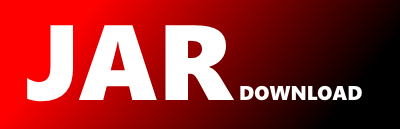
com.pulumi.vault.managed.kotlin.inputs.KeysAzureArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.managed.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.managed.inputs.KeysAzureArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property allowGenerateKey If no existing key can be found in the referenced backend, instructs Vault to generate a key within the backend
* @property allowReplaceKey Controls the ability for Vault to replace through generation or importing a key into the configured backend even if a key is present, if set to false those operations are forbidden if a key exists.
* @property allowStoreKey Controls the ability for Vault to import a key to the configured backend, if 'false', those operations will be forbidden
* @property anyMount Allow usage from any mount point within the namespace if 'true'
* @property clientId The client id for credentials to query the Azure APIs
* @property clientSecret The client secret for credentials to query the Azure APIs
* @property environment The Azure Cloud environment API endpoints to use
* @property keyBits The size in bits for an RSA key. This field is required when 'key_type' is 'RSA' or when 'allow_generate_key' is true
* @property keyName The Key Vault key to use for encryption and decryption
* @property keyType The type of key to use
* @property name A unique lowercase name that serves as identifying the key
* @property resource The Azure Key Vault resource's DNS Suffix to connect to
* @property tenantId The tenant id for the Azure Active Directory organization
* @property uuid ID of the managed key read from Vault
* @property vaultName The Key Vault vault to use the encryption keys for encryption and decryption
*/
public data class KeysAzureArgs(
public val allowGenerateKey: Output? = null,
public val allowReplaceKey: Output? = null,
public val allowStoreKey: Output? = null,
public val anyMount: Output? = null,
public val clientId: Output,
public val clientSecret: Output,
public val environment: Output? = null,
public val keyBits: Output? = null,
public val keyName: Output,
public val keyType: Output,
public val name: Output,
public val resource: Output? = null,
public val tenantId: Output,
public val uuid: Output? = null,
public val vaultName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.managed.inputs.KeysAzureArgs =
com.pulumi.vault.managed.inputs.KeysAzureArgs.builder()
.allowGenerateKey(allowGenerateKey?.applyValue({ args0 -> args0 }))
.allowReplaceKey(allowReplaceKey?.applyValue({ args0 -> args0 }))
.allowStoreKey(allowStoreKey?.applyValue({ args0 -> args0 }))
.anyMount(anyMount?.applyValue({ args0 -> args0 }))
.clientId(clientId.applyValue({ args0 -> args0 }))
.clientSecret(clientSecret.applyValue({ args0 -> args0 }))
.environment(environment?.applyValue({ args0 -> args0 }))
.keyBits(keyBits?.applyValue({ args0 -> args0 }))
.keyName(keyName.applyValue({ args0 -> args0 }))
.keyType(keyType.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.resource(resource?.applyValue({ args0 -> args0 }))
.tenantId(tenantId.applyValue({ args0 -> args0 }))
.uuid(uuid?.applyValue({ args0 -> args0 }))
.vaultName(vaultName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KeysAzureArgs].
*/
@PulumiTagMarker
public class KeysAzureArgsBuilder internal constructor() {
private var allowGenerateKey: Output? = null
private var allowReplaceKey: Output? = null
private var allowStoreKey: Output? = null
private var anyMount: Output? = null
private var clientId: Output? = null
private var clientSecret: Output? = null
private var environment: Output? = null
private var keyBits: Output? = null
private var keyName: Output? = null
private var keyType: Output? = null
private var name: Output? = null
private var resource: Output? = null
private var tenantId: Output? = null
private var uuid: Output? = null
private var vaultName: Output? = null
/**
* @param value If no existing key can be found in the referenced backend, instructs Vault to generate a key within the backend
*/
@JvmName("njwjfpipnhwlhrov")
public suspend fun allowGenerateKey(`value`: Output) {
this.allowGenerateKey = value
}
/**
* @param value Controls the ability for Vault to replace through generation or importing a key into the configured backend even if a key is present, if set to false those operations are forbidden if a key exists.
*/
@JvmName("uwwpkqgaahprrmbc")
public suspend fun allowReplaceKey(`value`: Output) {
this.allowReplaceKey = value
}
/**
* @param value Controls the ability for Vault to import a key to the configured backend, if 'false', those operations will be forbidden
*/
@JvmName("cpjqyrmtyifxynvd")
public suspend fun allowStoreKey(`value`: Output) {
this.allowStoreKey = value
}
/**
* @param value Allow usage from any mount point within the namespace if 'true'
*/
@JvmName("oswbuukwqbykvdfx")
public suspend fun anyMount(`value`: Output) {
this.anyMount = value
}
/**
* @param value The client id for credentials to query the Azure APIs
*/
@JvmName("fqeadtusktgwoges")
public suspend fun clientId(`value`: Output) {
this.clientId = value
}
/**
* @param value The client secret for credentials to query the Azure APIs
*/
@JvmName("mbchnahurirxwejo")
public suspend fun clientSecret(`value`: Output) {
this.clientSecret = value
}
/**
* @param value The Azure Cloud environment API endpoints to use
*/
@JvmName("tjpgmfrgspcsvjuh")
public suspend fun environment(`value`: Output) {
this.environment = value
}
/**
* @param value The size in bits for an RSA key. This field is required when 'key_type' is 'RSA' or when 'allow_generate_key' is true
*/
@JvmName("tcwbodvmxbbbwjjp")
public suspend fun keyBits(`value`: Output) {
this.keyBits = value
}
/**
* @param value The Key Vault key to use for encryption and decryption
*/
@JvmName("nspypksjlurwkhro")
public suspend fun keyName(`value`: Output) {
this.keyName = value
}
/**
* @param value The type of key to use
*/
@JvmName("ebogybrdeyxlfwgv")
public suspend fun keyType(`value`: Output) {
this.keyType = value
}
/**
* @param value A unique lowercase name that serves as identifying the key
*/
@JvmName("pjkuypiavtwbfmee")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The Azure Key Vault resource's DNS Suffix to connect to
*/
@JvmName("mjmafhhhbmuxvuhp")
public suspend fun resource(`value`: Output) {
this.resource = value
}
/**
* @param value The tenant id for the Azure Active Directory organization
*/
@JvmName("hsriavcykylappjh")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value ID of the managed key read from Vault
*/
@JvmName("nttoqdlfspirbjxx")
public suspend fun uuid(`value`: Output) {
this.uuid = value
}
/**
* @param value The Key Vault vault to use the encryption keys for encryption and decryption
*/
@JvmName("xebwqtjsyibtljrg")
public suspend fun vaultName(`value`: Output) {
this.vaultName = value
}
/**
* @param value If no existing key can be found in the referenced backend, instructs Vault to generate a key within the backend
*/
@JvmName("qkmjapgdpygpfcgv")
public suspend fun allowGenerateKey(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowGenerateKey = mapped
}
/**
* @param value Controls the ability for Vault to replace through generation or importing a key into the configured backend even if a key is present, if set to false those operations are forbidden if a key exists.
*/
@JvmName("cxlpmyrgagajhkvs")
public suspend fun allowReplaceKey(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowReplaceKey = mapped
}
/**
* @param value Controls the ability for Vault to import a key to the configured backend, if 'false', those operations will be forbidden
*/
@JvmName("bjaiwgullbcfjwmm")
public suspend fun allowStoreKey(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowStoreKey = mapped
}
/**
* @param value Allow usage from any mount point within the namespace if 'true'
*/
@JvmName("eresmpqfhjvnrrru")
public suspend fun anyMount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.anyMount = mapped
}
/**
* @param value The client id for credentials to query the Azure APIs
*/
@JvmName("mhebbsounmvrrppe")
public suspend fun clientId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientId = mapped
}
/**
* @param value The client secret for credentials to query the Azure APIs
*/
@JvmName("qwufinwhsvbibxoq")
public suspend fun clientSecret(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientSecret = mapped
}
/**
* @param value The Azure Cloud environment API endpoints to use
*/
@JvmName("eodnngqfpygpnvsn")
public suspend fun environment(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.environment = mapped
}
/**
* @param value The size in bits for an RSA key. This field is required when 'key_type' is 'RSA' or when 'allow_generate_key' is true
*/
@JvmName("hjkxrxtwstsfjnqq")
public suspend fun keyBits(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyBits = mapped
}
/**
* @param value The Key Vault key to use for encryption and decryption
*/
@JvmName("ripdoveptspguupb")
public suspend fun keyName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyName = mapped
}
/**
* @param value The type of key to use
*/
@JvmName("yvbwhwpkwfmixmbr")
public suspend fun keyType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyType = mapped
}
/**
* @param value A unique lowercase name that serves as identifying the key
*/
@JvmName("mhfdivmymuxyhjqv")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The Azure Key Vault resource's DNS Suffix to connect to
*/
@JvmName("enxrjgogqjakbxxc")
public suspend fun resource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resource = mapped
}
/**
* @param value The tenant id for the Azure Active Directory organization
*/
@JvmName("vvcwhgyvitufqllq")
public suspend fun tenantId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tenantId = mapped
}
/**
* @param value ID of the managed key read from Vault
*/
@JvmName("efdboyovslebewsu")
public suspend fun uuid(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uuid = mapped
}
/**
* @param value The Key Vault vault to use the encryption keys for encryption and decryption
*/
@JvmName("xhiedjcbhmulbddj")
public suspend fun vaultName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.vaultName = mapped
}
internal fun build(): KeysAzureArgs = KeysAzureArgs(
allowGenerateKey = allowGenerateKey,
allowReplaceKey = allowReplaceKey,
allowStoreKey = allowStoreKey,
anyMount = anyMount,
clientId = clientId ?: throw PulumiNullFieldException("clientId"),
clientSecret = clientSecret ?: throw PulumiNullFieldException("clientSecret"),
environment = environment,
keyBits = keyBits,
keyName = keyName ?: throw PulumiNullFieldException("keyName"),
keyType = keyType ?: throw PulumiNullFieldException("keyType"),
name = name ?: throw PulumiNullFieldException("name"),
resource = resource,
tenantId = tenantId ?: throw PulumiNullFieldException("tenantId"),
uuid = uuid,
vaultName = vaultName ?: throw PulumiNullFieldException("vaultName"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy