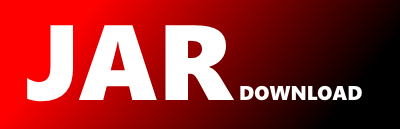
com.pulumi.vault.ldap.kotlin.SecretBackendLibrarySet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.ldap.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [SecretBackendLibrarySet].
*/
@PulumiTagMarker
public class SecretBackendLibrarySetResourceBuilder internal constructor() {
public var name: String? = null
public var args: SecretBackendLibrarySetArgs = SecretBackendLibrarySetArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SecretBackendLibrarySetArgsBuilder.() -> Unit) {
val builder = SecretBackendLibrarySetArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SecretBackendLibrarySet {
val builtJavaResource = com.pulumi.vault.ldap.SecretBackendLibrarySet(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SecretBackendLibrarySet(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const config = new vault.ldap.SecretBackend("config", {
* path: "ldap",
* binddn: "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass: "SuperSecretPassw0rd",
* url: "ldaps://localhost",
* insecureTls: true,
* userdn: "CN=Users,DC=corp,DC=example,DC=net",
* });
* const qa = new vault.ldap.SecretBackendLibrarySet("qa", {
* mount: config.path,
* name: "qa",
* serviceAccountNames: [
* "Bob",
* "Mary",
* ],
* ttl: 60,
* disableCheckInEnforcement: true,
* maxTtl: 120,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* config = vault.ldap.SecretBackend("config",
* path="ldap",
* binddn="CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass="SuperSecretPassw0rd",
* url="ldaps://localhost",
* insecure_tls=True,
* userdn="CN=Users,DC=corp,DC=example,DC=net")
* qa = vault.ldap.SecretBackendLibrarySet("qa",
* mount=config.path,
* name="qa",
* service_account_names=[
* "Bob",
* "Mary",
* ],
* ttl=60,
* disable_check_in_enforcement=True,
* max_ttl=120)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var config = new Vault.Ldap.SecretBackend("config", new()
* {
* Path = "ldap",
* Binddn = "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* Bindpass = "SuperSecretPassw0rd",
* Url = "ldaps://localhost",
* InsecureTls = true,
* Userdn = "CN=Users,DC=corp,DC=example,DC=net",
* });
* var qa = new Vault.Ldap.SecretBackendLibrarySet("qa", new()
* {
* Mount = config.Path,
* Name = "qa",
* ServiceAccountNames = new[]
* {
* "Bob",
* "Mary",
* },
* Ttl = 60,
* DisableCheckInEnforcement = true,
* MaxTtl = 120,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/ldap"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* config, err := ldap.NewSecretBackend(ctx, "config", &ldap.SecretBackendArgs{
* Path: pulumi.String("ldap"),
* Binddn: pulumi.String("CN=Administrator,CN=Users,DC=corp,DC=example,DC=net"),
* Bindpass: pulumi.String("SuperSecretPassw0rd"),
* Url: pulumi.String("ldaps://localhost"),
* InsecureTls: pulumi.Bool(true),
* Userdn: pulumi.String("CN=Users,DC=corp,DC=example,DC=net"),
* })
* if err != nil {
* return err
* }
* _, err = ldap.NewSecretBackendLibrarySet(ctx, "qa", &ldap.SecretBackendLibrarySetArgs{
* Mount: config.Path,
* Name: pulumi.String("qa"),
* ServiceAccountNames: pulumi.StringArray{
* pulumi.String("Bob"),
* pulumi.String("Mary"),
* },
* Ttl: pulumi.Int(60),
* DisableCheckInEnforcement: pulumi.Bool(true),
* MaxTtl: pulumi.Int(120),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.ldap.SecretBackend;
* import com.pulumi.vault.ldap.SecretBackendArgs;
* import com.pulumi.vault.ldap.SecretBackendLibrarySet;
* import com.pulumi.vault.ldap.SecretBackendLibrarySetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var config = new SecretBackend("config", SecretBackendArgs.builder()
* .path("ldap")
* .binddn("CN=Administrator,CN=Users,DC=corp,DC=example,DC=net")
* .bindpass("SuperSecretPassw0rd")
* .url("ldaps://localhost")
* .insecureTls("true")
* .userdn("CN=Users,DC=corp,DC=example,DC=net")
* .build());
* var qa = new SecretBackendLibrarySet("qa", SecretBackendLibrarySetArgs.builder()
* .mount(config.path())
* .name("qa")
* .serviceAccountNames(
* "Bob",
* "Mary")
* .ttl(60)
* .disableCheckInEnforcement(true)
* .maxTtl(120)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* config:
* type: vault:ldap:SecretBackend
* properties:
* path: ldap
* binddn: CN=Administrator,CN=Users,DC=corp,DC=example,DC=net
* bindpass: SuperSecretPassw0rd
* url: ldaps://localhost
* insecureTls: 'true'
* userdn: CN=Users,DC=corp,DC=example,DC=net
* qa:
* type: vault:ldap:SecretBackendLibrarySet
* properties:
* mount: ${config.path}
* name: qa
* serviceAccountNames:
* - Bob
* - Mary
* ttl: 60
* disableCheckInEnforcement: true
* maxTtl: 120
* ```
*
* ## Import
* LDAP secret backend libraries can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:ldap/secretBackendLibrarySet:SecretBackendLibrarySet qa ldap/library/bob
* ```
*/
public class SecretBackendLibrarySet internal constructor(
override val javaResource: com.pulumi.vault.ldap.SecretBackendLibrarySet,
) : KotlinCustomResource(javaResource, SecretBackendLibrarySetMapper) {
/**
* Disable enforcing that service
* accounts must be checked in by the entity or client token that checked them
* out. Defaults to false.
*/
public val disableCheckInEnforcement: Output?
get() = javaResource.disableCheckInEnforcement().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum password time-to-live in seconds. Defaults
* to the configuration max_ttl if not provided.
*/
public val maxTtl: Output
get() = javaResource.maxTtl().applyValue({ args0 -> args0 })
/**
* The path where the LDAP secrets backend is mounted.
*/
public val mount: Output?
get() = javaResource.mount().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The name to identify this set of service accounts.
* Must be unique within the backend.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies the slice of service accounts mapped to this set.
*/
public val serviceAccountNames: Output>
get() = javaResource.serviceAccountNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The password time-to-live in seconds. Defaults to the configuration
* ttl if not provided.
*/
public val ttl: Output
get() = javaResource.ttl().applyValue({ args0 -> args0 })
}
public object SecretBackendLibrarySetMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.ldap.SecretBackendLibrarySet::class == javaResource::class
override fun map(javaResource: Resource): SecretBackendLibrarySet =
SecretBackendLibrarySet(javaResource as com.pulumi.vault.ldap.SecretBackendLibrarySet)
}
/**
* @see [SecretBackendLibrarySet].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [SecretBackendLibrarySet].
*/
public suspend fun secretBackendLibrarySet(
name: String,
block: suspend SecretBackendLibrarySetResourceBuilder.() -> Unit,
): SecretBackendLibrarySet {
val builder = SecretBackendLibrarySetResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [SecretBackendLibrarySet].
* @param name The _unique_ name of the resulting resource.
*/
public fun secretBackendLibrarySet(name: String): SecretBackendLibrarySet {
val builder = SecretBackendLibrarySetResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy