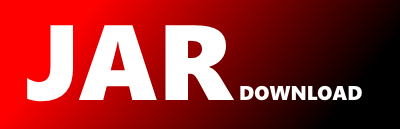
com.pulumi.vault.kv.kotlin.SecretArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.kv.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.kv.SecretArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Writes a KV-V1 secret to a given path in Vault.
* For more information on Vault's KV-V1 secret backend
* [see here](https://www.vaultproject.io/docs/secrets/kv/kv-v1).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const kvv1 = new vault.Mount("kvv1", {
* path: "kvv1",
* type: "kv",
* options: {
* version: "1",
* },
* description: "KV Version 1 secret engine mount",
* });
* const secret = new vault.kv.Secret("secret", {
* path: pulumi.interpolate`${kvv1.path}/secret`,
* dataJson: JSON.stringify({
* zip: "zap",
* foo: "bar",
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_vault as vault
* kvv1 = vault.Mount("kvv1",
* path="kvv1",
* type="kv",
* options={
* "version": "1",
* },
* description="KV Version 1 secret engine mount")
* secret = vault.kv.Secret("secret",
* path=kvv1.path.apply(lambda path: f"{path}/secret"),
* data_json=json.dumps({
* "zip": "zap",
* "foo": "bar",
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var kvv1 = new Vault.Mount("kvv1", new()
* {
* Path = "kvv1",
* Type = "kv",
* Options =
* {
* { "version", "1" },
* },
* Description = "KV Version 1 secret engine mount",
* });
* var secret = new Vault.Kv.Secret("secret", new()
* {
* Path = kvv1.Path.Apply(path => $"{path}/secret"),
* DataJson = JsonSerializer.Serialize(new Dictionary
* {
* ["zip"] = "zap",
* ["foo"] = "bar",
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "fmt"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/kv"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* kvv1, err := vault.NewMount(ctx, "kvv1", &vault.MountArgs{
* Path: pulumi.String("kvv1"),
* Type: pulumi.String("kv"),
* Options: pulumi.Map{
* "version": pulumi.Any("1"),
* },
* Description: pulumi.String("KV Version 1 secret engine mount"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "zip": "zap",
* "foo": "bar",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = kv.NewSecret(ctx, "secret", &kv.SecretArgs{
* Path: kvv1.Path.ApplyT(func(path string) (string, error) {
* return fmt.Sprintf("%v/secret", path), nil
* }).(pulumi.StringOutput),
* DataJson: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.kv.Secret;
* import com.pulumi.vault.kv.SecretArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kvv1 = new Mount("kvv1", MountArgs.builder()
* .path("kvv1")
* .type("kv")
* .options(Map.of("version", "1"))
* .description("KV Version 1 secret engine mount")
* .build());
* var secret = new Secret("secret", SecretArgs.builder()
* .path(kvv1.path().applyValue(path -> String.format("%s/secret", path)))
* .dataJson(serializeJson(
* jsonObject(
* jsonProperty("zip", "zap"),
* jsonProperty("foo", "bar")
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* kvv1:
* type: vault:Mount
* properties:
* path: kvv1
* type: kv
* options:
* version: '1'
* description: KV Version 1 secret engine mount
* secret:
* type: vault:kv:Secret
* properties:
* path: ${kvv1.path}/secret
* dataJson:
* fn::toJSON:
* zip: zap
* foo: bar
* ```
*
* ## Required Vault Capabilities
* Use of this resource requires the `create` or `update` capability
* (depending on whether the resource already exists) on the given path,
* the `delete` capability if the resource is removed from configuration,
* and the `read` capability for drift detection (by default).
* ## Import
* KV-V1 secrets can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:kv/secret:Secret secret kvv1/secret
* ```
* @property dataJson JSON-encoded string that will be
* written as the secret data at the given path.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path Full path of the KV-V1 secret.
*/
public data class SecretArgs(
public val dataJson: Output? = null,
public val namespace: Output? = null,
public val path: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.kv.SecretArgs = com.pulumi.vault.kv.SecretArgs.builder()
.dataJson(dataJson?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretArgs].
*/
@PulumiTagMarker
public class SecretArgsBuilder internal constructor() {
private var dataJson: Output? = null
private var namespace: Output? = null
private var path: Output? = null
/**
* @param value JSON-encoded string that will be
* written as the secret data at the given path.
*/
@JvmName("srwmtvqshwhghwly")
public suspend fun dataJson(`value`: Output) {
this.dataJson = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("hdbdkxshhyjbenaj")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Full path of the KV-V1 secret.
*/
@JvmName("tdocihiduxcysgvv")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value JSON-encoded string that will be
* written as the secret data at the given path.
*/
@JvmName("vtrahlfyxsrktgbi")
public suspend fun dataJson(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataJson = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("gxhgpkbmeihrtfuq")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Full path of the KV-V1 secret.
*/
@JvmName("mhwopawgitqdemix")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
internal fun build(): SecretArgs = SecretArgs(
dataJson = dataJson,
namespace = namespace,
path = path,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy