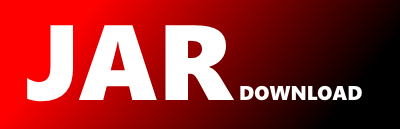
com.pulumi.vault.kv.kotlin.KvFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kv.kotlin
import com.pulumi.vault.kv.KvFunctions.getSecretPlain
import com.pulumi.vault.kv.KvFunctions.getSecretSubkeysV2Plain
import com.pulumi.vault.kv.KvFunctions.getSecretV2Plain
import com.pulumi.vault.kv.KvFunctions.getSecretsListPlain
import com.pulumi.vault.kv.KvFunctions.getSecretsListV2Plain
import com.pulumi.vault.kv.kotlin.inputs.GetSecretPlainArgs
import com.pulumi.vault.kv.kotlin.inputs.GetSecretPlainArgsBuilder
import com.pulumi.vault.kv.kotlin.inputs.GetSecretSubkeysV2PlainArgs
import com.pulumi.vault.kv.kotlin.inputs.GetSecretSubkeysV2PlainArgsBuilder
import com.pulumi.vault.kv.kotlin.inputs.GetSecretV2PlainArgs
import com.pulumi.vault.kv.kotlin.inputs.GetSecretV2PlainArgsBuilder
import com.pulumi.vault.kv.kotlin.inputs.GetSecretsListPlainArgs
import com.pulumi.vault.kv.kotlin.inputs.GetSecretsListPlainArgsBuilder
import com.pulumi.vault.kv.kotlin.inputs.GetSecretsListV2PlainArgs
import com.pulumi.vault.kv.kotlin.inputs.GetSecretsListV2PlainArgsBuilder
import com.pulumi.vault.kv.kotlin.outputs.GetSecretResult
import com.pulumi.vault.kv.kotlin.outputs.GetSecretSubkeysV2Result
import com.pulumi.vault.kv.kotlin.outputs.GetSecretV2Result
import com.pulumi.vault.kv.kotlin.outputs.GetSecretsListResult
import com.pulumi.vault.kv.kotlin.outputs.GetSecretsListV2Result
import kotlinx.coroutines.future.await
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.vault.kv.kotlin.outputs.GetSecretResult.Companion.toKotlin as getSecretResultToKotlin
import com.pulumi.vault.kv.kotlin.outputs.GetSecretSubkeysV2Result.Companion.toKotlin as getSecretSubkeysV2ResultToKotlin
import com.pulumi.vault.kv.kotlin.outputs.GetSecretV2Result.Companion.toKotlin as getSecretV2ResultToKotlin
import com.pulumi.vault.kv.kotlin.outputs.GetSecretsListResult.Companion.toKotlin as getSecretsListResultToKotlin
import com.pulumi.vault.kv.kotlin.outputs.GetSecretsListV2Result.Companion.toKotlin as getSecretsListV2ResultToKotlin
public object KvFunctions {
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const kvv1 = new vault.Mount("kvv1", {
* path: "kvv1",
* type: "kv",
* options: {
* version: "1",
* },
* description: "KV Version 1 secret engine mount",
* });
* const secret = new vault.kv.Secret("secret", {
* path: pulumi.interpolate`${kvv1.path}/secret`,
* dataJson: JSON.stringify({
* zip: "zap",
* foo: "bar",
* }),
* });
* const secretData = vault.kv.getSecretOutput({
* path: secret.path,
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_vault as vault
* kvv1 = vault.Mount("kvv1",
* path="kvv1",
* type="kv",
* options={
* "version": "1",
* },
* description="KV Version 1 secret engine mount")
* secret = vault.kv.Secret("secret",
* path=kvv1.path.apply(lambda path: f"{path}/secret"),
* data_json=json.dumps({
* "zip": "zap",
* "foo": "bar",
* }))
* secret_data = vault.kv.get_secret_output(path=secret.path)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var kvv1 = new Vault.Mount("kvv1", new()
* {
* Path = "kvv1",
* Type = "kv",
* Options =
* {
* { "version", "1" },
* },
* Description = "KV Version 1 secret engine mount",
* });
* var secret = new Vault.Kv.Secret("secret", new()
* {
* Path = kvv1.Path.Apply(path => $"{path}/secret"),
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["zip"] = "zap",
* ["foo"] = "bar",
* }),
* });
* var secretData = Vault.kv.GetSecret.Invoke(new()
* {
* Path = secret.Path,
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "fmt"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kv"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* kvv1, err := vault.NewMount(ctx, "kvv1", &vault.MountArgs{
* Path: pulumi.String("kvv1"),
* Type: pulumi.String("kv"),
* Options: pulumi.Map{
* "version": pulumi.Any("1"),
* },
* Description: pulumi.String("KV Version 1 secret engine mount"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "zip": "zap",
* "foo": "bar",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* secret, err := kv.NewSecret(ctx, "secret", &kv.SecretArgs{
* Path: kvv1.Path.ApplyT(func(path string) (string, error) {
* return fmt.Sprintf("%v/secret", path), nil
* }).(pulumi.StringOutput),
* DataJson: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* _ = kv.LookupSecretOutput(ctx, kv.GetSecretOutputArgs{
* Path: secret.Path,
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.kv.Secret;
* import com.pulumi.vault.kv.SecretArgs;
* import com.pulumi.vault.kv.KvFunctions;
* import com.pulumi.vault.kv.inputs.GetSecretArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kvv1 = new Mount("kvv1", MountArgs.builder()
* .path("kvv1")
* .type("kv")
* .options(Map.of("version", "1"))
* .description("KV Version 1 secret engine mount")
* .build());
* var secret = new Secret("secret", SecretArgs.builder()
* .path(kvv1.path().applyValue(path -> String.format("%s/secret", path)))
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("zip", "zap"),
* jsonProperty("foo", "bar")
* )))
* .build());
* final var secretData = KvFunctions.getSecret(GetSecretArgs.builder()
* .path(secret.path())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kvv1:
* type: vault:Mount
* properties:
* path: kvv1
* type: kv
* options:
* version: '1'
* description: KV Version 1 secret engine mount
* secret:
* type: vault:kv:Secret
* properties:
* path: ${kvv1.path}/secret
* dataJson:
* fn::toJSON:
* zip: zap
* foo: bar
* variables:
* secretData:
* fn::invoke:
* Function: vault:kv:getSecret
* Arguments:
* path: ${secret.path}
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `read` capability on the given path.
* @param argument A collection of arguments for invoking getSecret.
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(argument: GetSecretPlainArgs): GetSecretResult =
getSecretResultToKotlin(getSecretPlain(argument.toJava()).await())
/**
* @see [getSecret].
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param path Full path of the KV-V1 secret.
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(namespace: String? = null, path: String): GetSecretResult {
val argument = GetSecretPlainArgs(
namespace = namespace,
path = path,
)
return getSecretResultToKotlin(getSecretPlain(argument.toJava()).await())
}
/**
* @see [getSecret].
* @param argument Builder for [com.pulumi.vault.kv.kotlin.inputs.GetSecretPlainArgs].
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(argument: suspend GetSecretPlainArgsBuilder.() -> Unit): GetSecretResult {
val builder = GetSecretPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretResultToKotlin(getSecretPlain(builtArgument.toJava()).await())
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const kvv2 = new vault.Mount("kvv2", {
* path: "kvv2",
* type: "kv",
* options: {
* version: "2",
* },
* description: "KV Version 2 secret engine mount",
* });
* const awsSecret = new vault.kv.SecretV2("aws_secret", {
* mount: kvv2.path,
* name: "aws_secret",
* dataJson: JSON.stringify({
* zip: "zap",
* foo: "bar",
* }),
* });
* const test = vault.kv.getSecretSubkeysV2Output({
* mount: kvv2.path,
* name: awsSecret.name,
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_vault as vault
* kvv2 = vault.Mount("kvv2",
* path="kvv2",
* type="kv",
* options={
* "version": "2",
* },
* description="KV Version 2 secret engine mount")
* aws_secret = vault.kv.SecretV2("aws_secret",
* mount=kvv2.path,
* name="aws_secret",
* data_json=json.dumps({
* "zip": "zap",
* "foo": "bar",
* }))
* test = vault.kv.get_secret_subkeys_v2_output(mount=kvv2.path,
* name=aws_secret.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var kvv2 = new Vault.Mount("kvv2", new()
* {
* Path = "kvv2",
* Type = "kv",
* Options =
* {
* { "version", "2" },
* },
* Description = "KV Version 2 secret engine mount",
* });
* var awsSecret = new Vault.Kv.SecretV2("aws_secret", new()
* {
* Mount = kvv2.Path,
* Name = "aws_secret",
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["zip"] = "zap",
* ["foo"] = "bar",
* }),
* });
* var test = Vault.kv.GetSecretSubkeysV2.Invoke(new()
* {
* Mount = kvv2.Path,
* Name = awsSecret.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kv"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* kvv2, err := vault.NewMount(ctx, "kvv2", &vault.MountArgs{
* Path: pulumi.String("kvv2"),
* Type: pulumi.String("kv"),
* Options: pulumi.Map{
* "version": pulumi.Any("2"),
* },
* Description: pulumi.String("KV Version 2 secret engine mount"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "zip": "zap",
* "foo": "bar",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* awsSecret, err := kv.NewSecretV2(ctx, "aws_secret", &kv.SecretV2Args{
* Mount: kvv2.Path,
* Name: pulumi.String("aws_secret"),
* DataJson: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* _ = kv.GetSecretSubkeysV2Output(ctx, kv.GetSecretSubkeysV2OutputArgs{
* Mount: kvv2.Path,
* Name: awsSecret.Name,
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.kv.SecretV2;
* import com.pulumi.vault.kv.SecretV2Args;
* import com.pulumi.vault.kv.KvFunctions;
* import com.pulumi.vault.kv.inputs.GetSecretSubkeysV2Args;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kvv2 = new Mount("kvv2", MountArgs.builder()
* .path("kvv2")
* .type("kv")
* .options(Map.of("version", "2"))
* .description("KV Version 2 secret engine mount")
* .build());
* var awsSecret = new SecretV2("awsSecret", SecretV2Args.builder()
* .mount(kvv2.path())
* .name("aws_secret")
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("zip", "zap"),
* jsonProperty("foo", "bar")
* )))
* .build());
* final var test = KvFunctions.getSecretSubkeysV2(GetSecretSubkeysV2Args.builder()
* .mount(kvv2.path())
* .name(awsSecret.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kvv2:
* type: vault:Mount
* properties:
* path: kvv2
* type: kv
* options:
* version: '2'
* description: KV Version 2 secret engine mount
* awsSecret:
* type: vault:kv:SecretV2
* name: aws_secret
* properties:
* mount: ${kvv2.path}
* name: aws_secret
* dataJson:
* fn::toJSON:
* zip: zap
* foo: bar
* variables:
* test:
* fn::invoke:
* Function: vault:kv:getSecretSubkeysV2
* Arguments:
* mount: ${kvv2.path}
* name: ${awsSecret.name}
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `read` capability on the given path.
* @param argument A collection of arguments for invoking getSecretSubkeysV2.
* @return A collection of values returned by getSecretSubkeysV2.
*/
public suspend fun getSecretSubkeysV2(argument: GetSecretSubkeysV2PlainArgs): GetSecretSubkeysV2Result =
getSecretSubkeysV2ResultToKotlin(getSecretSubkeysV2Plain(argument.toJava()).await())
/**
* @see [getSecretSubkeysV2].
* @param depth Specifies the deepest nesting level to provide in the output.
* If non-zero, keys that reside at the specified depth value will be
* artificially treated as leaves and will thus be `null` even if further
* underlying sub-keys exist.
* @param mount Path where KV-V2 engine is mounted.
* @param name Full name of the secret. For a nested secret
* the name is the nested path excluding the mount and data
* prefix. For example, for a secret at `kvv2/data/foo/bar/baz`
* the name is `foo/bar/baz`.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param version Specifies the version to return. If not
* set the latest version is returned.
* @return A collection of values returned by getSecretSubkeysV2.
*/
public suspend fun getSecretSubkeysV2(
depth: Int? = null,
mount: String,
name: String,
namespace: String? = null,
version: Int? = null,
): GetSecretSubkeysV2Result {
val argument = GetSecretSubkeysV2PlainArgs(
depth = depth,
mount = mount,
name = name,
namespace = namespace,
version = version,
)
return getSecretSubkeysV2ResultToKotlin(getSecretSubkeysV2Plain(argument.toJava()).await())
}
/**
* @see [getSecretSubkeysV2].
* @param argument Builder for [com.pulumi.vault.kv.kotlin.inputs.GetSecretSubkeysV2PlainArgs].
* @return A collection of values returned by getSecretSubkeysV2.
*/
public suspend fun getSecretSubkeysV2(argument: suspend GetSecretSubkeysV2PlainArgsBuilder.() -> Unit): GetSecretSubkeysV2Result {
val builder = GetSecretSubkeysV2PlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretSubkeysV2ResultToKotlin(getSecretSubkeysV2Plain(builtArgument.toJava()).await())
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const kvv2 = new vault.Mount("kvv2", {
* path: "kvv2",
* type: "kv",
* options: {
* version: "2",
* },
* description: "KV Version 2 secret engine mount",
* });
* const exampleSecretV2 = new vault.kv.SecretV2("example", {
* mount: kvv2.path,
* name: "secret",
* cas: 1,
* deleteAllVersions: true,
* dataJson: JSON.stringify({
* zip: "zap",
* foo: "bar",
* }),
* });
* const example = vault.kv.getSecretV2Output({
* mount: kvv2.path,
* name: exampleSecretV2.name,
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_vault as vault
* kvv2 = vault.Mount("kvv2",
* path="kvv2",
* type="kv",
* options={
* "version": "2",
* },
* description="KV Version 2 secret engine mount")
* example_secret_v2 = vault.kv.SecretV2("example",
* mount=kvv2.path,
* name="secret",
* cas=1,
* delete_all_versions=True,
* data_json=json.dumps({
* "zip": "zap",
* "foo": "bar",
* }))
* example = vault.kv.get_secret_v2_output(mount=kvv2.path,
* name=example_secret_v2.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var kvv2 = new Vault.Mount("kvv2", new()
* {
* Path = "kvv2",
* Type = "kv",
* Options =
* {
* { "version", "2" },
* },
* Description = "KV Version 2 secret engine mount",
* });
* var exampleSecretV2 = new Vault.Kv.SecretV2("example", new()
* {
* Mount = kvv2.Path,
* Name = "secret",
* Cas = 1,
* DeleteAllVersions = true,
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["zip"] = "zap",
* ["foo"] = "bar",
* }),
* });
* var example = Vault.kv.GetSecretV2.Invoke(new()
* {
* Mount = kvv2.Path,
* Name = exampleSecretV2.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kv"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* kvv2, err := vault.NewMount(ctx, "kvv2", &vault.MountArgs{
* Path: pulumi.String("kvv2"),
* Type: pulumi.String("kv"),
* Options: pulumi.Map{
* "version": pulumi.Any("2"),
* },
* Description: pulumi.String("KV Version 2 secret engine mount"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "zip": "zap",
* "foo": "bar",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* exampleSecretV2, err := kv.NewSecretV2(ctx, "example", &kv.SecretV2Args{
* Mount: kvv2.Path,
* Name: pulumi.String("secret"),
* Cas: pulumi.Int(1),
* DeleteAllVersions: pulumi.Bool(true),
* DataJson: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* _ = kv.LookupSecretV2Output(ctx, kv.GetSecretV2OutputArgs{
* Mount: kvv2.Path,
* Name: exampleSecretV2.Name,
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.kv.SecretV2;
* import com.pulumi.vault.kv.SecretV2Args;
* import com.pulumi.vault.kv.KvFunctions;
* import com.pulumi.vault.kv.inputs.GetSecretV2Args;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kvv2 = new Mount("kvv2", MountArgs.builder()
* .path("kvv2")
* .type("kv")
* .options(Map.of("version", "2"))
* .description("KV Version 2 secret engine mount")
* .build());
* var exampleSecretV2 = new SecretV2("exampleSecretV2", SecretV2Args.builder()
* .mount(kvv2.path())
* .name("secret")
* .cas(1)
* .deleteAllVersions(true)
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("zip", "zap"),
* jsonProperty("foo", "bar")
* )))
* .build());
* final var example = KvFunctions.getSecretV2(GetSecretV2Args.builder()
* .mount(kvv2.path())
* .name(exampleSecretV2.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kvv2:
* type: vault:Mount
* properties:
* path: kvv2
* type: kv
* options:
* version: '2'
* description: KV Version 2 secret engine mount
* exampleSecretV2:
* type: vault:kv:SecretV2
* name: example
* properties:
* mount: ${kvv2.path}
* name: secret
* cas: 1
* deleteAllVersions: true
* dataJson:
* fn::toJSON:
* zip: zap
* foo: bar
* variables:
* example:
* fn::invoke:
* Function: vault:kv:getSecretV2
* Arguments:
* mount: ${kvv2.path}
* name: ${exampleSecretV2.name}
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `read` capability on the given path.
* @param argument A collection of arguments for invoking getSecretV2.
* @return A collection of values returned by getSecretV2.
*/
public suspend fun getSecretV2(argument: GetSecretV2PlainArgs): GetSecretV2Result =
getSecretV2ResultToKotlin(getSecretV2Plain(argument.toJava()).await())
/**
* @see [getSecretV2].
* @param mount Path where KV-V2 engine is mounted.
* @param name Full name of the secret. For a nested secret
* the name is the nested path excluding the mount and data
* prefix. For example, for a secret at `kvv2/data/foo/bar/baz`
* the name is `foo/bar/baz`.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param version Version of the secret to retrieve.
* @return A collection of values returned by getSecretV2.
*/
public suspend fun getSecretV2(
mount: String,
name: String,
namespace: String? = null,
version: Int? = null,
): GetSecretV2Result {
val argument = GetSecretV2PlainArgs(
mount = mount,
name = name,
namespace = namespace,
version = version,
)
return getSecretV2ResultToKotlin(getSecretV2Plain(argument.toJava()).await())
}
/**
* @see [getSecretV2].
* @param argument Builder for [com.pulumi.vault.kv.kotlin.inputs.GetSecretV2PlainArgs].
* @return A collection of values returned by getSecretV2.
*/
public suspend fun getSecretV2(argument: suspend GetSecretV2PlainArgsBuilder.() -> Unit): GetSecretV2Result {
val builder = GetSecretV2PlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretV2ResultToKotlin(getSecretV2Plain(builtArgument.toJava()).await())
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const kvv1 = new vault.Mount("kvv1", {
* path: "kvv1",
* type: "kv",
* options: {
* version: "1",
* },
* description: "KV Version 1 secret engine mount",
* });
* const awsSecret = new vault.kv.Secret("aws_secret", {
* path: pulumi.interpolate`${kvv1.path}/aws-secret`,
* dataJson: JSON.stringify({
* zip: "zap",
* }),
* });
* const azureSecret = new vault.kv.Secret("azure_secret", {
* path: pulumi.interpolate`${kvv1.path}/azure-secret`,
* dataJson: JSON.stringify({
* foo: "bar",
* }),
* });
* const secrets = vault.kv.getSecretsListOutput({
* path: kvv1.path,
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_vault as vault
* kvv1 = vault.Mount("kvv1",
* path="kvv1",
* type="kv",
* options={
* "version": "1",
* },
* description="KV Version 1 secret engine mount")
* aws_secret = vault.kv.Secret("aws_secret",
* path=kvv1.path.apply(lambda path: f"{path}/aws-secret"),
* data_json=json.dumps({
* "zip": "zap",
* }))
* azure_secret = vault.kv.Secret("azure_secret",
* path=kvv1.path.apply(lambda path: f"{path}/azure-secret"),
* data_json=json.dumps({
* "foo": "bar",
* }))
* secrets = vault.kv.get_secrets_list_output(path=kvv1.path)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var kvv1 = new Vault.Mount("kvv1", new()
* {
* Path = "kvv1",
* Type = "kv",
* Options =
* {
* { "version", "1" },
* },
* Description = "KV Version 1 secret engine mount",
* });
* var awsSecret = new Vault.Kv.Secret("aws_secret", new()
* {
* Path = kvv1.Path.Apply(path => $"{path}/aws-secret"),
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["zip"] = "zap",
* }),
* });
* var azureSecret = new Vault.Kv.Secret("azure_secret", new()
* {
* Path = kvv1.Path.Apply(path => $"{path}/azure-secret"),
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["foo"] = "bar",
* }),
* });
* var secrets = Vault.kv.GetSecretsList.Invoke(new()
* {
* Path = kvv1.Path,
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "fmt"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kv"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* kvv1, err := vault.NewMount(ctx, "kvv1", &vault.MountArgs{
* Path: pulumi.String("kvv1"),
* Type: pulumi.String("kv"),
* Options: pulumi.Map{
* "version": pulumi.Any("1"),
* },
* Description: pulumi.String("KV Version 1 secret engine mount"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "zip": "zap",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = kv.NewSecret(ctx, "aws_secret", &kv.SecretArgs{
* Path: kvv1.Path.ApplyT(func(path string) (string, error) {
* return fmt.Sprintf("%v/aws-secret", path), nil
* }).(pulumi.StringOutput),
* DataJson: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* tmpJSON1, err := json.Marshal(map[string]interface{}{
* "foo": "bar",
* })
* if err != nil {
* return err
* }
* json1 := string(tmpJSON1)
* _, err = kv.NewSecret(ctx, "azure_secret", &kv.SecretArgs{
* Path: kvv1.Path.ApplyT(func(path string) (string, error) {
* return fmt.Sprintf("%v/azure-secret", path), nil
* }).(pulumi.StringOutput),
* DataJson: pulumi.String(json1),
* })
* if err != nil {
* return err
* }
* _ = kv.GetSecretsListOutput(ctx, kv.GetSecretsListOutputArgs{
* Path: kvv1.Path,
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.kv.Secret;
* import com.pulumi.vault.kv.SecretArgs;
* import com.pulumi.vault.kv.KvFunctions;
* import com.pulumi.vault.kv.inputs.GetSecretsListArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kvv1 = new Mount("kvv1", MountArgs.builder()
* .path("kvv1")
* .type("kv")
* .options(Map.of("version", "1"))
* .description("KV Version 1 secret engine mount")
* .build());
* var awsSecret = new Secret("awsSecret", SecretArgs.builder()
* .path(kvv1.path().applyValue(path -> String.format("%s/aws-secret", path)))
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("zip", "zap")
* )))
* .build());
* var azureSecret = new Secret("azureSecret", SecretArgs.builder()
* .path(kvv1.path().applyValue(path -> String.format("%s/azure-secret", path)))
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("foo", "bar")
* )))
* .build());
* final var secrets = KvFunctions.getSecretsList(GetSecretsListArgs.builder()
* .path(kvv1.path())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kvv1:
* type: vault:Mount
* properties:
* path: kvv1
* type: kv
* options:
* version: '1'
* description: KV Version 1 secret engine mount
* awsSecret:
* type: vault:kv:Secret
* name: aws_secret
* properties:
* path: ${kvv1.path}/aws-secret
* dataJson:
* fn::toJSON:
* zip: zap
* azureSecret:
* type: vault:kv:Secret
* name: azure_secret
* properties:
* path: ${kvv1.path}/azure-secret
* dataJson:
* fn::toJSON:
* foo: bar
* variables:
* secrets:
* fn::invoke:
* Function: vault:kv:getSecretsList
* Arguments:
* path: ${kvv1.path}
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `read` capability on the given path.
* @param argument A collection of arguments for invoking getSecretsList.
* @return A collection of values returned by getSecretsList.
*/
public suspend fun getSecretsList(argument: GetSecretsListPlainArgs): GetSecretsListResult =
getSecretsListResultToKotlin(getSecretsListPlain(argument.toJava()).await())
/**
* @see [getSecretsList].
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param path Full KV-V1 path where secrets will be listed.
* @return A collection of values returned by getSecretsList.
*/
public suspend fun getSecretsList(namespace: String? = null, path: String): GetSecretsListResult {
val argument = GetSecretsListPlainArgs(
namespace = namespace,
path = path,
)
return getSecretsListResultToKotlin(getSecretsListPlain(argument.toJava()).await())
}
/**
* @see [getSecretsList].
* @param argument Builder for [com.pulumi.vault.kv.kotlin.inputs.GetSecretsListPlainArgs].
* @return A collection of values returned by getSecretsList.
*/
public suspend fun getSecretsList(argument: suspend GetSecretsListPlainArgsBuilder.() -> Unit): GetSecretsListResult {
val builder = GetSecretsListPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretsListResultToKotlin(getSecretsListPlain(builtArgument.toJava()).await())
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const kvv2 = new vault.Mount("kvv2", {
* path: "kvv2",
* type: "kv",
* options: {
* version: "2",
* },
* description: "KV Version 2 secret engine mount",
* });
* const awsSecret = new vault.kv.SecretV2("aws_secret", {
* mount: kvv2.path,
* name: "aws_secret",
* dataJson: JSON.stringify({
* zip: "zap",
* }),
* });
* const azureSecret = new vault.kv.SecretV2("azure_secret", {
* mount: kvv2.path,
* name: "azure_secret",
* dataJson: JSON.stringify({
* foo: "bar",
* }),
* });
* const nestedSecret = new vault.kv.SecretV2("nested_secret", {
* mount: kvv2.path,
* name: pulumi.interpolate`${azureSecret.name}/dev`,
* dataJson: JSON.stringify({
* password: "test",
* }),
* });
* const secrets = vault.kv.getSecretsListV2Output({
* mount: kvv2.path,
* });
* const nestedSecrets = kvv2.path.apply(path => vault.kv.getSecretsListV2Output({
* mount: path,
* name: test2.name,
* }));
* ```
* ```python
* import pulumi
* import json
* import pulumi_vault as vault
* kvv2 = vault.Mount("kvv2",
* path="kvv2",
* type="kv",
* options={
* "version": "2",
* },
* description="KV Version 2 secret engine mount")
* aws_secret = vault.kv.SecretV2("aws_secret",
* mount=kvv2.path,
* name="aws_secret",
* data_json=json.dumps({
* "zip": "zap",
* }))
* azure_secret = vault.kv.SecretV2("azure_secret",
* mount=kvv2.path,
* name="azure_secret",
* data_json=json.dumps({
* "foo": "bar",
* }))
* nested_secret = vault.kv.SecretV2("nested_secret",
* mount=kvv2.path,
* name=azure_secret.name.apply(lambda name: f"{name}/dev"),
* data_json=json.dumps({
* "password": "test",
* }))
* secrets = vault.kv.get_secrets_list_v2_output(mount=kvv2.path)
* nested_secrets = kvv2.path.apply(lambda path: vault.kv.get_secrets_list_v2_output(mount=path,
* name=test2["name"]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var kvv2 = new Vault.Mount("kvv2", new()
* {
* Path = "kvv2",
* Type = "kv",
* Options =
* {
* { "version", "2" },
* },
* Description = "KV Version 2 secret engine mount",
* });
* var awsSecret = new Vault.Kv.SecretV2("aws_secret", new()
* {
* Mount = kvv2.Path,
* Name = "aws_secret",
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["zip"] = "zap",
* }),
* });
* var azureSecret = new Vault.Kv.SecretV2("azure_secret", new()
* {
* Mount = kvv2.Path,
* Name = "azure_secret",
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["foo"] = "bar",
* }),
* });
* var nestedSecret = new Vault.Kv.SecretV2("nested_secret", new()
* {
* Mount = kvv2.Path,
* Name = azureSecret.Name.Apply(name => $"{name}/dev"),
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["password"] = "test",
* }),
* });
* var secrets = Vault.kv.GetSecretsListV2.Invoke(new()
* {
* Mount = kvv2.Path,
* });
* var nestedSecrets = Vault.kv.GetSecretsListV2.Invoke(new()
* {
* Mount = kvv2.Path,
* Name = test2.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "fmt"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kv"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* kvv2, err := vault.NewMount(ctx, "kvv2", &vault.MountArgs{
* Path: pulumi.String("kvv2"),
* Type: pulumi.String("kv"),
* Options: pulumi.Map{
* "version": pulumi.Any("2"),
* },
* Description: pulumi.String("KV Version 2 secret engine mount"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "zip": "zap",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = kv.NewSecretV2(ctx, "aws_secret", &kv.SecretV2Args{
* Mount: kvv2.Path,
* Name: pulumi.String("aws_secret"),
* DataJson: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* tmpJSON1, err := json.Marshal(map[string]interface{}{
* "foo": "bar",
* })
* if err != nil {
* return err
* }
* json1 := string(tmpJSON1)
* azureSecret, err := kv.NewSecretV2(ctx, "azure_secret", &kv.SecretV2Args{
* Mount: kvv2.Path,
* Name: pulumi.String("azure_secret"),
* DataJson: pulumi.String(json1),
* })
* if err != nil {
* return err
* }
* tmpJSON2, err := json.Marshal(map[string]interface{}{
* "password": "test",
* })
* if err != nil {
* return err
* }
* json2 := string(tmpJSON2)
* _, err = kv.NewSecretV2(ctx, "nested_secret", &kv.SecretV2Args{
* Mount: kvv2.Path,
* Name: azureSecret.Name.ApplyT(func(name string) (string, error) {
* return fmt.Sprintf("%v/dev", name), nil
* }).(pulumi.StringOutput),
* DataJson: pulumi.String(json2),
* })
* if err != nil {
* return err
* }
* _ = kv.GetSecretsListV2Output(ctx, kv.GetSecretsListV2OutputArgs{
* Mount: kvv2.Path,
* }, nil)
* _ = kvv2.Path.ApplyT(func(path string) (kv.GetSecretsListV2Result, error) {
* return kv.GetSecretsListV2Result(interface{}(kv.GetSecretsListV2Output(ctx, kv.GetSecretsListV2OutputArgs{
* Mount: path,
* Name: test2.Name,
* }, nil))), nil
* }).(kv.GetSecretsListV2ResultOutput)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.kv.SecretV2;
* import com.pulumi.vault.kv.SecretV2Args;
* import com.pulumi.vault.kv.KvFunctions;
* import com.pulumi.vault.kv.inputs.GetSecretsListV2Args;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kvv2 = new Mount("kvv2", MountArgs.builder()
* .path("kvv2")
* .type("kv")
* .options(Map.of("version", "2"))
* .description("KV Version 2 secret engine mount")
* .build());
* var awsSecret = new SecretV2("awsSecret", SecretV2Args.builder()
* .mount(kvv2.path())
* .name("aws_secret")
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("zip", "zap")
* )))
* .build());
* var azureSecret = new SecretV2("azureSecret", SecretV2Args.builder()
* .mount(kvv2.path())
* .name("azure_secret")
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("foo", "bar")
* )))
* .build());
* var nestedSecret = new SecretV2("nestedSecret", SecretV2Args.builder()
* .mount(kvv2.path())
* .name(azureSecret.name().applyValue(name -> String.format("%s/dev", name)))
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("password", "test")
* )))
* .build());
* final var secrets = KvFunctions.getSecretsListV2(GetSecretsListV2Args.builder()
* .mount(kvv2.path())
* .build());
* final var nestedSecrets = KvFunctions.getSecretsListV2(GetSecretsListV2Args.builder()
* .mount(kvv2.path())
* .name(test2.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kvv2:
* type: vault:Mount
* properties:
* path: kvv2
* type: kv
* options:
* version: '2'
* description: KV Version 2 secret engine mount
* awsSecret:
* type: vault:kv:SecretV2
* name: aws_secret
* properties:
* mount: ${kvv2.path}
* name: aws_secret
* dataJson:
* fn::toJSON:
* zip: zap
* azureSecret:
* type: vault:kv:SecretV2
* name: azure_secret
* properties:
* mount: ${kvv2.path}
* name: azure_secret
* dataJson:
* fn::toJSON:
* foo: bar
* nestedSecret:
* type: vault:kv:SecretV2
* name: nested_secret
* properties:
* mount: ${kvv2.path}
* name: ${azureSecret.name}/dev
* dataJson:
* fn::toJSON:
* password: test
* variables:
* secrets:
* fn::invoke:
* Function: vault:kv:getSecretsListV2
* Arguments:
* mount: ${kvv2.path}
* nestedSecrets:
* fn::invoke:
* Function: vault:kv:getSecretsListV2
* Arguments:
* mount: ${kvv2.path}
* name: ${test2.name}
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `read` capability on the given path.
* @param argument A collection of arguments for invoking getSecretsListV2.
* @return A collection of values returned by getSecretsListV2.
*/
public suspend fun getSecretsListV2(argument: GetSecretsListV2PlainArgs): GetSecretsListV2Result =
getSecretsListV2ResultToKotlin(getSecretsListV2Plain(argument.toJava()).await())
/**
* @see [getSecretsListV2].
* @param mount Path where KV-V2 engine is mounted.
* @param name Full name of the secret. For a nested secret
* the name is the nested path excluding the mount and data
* prefix. For example, for a secret at `kvv2/data/foo/bar/baz`
* the name is `foo/bar/baz`.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @return A collection of values returned by getSecretsListV2.
*/
public suspend fun getSecretsListV2(
mount: String,
name: String? = null,
namespace: String? = null,
): GetSecretsListV2Result {
val argument = GetSecretsListV2PlainArgs(
mount = mount,
name = name,
namespace = namespace,
)
return getSecretsListV2ResultToKotlin(getSecretsListV2Plain(argument.toJava()).await())
}
/**
* @see [getSecretsListV2].
* @param argument Builder for [com.pulumi.vault.kv.kotlin.inputs.GetSecretsListV2PlainArgs].
* @return A collection of values returned by getSecretsListV2.
*/
public suspend fun getSecretsListV2(argument: suspend GetSecretsListV2PlainArgsBuilder.() -> Unit): GetSecretsListV2Result {
val builder = GetSecretsListV2PlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretsListV2ResultToKotlin(getSecretsListV2Plain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy