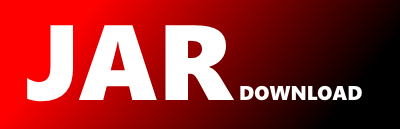
com.pulumi.vault.ldap.kotlin.SecretBackendDynamicRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.ldap.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.ldap.SecretBackendDynamicRoleArgs.builder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const config = new vault.ldap.SecretBackend("config", {
* path: "my-custom-ldap",
* binddn: "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass: "SuperSecretPassw0rd",
* url: "ldaps://localhost",
* userdn: "CN=Users,DC=corp,DC=example,DC=net",
* });
* const role = new vault.ldap.SecretBackendDynamicRole("role", {
* mount: config.path,
* roleName: "alice",
* creationLdif: `dn: cn={{.Username}},ou=users,dc=learn,dc=example
* objectClass: person
* objectClass: top
* cn: learn
* sn: {{.Password | utf16le | base64}}
* memberOf: cn=dev,ou=groups,dc=learn,dc=example
* userPassword: {{.Password}}
* `,
* deletionLdif: `dn: cn={{.Username}},ou=users,dc=learn,dc=example
* changetype: delete
* rollback_ldif = <
* {
* var config = new Vault.Ldap.SecretBackend("config", new()
* {
* Path = "my-custom-ldap",
* Binddn = "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* Bindpass = "SuperSecretPassw0rd",
* Url = "ldaps://localhost",
* Userdn = "CN=Users,DC=corp,DC=example,DC=net",
* });
* var role = new Vault.Ldap.SecretBackendDynamicRole("role", new()
* {
* Mount = config.Path,
* RoleName = "alice",
* CreationLdif = @"dn: cn={{.Username}},ou=users,dc=learn,dc=example
* objectClass: person
* objectClass: top
* cn: learn
* sn: {{.Password | utf16le | base64}}
* memberOf: cn=dev,ou=groups,dc=learn,dc=example
* userPassword: {{.Password}}
* ",
* DeletionLdif = @"dn: cn={{.Username}},ou=users,dc=learn,dc=example
* changetype: delete
* rollback_ldif = <
* ## Import
* LDAP secret backend dynamic role can be imported using the full path to the role
* of the form: `/dynamic-role/` e.g.
* ```sh
* $ pulumi import vault:ldap/secretBackendDynamicRole:SecretBackendDynamicRole role ldap/role/dynamic-role
* ```
* @property creationLdif A templatized LDIF string used to create a user
* account. This may contain multiple LDIF entries. The `creation_ldif` can also
* be used to add the user account to an existing group. All LDIF entries are
* performed in order. If Vault encounters an error while executing the
* `creation_ldif` it will stop at the first error and not execute any remaining
* LDIF entries. If an error occurs and `rollback_ldif` is specified, the LDIF
* entries in `rollback_ldif` will be executed. See `rollback_ldif` for more
* details. This field may optionally be provided as a base64 encoded string.
* @property defaultTtl Specifies the TTL for the leases associated with this role.
* @property deletionLdif A templatized LDIF string used to delete the
* user account once its TTL has expired. This may contain multiple LDIF
* entries. All LDIF entries are performed in order. If Vault encounters an
* error while executing an entry in the `deletion_ldif` it will attempt to
* continue executing any remaining entries. This field may optionally be
* provided as a base64 encoded string.
* @property maxTtl Specifies the maximum TTL for the leases associated with this role.
* @property mount The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ldap`.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property roleName Name of the role.
* @property rollbackLdif A templatized LDIF string used to attempt to
* rollback any changes in the event that execution of the `creation_ldif` results
* in an error. This may contain multiple LDIF entries. All LDIF entries are
* performed in order. If Vault encounters an error while executing an entry in
* the `rollback_ldif` it will attempt to continue executing any remaining
* entries. This field may optionally be provided as a base64 encoded string.
* @property usernameTemplate A template used to generate a dynamic
* username. This will be used to fill in the `.Username` field within the
* `creation_ldif` string.
*/
public data class SecretBackendDynamicRoleArgs(
public val creationLdif: Output? = null,
public val defaultTtl: Output? = null,
public val deletionLdif: Output? = null,
public val maxTtl: Output? = null,
public val mount: Output? = null,
public val namespace: Output? = null,
public val roleName: Output? = null,
public val rollbackLdif: Output? = null,
public val usernameTemplate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.ldap.SecretBackendDynamicRoleArgs =
com.pulumi.vault.ldap.SecretBackendDynamicRoleArgs.builder()
.creationLdif(creationLdif?.applyValue({ args0 -> args0 }))
.defaultTtl(defaultTtl?.applyValue({ args0 -> args0 }))
.deletionLdif(deletionLdif?.applyValue({ args0 -> args0 }))
.maxTtl(maxTtl?.applyValue({ args0 -> args0 }))
.mount(mount?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.roleName(roleName?.applyValue({ args0 -> args0 }))
.rollbackLdif(rollbackLdif?.applyValue({ args0 -> args0 }))
.usernameTemplate(usernameTemplate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendDynamicRoleArgs].
*/
@PulumiTagMarker
public class SecretBackendDynamicRoleArgsBuilder internal constructor() {
private var creationLdif: Output? = null
private var defaultTtl: Output? = null
private var deletionLdif: Output? = null
private var maxTtl: Output? = null
private var mount: Output? = null
private var namespace: Output? = null
private var roleName: Output? = null
private var rollbackLdif: Output? = null
private var usernameTemplate: Output? = null
/**
* @param value A templatized LDIF string used to create a user
* account. This may contain multiple LDIF entries. The `creation_ldif` can also
* be used to add the user account to an existing group. All LDIF entries are
* performed in order. If Vault encounters an error while executing the
* `creation_ldif` it will stop at the first error and not execute any remaining
* LDIF entries. If an error occurs and `rollback_ldif` is specified, the LDIF
* entries in `rollback_ldif` will be executed. See `rollback_ldif` for more
* details. This field may optionally be provided as a base64 encoded string.
*/
@JvmName("crgrbxjjrywmmwgp")
public suspend fun creationLdif(`value`: Output) {
this.creationLdif = value
}
/**
* @param value Specifies the TTL for the leases associated with this role.
*/
@JvmName("nuyocdbyrfwdtefr")
public suspend fun defaultTtl(`value`: Output) {
this.defaultTtl = value
}
/**
* @param value A templatized LDIF string used to delete the
* user account once its TTL has expired. This may contain multiple LDIF
* entries. All LDIF entries are performed in order. If Vault encounters an
* error while executing an entry in the `deletion_ldif` it will attempt to
* continue executing any remaining entries. This field may optionally be
* provided as a base64 encoded string.
*/
@JvmName("aavwxxjfhueqoxyx")
public suspend fun deletionLdif(`value`: Output) {
this.deletionLdif = value
}
/**
* @param value Specifies the maximum TTL for the leases associated with this role.
*/
@JvmName("rbhnqxbwtpymriru")
public suspend fun maxTtl(`value`: Output) {
this.maxTtl = value
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ldap`.
*/
@JvmName("xxbuthweccujlsqg")
public suspend fun mount(`value`: Output) {
this.mount = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("nkelrctqfeipsgxc")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Name of the role.
*/
@JvmName("bmnswusoxkrolhwg")
public suspend fun roleName(`value`: Output) {
this.roleName = value
}
/**
* @param value A templatized LDIF string used to attempt to
* rollback any changes in the event that execution of the `creation_ldif` results
* in an error. This may contain multiple LDIF entries. All LDIF entries are
* performed in order. If Vault encounters an error while executing an entry in
* the `rollback_ldif` it will attempt to continue executing any remaining
* entries. This field may optionally be provided as a base64 encoded string.
*/
@JvmName("tokgrogjlurkcwke")
public suspend fun rollbackLdif(`value`: Output) {
this.rollbackLdif = value
}
/**
* @param value A template used to generate a dynamic
* username. This will be used to fill in the `.Username` field within the
* `creation_ldif` string.
*/
@JvmName("nebeqdigequyxnvo")
public suspend fun usernameTemplate(`value`: Output) {
this.usernameTemplate = value
}
/**
* @param value A templatized LDIF string used to create a user
* account. This may contain multiple LDIF entries. The `creation_ldif` can also
* be used to add the user account to an existing group. All LDIF entries are
* performed in order. If Vault encounters an error while executing the
* `creation_ldif` it will stop at the first error and not execute any remaining
* LDIF entries. If an error occurs and `rollback_ldif` is specified, the LDIF
* entries in `rollback_ldif` will be executed. See `rollback_ldif` for more
* details. This field may optionally be provided as a base64 encoded string.
*/
@JvmName("efogrtpvyttmprsi")
public suspend fun creationLdif(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.creationLdif = mapped
}
/**
* @param value Specifies the TTL for the leases associated with this role.
*/
@JvmName("nxsqnyxabhmbhujj")
public suspend fun defaultTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultTtl = mapped
}
/**
* @param value A templatized LDIF string used to delete the
* user account once its TTL has expired. This may contain multiple LDIF
* entries. All LDIF entries are performed in order. If Vault encounters an
* error while executing an entry in the `deletion_ldif` it will attempt to
* continue executing any remaining entries. This field may optionally be
* provided as a base64 encoded string.
*/
@JvmName("jqdpirqmagrpiknh")
public suspend fun deletionLdif(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deletionLdif = mapped
}
/**
* @param value Specifies the maximum TTL for the leases associated with this role.
*/
@JvmName("thpfkpkruigerlkf")
public suspend fun maxTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxTtl = mapped
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ldap`.
*/
@JvmName("nxxtqqddyuqiyddi")
public suspend fun mount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mount = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("snsneifqqlnvnpgo")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Name of the role.
*/
@JvmName("qkjshuyhqmgjbdmb")
public suspend fun roleName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.roleName = mapped
}
/**
* @param value A templatized LDIF string used to attempt to
* rollback any changes in the event that execution of the `creation_ldif` results
* in an error. This may contain multiple LDIF entries. All LDIF entries are
* performed in order. If Vault encounters an error while executing an entry in
* the `rollback_ldif` it will attempt to continue executing any remaining
* entries. This field may optionally be provided as a base64 encoded string.
*/
@JvmName("byrkxvppixqcakiq")
public suspend fun rollbackLdif(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rollbackLdif = mapped
}
/**
* @param value A template used to generate a dynamic
* username. This will be used to fill in the `.Username` field within the
* `creation_ldif` string.
*/
@JvmName("gvdxblvafpchgpey")
public suspend fun usernameTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameTemplate = mapped
}
internal fun build(): SecretBackendDynamicRoleArgs = SecretBackendDynamicRoleArgs(
creationLdif = creationLdif,
defaultTtl = defaultTtl,
deletionLdif = deletionLdif,
maxTtl = maxTtl,
mount = mount,
namespace = namespace,
roleName = roleName,
rollbackLdif = rollbackLdif,
usernameTemplate = usernameTemplate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy