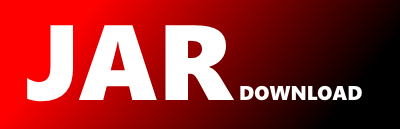
com.pulumi.vault.ldap.kotlin.SecretBackendStaticRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.ldap.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.ldap.SecretBackendStaticRoleArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const config = new vault.ldap.SecretBackend("config", {
* path: "my-custom-ldap",
* binddn: "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass: "SuperSecretPassw0rd",
* url: "ldaps://localhost",
* insecureTls: true,
* userdn: "CN=Users,DC=corp,DC=example,DC=net",
* });
* const role = new vault.ldap.SecretBackendStaticRole("role", {
* mount: config.path,
* username: "alice",
* dn: "cn=alice,ou=Users,DC=corp,DC=example,DC=net",
* roleName: "alice",
* rotationPeriod: 60,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* config = vault.ldap.SecretBackend("config",
* path="my-custom-ldap",
* binddn="CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass="SuperSecretPassw0rd",
* url="ldaps://localhost",
* insecure_tls=True,
* userdn="CN=Users,DC=corp,DC=example,DC=net")
* role = vault.ldap.SecretBackendStaticRole("role",
* mount=config.path,
* username="alice",
* dn="cn=alice,ou=Users,DC=corp,DC=example,DC=net",
* role_name="alice",
* rotation_period=60)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var config = new Vault.Ldap.SecretBackend("config", new()
* {
* Path = "my-custom-ldap",
* Binddn = "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* Bindpass = "SuperSecretPassw0rd",
* Url = "ldaps://localhost",
* InsecureTls = true,
* Userdn = "CN=Users,DC=corp,DC=example,DC=net",
* });
* var role = new Vault.Ldap.SecretBackendStaticRole("role", new()
* {
* Mount = config.Path,
* Username = "alice",
* Dn = "cn=alice,ou=Users,DC=corp,DC=example,DC=net",
* RoleName = "alice",
* RotationPeriod = 60,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/ldap"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* config, err := ldap.NewSecretBackend(ctx, "config", &ldap.SecretBackendArgs{
* Path: pulumi.String("my-custom-ldap"),
* Binddn: pulumi.String("CN=Administrator,CN=Users,DC=corp,DC=example,DC=net"),
* Bindpass: pulumi.String("SuperSecretPassw0rd"),
* Url: pulumi.String("ldaps://localhost"),
* InsecureTls: pulumi.Bool(true),
* Userdn: pulumi.String("CN=Users,DC=corp,DC=example,DC=net"),
* })
* if err != nil {
* return err
* }
* _, err = ldap.NewSecretBackendStaticRole(ctx, "role", &ldap.SecretBackendStaticRoleArgs{
* Mount: config.Path,
* Username: pulumi.String("alice"),
* Dn: pulumi.String("cn=alice,ou=Users,DC=corp,DC=example,DC=net"),
* RoleName: pulumi.String("alice"),
* RotationPeriod: pulumi.Int(60),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.ldap.SecretBackend;
* import com.pulumi.vault.ldap.SecretBackendArgs;
* import com.pulumi.vault.ldap.SecretBackendStaticRole;
* import com.pulumi.vault.ldap.SecretBackendStaticRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var config = new SecretBackend("config", SecretBackendArgs.builder()
* .path("my-custom-ldap")
* .binddn("CN=Administrator,CN=Users,DC=corp,DC=example,DC=net")
* .bindpass("SuperSecretPassw0rd")
* .url("ldaps://localhost")
* .insecureTls("true")
* .userdn("CN=Users,DC=corp,DC=example,DC=net")
* .build());
* var role = new SecretBackendStaticRole("role", SecretBackendStaticRoleArgs.builder()
* .mount(config.path())
* .username("alice")
* .dn("cn=alice,ou=Users,DC=corp,DC=example,DC=net")
* .roleName("alice")
* .rotationPeriod(60)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* config:
* type: vault:ldap:SecretBackend
* properties:
* path: my-custom-ldap
* binddn: CN=Administrator,CN=Users,DC=corp,DC=example,DC=net
* bindpass: SuperSecretPassw0rd
* url: ldaps://localhost
* insecureTls: 'true'
* userdn: CN=Users,DC=corp,DC=example,DC=net
* role:
* type: vault:ldap:SecretBackendStaticRole
* properties:
* mount: ${config.path}
* username: alice
* dn: cn=alice,ou=Users,DC=corp,DC=example,DC=net
* roleName: alice
* rotationPeriod: 60
* ```
*
* ## Import
* LDAP secret backend static role can be imported using the full path to the role
* of the form: `/static-role/` e.g.
* ```sh
* $ pulumi import vault:ldap/secretBackendStaticRole:SecretBackendStaticRole role ldap/static-role/example-role
* ```
* @property dn Distinguished name (DN) of the existing LDAP entry to manage
* password rotation for. If given, it will take precedence over `username` for the LDAP
* search performed during password rotation. Cannot be modified after creation.
* @property mount The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ldap`.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property roleName Name of the role.
* @property rotationPeriod How often Vault should rotate the password of the user entry.
* @property skipImportRotation Causes vault to skip the initial secret rotation on import. Not applicable to updates.
* Requires Vault 1.16 or above.
* @property username The username of the existing LDAP entry to manage password rotation for.
*/
public data class SecretBackendStaticRoleArgs(
public val dn: Output? = null,
public val mount: Output? = null,
public val namespace: Output? = null,
public val roleName: Output? = null,
public val rotationPeriod: Output? = null,
public val skipImportRotation: Output? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.ldap.SecretBackendStaticRoleArgs =
com.pulumi.vault.ldap.SecretBackendStaticRoleArgs.builder()
.dn(dn?.applyValue({ args0 -> args0 }))
.mount(mount?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.roleName(roleName?.applyValue({ args0 -> args0 }))
.rotationPeriod(rotationPeriod?.applyValue({ args0 -> args0 }))
.skipImportRotation(skipImportRotation?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendStaticRoleArgs].
*/
@PulumiTagMarker
public class SecretBackendStaticRoleArgsBuilder internal constructor() {
private var dn: Output? = null
private var mount: Output? = null
private var namespace: Output? = null
private var roleName: Output? = null
private var rotationPeriod: Output? = null
private var skipImportRotation: Output? = null
private var username: Output? = null
/**
* @param value Distinguished name (DN) of the existing LDAP entry to manage
* password rotation for. If given, it will take precedence over `username` for the LDAP
* search performed during password rotation. Cannot be modified after creation.
*/
@JvmName("nookxgxjakdemrba")
public suspend fun dn(`value`: Output) {
this.dn = value
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ldap`.
*/
@JvmName("lpdlxxlecabwdgey")
public suspend fun mount(`value`: Output) {
this.mount = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("fgeghnnorvgvcayy")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Name of the role.
*/
@JvmName("gotolpqoqmhnphna")
public suspend fun roleName(`value`: Output) {
this.roleName = value
}
/**
* @param value How often Vault should rotate the password of the user entry.
*/
@JvmName("cvqglqjkvtvfidht")
public suspend fun rotationPeriod(`value`: Output) {
this.rotationPeriod = value
}
/**
* @param value Causes vault to skip the initial secret rotation on import. Not applicable to updates.
* Requires Vault 1.16 or above.
*/
@JvmName("fqddcmcvxludupna")
public suspend fun skipImportRotation(`value`: Output) {
this.skipImportRotation = value
}
/**
* @param value The username of the existing LDAP entry to manage password rotation for.
*/
@JvmName("lsrjddydyseosdbp")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Distinguished name (DN) of the existing LDAP entry to manage
* password rotation for. If given, it will take precedence over `username` for the LDAP
* search performed during password rotation. Cannot be modified after creation.
*/
@JvmName("nhhmwkigcfjofcln")
public suspend fun dn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dn = mapped
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ldap`.
*/
@JvmName("pdqgwavvecsevrrx")
public suspend fun mount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mount = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("roaqgfyhoerfihum")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Name of the role.
*/
@JvmName("munrasmxcrvafkap")
public suspend fun roleName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.roleName = mapped
}
/**
* @param value How often Vault should rotate the password of the user entry.
*/
@JvmName("fadlvqdxjsvkjrdh")
public suspend fun rotationPeriod(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rotationPeriod = mapped
}
/**
* @param value Causes vault to skip the initial secret rotation on import. Not applicable to updates.
* Requires Vault 1.16 or above.
*/
@JvmName("havxomjmbmwhsaji")
public suspend fun skipImportRotation(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skipImportRotation = mapped
}
/**
* @param value The username of the existing LDAP entry to manage password rotation for.
*/
@JvmName("egyytxjvyyxpiesn")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): SecretBackendStaticRoleArgs = SecretBackendStaticRoleArgs(
dn = dn,
mount = mount,
namespace = namespace,
roleName = roleName,
rotationPeriod = rotationPeriod,
skipImportRotation = skipImportRotation,
username = username,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy