRolePlainArgs(
public val audience: String? = null,
public val backend: String? = null,
public val namespace: String? = null,
public val roleName: String,
public val tokenBoundCidrs: List? = null,
public val tokenExplicitMaxTtl: Int? = null,
public val tokenMaxTtl: Int? = null,
public val tokenNoDefaultPolicy: Boolean? = null,
public val tokenNumUses: Int? = null,
public val tokenPeriod: Int? = null,
public val tokenPolicies: List? = null,
public val tokenTtl: Int? = null,
public val tokenType: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.kubernetes.inputs.GetAuthBackendRolePlainArgs =
com.pulumi.vault.kubernetes.inputs.GetAuthBackendRolePlainArgs.builder()
.audience(audience?.let({ args0 -> args0 }))
.backend(backend?.let({ args0 -> args0 }))
.namespace(namespace?.let({ args0 -> args0 }))
.roleName(roleName.let({ args0 -> args0 }))
.tokenBoundCidrs(tokenBoundCidrs?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenExplicitMaxTtl(tokenExplicitMaxTtl?.let({ args0 -> args0 }))
.tokenMaxTtl(tokenMaxTtl?.let({ args0 -> args0 }))
.tokenNoDefaultPolicy(tokenNoDefaultPolicy?.let({ args0 -> args0 }))
.tokenNumUses(tokenNumUses?.let({ args0 -> args0 }))
.tokenPeriod(tokenPeriod?.let({ args0 -> args0 }))
.tokenPolicies(tokenPolicies?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenTtl(tokenTtl?.let({ args0 -> args0 }))
.tokenType(tokenType?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetAuthBackendRolePlainArgs].
*/
@PulumiTagMarker
public class GetAuthBackendRolePlainArgsBuilder internal constructor() {
private var audience: String? = null
private var backend: String? = null
private var namespace: String? = null
private var roleName: String? = null
private var tokenBoundCidrs: List? = null
private var tokenExplicitMaxTtl: Int? = null
private var tokenMaxTtl: Int? = null
private var tokenNoDefaultPolicy: Boolean? = null
private var tokenNumUses: Int? = null
private var tokenPeriod: Int? = null
private var tokenPolicies: List? = null
private var tokenTtl: Int? = null
private var tokenType: String? = null
/**
* @param value Audience claim to verify in the JWT.
*/
@JvmName("qsduumcpnxqpaoqd")
public suspend fun audience(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.audience = mapped
}
/**
* @param value The unique name for the Kubernetes backend the role to
* retrieve Role attributes for resides in. Defaults to "kubernetes".
*/
@JvmName("hohjldsjuisjytyn")
public suspend fun backend(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.backend = mapped
}
/**
* @param value The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured namespace.
* *Available only for Vault Enterprise*.
*/
@JvmName("mvudvwmosnkvafso")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.namespace = mapped
}
/**
* @param value The name of the role to retrieve the Role attributes for.
*/
@JvmName("tasvnxjcvcwihonl")
public suspend fun roleName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.roleName = mapped
}
/**
* @param value List of CIDR blocks; if set, specifies blocks of IP
* addresses which can authenticate successfully, and ties the resulting token to these blocks
* as well.
*/
@JvmName("ddkgdlolithhfhtj")
public suspend fun tokenBoundCidrs(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenBoundCidrs = mapped
}
/**
* @param values List of CIDR blocks; if set, specifies blocks of IP
* addresses which can authenticate successfully, and ties the resulting token to these blocks
* as well.
*/
@JvmName("dieexpfkkccanhik")
public suspend fun tokenBoundCidrs(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tokenBoundCidrs = mapped
}
/**
* @param value If set, will encode an
* [explicit max TTL](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls)
* onto the token in number of seconds. This is a hard cap even if `token_ttl` and
* `token_max_ttl` would otherwise allow a renewal.
*/
@JvmName("lniawhomjssywdlj")
public suspend fun tokenExplicitMaxTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenExplicitMaxTtl = mapped
}
/**
* @param value The maximum lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
*/
@JvmName("ywqbbtmvaaoharng")
public suspend fun tokenMaxTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenMaxTtl = mapped
}
/**
* @param value If set, the default policy will not be set on
* generated tokens; otherwise it will be added to the policies set in token_policies.
*/
@JvmName("tjelafntgjypvakq")
public suspend fun tokenNoDefaultPolicy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenNoDefaultPolicy = mapped
}
/**
* @param value The
* [period](https://www.vaultproject.io/docs/concepts/tokens.html#token-time-to-live-periodic-tokens-and-explicit-max-ttls),
* if any, in number of seconds to set on the token.
*/
@JvmName("qlqqkkgmqxhiinfl")
public suspend fun tokenNumUses(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenNumUses = mapped
}
/**
* @param value (Optional) If set, indicates that the
* token generated using this role should never expire. The token should be renewed within the
* duration specified by this value. At each renewal, the token's TTL will be set to the
* value of this field. Specified in seconds.
*/
@JvmName("carwuncosgfmghig")
public suspend fun tokenPeriod(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenPeriod = mapped
}
/**
* @param value List of policies to encode onto generated tokens. Depending
* on the auth method, this list may be supplemented by user/group/other values.
*/
@JvmName("hvodcioxswekfycy")
public suspend fun tokenPolicies(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenPolicies = mapped
}
/**
* @param values List of policies to encode onto generated tokens. Depending
* on the auth method, this list may be supplemented by user/group/other values.
*/
@JvmName("kbgpqfsrtgvxhfhu")
public suspend fun tokenPolicies(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tokenPolicies = mapped
}
/**
* @param value The incremental lifetime for generated tokens in number of seconds.
* Its current value will be referenced at renewal time.
*/
@JvmName("ehhpgqrqydyqlejl")
public suspend fun tokenTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenTtl = mapped
}
/**
* @param value The type of token that should be generated. Can be `service`,
* `batch`, or `default` to use the mount's tuned default (which unless changed will be
* `service` tokens). For token store roles, there are two additional possibilities:
* `default-service` and `default-batch` which specify the type to return unless the client
* requests a different type at generation time.
*/
@JvmName("ceosehmxhafevtpf")
public suspend fun tokenType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tokenType = mapped
}
internal fun build(): GetAuthBackendRolePlainArgs = GetAuthBackendRolePlainArgs(
audience = audience,
backend = backend,
namespace = namespace,
roleName = roleName ?: throw PulumiNullFieldException("roleName"),
tokenBoundCidrs = tokenBoundCidrs,
tokenExplicitMaxTtl = tokenExplicitMaxTtl,
tokenMaxTtl = tokenMaxTtl,
tokenNoDefaultPolicy = tokenNoDefaultPolicy,
tokenNumUses = tokenNumUses,
tokenPeriod = tokenPeriod,
tokenPolicies = tokenPolicies,
tokenTtl = tokenTtl,
tokenType = tokenType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy