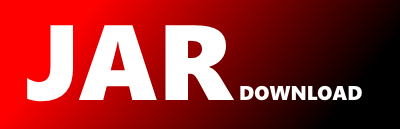
com.pulumi.vault.mongodbatlas.kotlin.SecretBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.mongodbatlas.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.mongodbatlas.SecretBackendArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const mongo = new vault.Mount("mongo", {
* path: "mongodbatlas",
* type: "mongodbatlas",
* description: "MongoDB Atlas secret engine mount",
* });
* const config = new vault.mongodbatlas.SecretBackend("config", {
* mount: mongo.path,
* privateKey: "privateKey",
* publicKey: "publicKey",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* mongo = vault.Mount("mongo",
* path="mongodbatlas",
* type="mongodbatlas",
* description="MongoDB Atlas secret engine mount")
* config = vault.mongodbatlas.SecretBackend("config",
* mount=mongo.path,
* private_key="privateKey",
* public_key="publicKey")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var mongo = new Vault.Mount("mongo", new()
* {
* Path = "mongodbatlas",
* Type = "mongodbatlas",
* Description = "MongoDB Atlas secret engine mount",
* });
* var config = new Vault.MongoDBAtlas.SecretBackend("config", new()
* {
* Mount = mongo.Path,
* PrivateKey = "privateKey",
* PublicKey = "publicKey",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/mongodbatlas"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* mongo, err := vault.NewMount(ctx, "mongo", &vault.MountArgs{
* Path: pulumi.String("mongodbatlas"),
* Type: pulumi.String("mongodbatlas"),
* Description: pulumi.String("MongoDB Atlas secret engine mount"),
* })
* if err != nil {
* return err
* }
* _, err = mongodbatlas.NewSecretBackend(ctx, "config", &mongodbatlas.SecretBackendArgs{
* Mount: mongo.Path,
* PrivateKey: pulumi.String("privateKey"),
* PublicKey: pulumi.String("publicKey"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.mongodbatlas.SecretBackend;
* import com.pulumi.vault.mongodbatlas.SecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var mongo = new Mount("mongo", MountArgs.builder()
* .path("mongodbatlas")
* .type("mongodbatlas")
* .description("MongoDB Atlas secret engine mount")
* .build());
* var config = new SecretBackend("config", SecretBackendArgs.builder()
* .mount(mongo.path())
* .privateKey("privateKey")
* .publicKey("publicKey")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* mongo:
* type: vault:Mount
* properties:
* path: mongodbatlas
* type: mongodbatlas
* description: MongoDB Atlas secret engine mount
* config:
* type: vault:mongodbatlas:SecretBackend
* properties:
* mount: ${mongo.path}
* privateKey: privateKey
* publicKey: publicKey
* ```
*
* ## Import
* MongoDB Atlas secret backends can be imported using the `${mount}/config`, e.g.
* ```sh
* $ pulumi import vault:mongodbatlas/secretBackend:SecretBackend config mongodbatlas/config
* ```
* @property mount Path where the MongoDB Atlas Secrets Engine is mounted.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property privateKey Specifies the Private API Key used to authenticate with the MongoDB Atlas API.
* @property publicKey Specifies the Public API Key used to authenticate with the MongoDB Atlas API.
*/
public data class SecretBackendArgs(
public val mount: Output? = null,
public val namespace: Output? = null,
public val privateKey: Output? = null,
public val publicKey: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.mongodbatlas.SecretBackendArgs =
com.pulumi.vault.mongodbatlas.SecretBackendArgs.builder()
.mount(mount?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.privateKey(privateKey?.applyValue({ args0 -> args0 }))
.publicKey(publicKey?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendArgs].
*/
@PulumiTagMarker
public class SecretBackendArgsBuilder internal constructor() {
private var mount: Output? = null
private var namespace: Output? = null
private var privateKey: Output? = null
private var publicKey: Output? = null
/**
* @param value Path where the MongoDB Atlas Secrets Engine is mounted.
*/
@JvmName("ghuntlberaeascbr")
public suspend fun mount(`value`: Output) {
this.mount = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("ypoultyyofhdhmar")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Specifies the Private API Key used to authenticate with the MongoDB Atlas API.
*/
@JvmName("huyarxgbuwrhdrkw")
public suspend fun privateKey(`value`: Output) {
this.privateKey = value
}
/**
* @param value Specifies the Public API Key used to authenticate with the MongoDB Atlas API.
*/
@JvmName("qskhbnknxvegxbco")
public suspend fun publicKey(`value`: Output) {
this.publicKey = value
}
/**
* @param value Path where the MongoDB Atlas Secrets Engine is mounted.
*/
@JvmName("krkivunaegywrgkq")
public suspend fun mount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mount = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("sqmsresykbfuhril")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Specifies the Private API Key used to authenticate with the MongoDB Atlas API.
*/
@JvmName("qqpsocpjgwjrwubi")
public suspend fun privateKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateKey = mapped
}
/**
* @param value Specifies the Public API Key used to authenticate with the MongoDB Atlas API.
*/
@JvmName("apmneqlmbrniutxk")
public suspend fun publicKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicKey = mapped
}
internal fun build(): SecretBackendArgs = SecretBackendArgs(
mount = mount,
namespace = namespace,
privateKey = privateKey,
publicKey = publicKey,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy