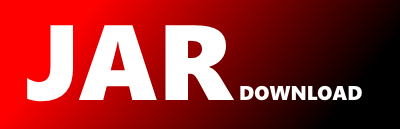
com.pulumi.vault.mongodbatlas.kotlin.SecretRoleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.mongodbatlas.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.mongodbatlas.SecretRoleArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const mongo = new vault.Mount("mongo", {
* path: "%s",
* type: "mongodbatlas",
* description: "MongoDB Atlas secret engine mount",
* });
* const config = new vault.mongodbatlas.SecretBackend("config", {
* mount: mongo.path,
* privateKey: "privateKey",
* publicKey: "publicKey",
* });
* const role = new vault.mongodbatlas.SecretRole("role", {
* mount: mongo.path,
* name: "tf-test-role",
* organizationId: "7cf5a45a9ccf6400e60981b7",
* projectId: "5cf5a45a9ccf6400e60981b6",
* roles: ["ORG_READ_ONLY"],
* ipAddresses: "192.168.1.5, 192.168.1.6",
* cidrBlocks: "192.168.1.3/35",
* projectRoles: ["GROUP_READ_ONLY"],
* ttl: "60",
* maxTtl: "120",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* mongo = vault.Mount("mongo",
* path="%s",
* type="mongodbatlas",
* description="MongoDB Atlas secret engine mount")
* config = vault.mongodbatlas.SecretBackend("config",
* mount=mongo.path,
* private_key="privateKey",
* public_key="publicKey")
* role = vault.mongodbatlas.SecretRole("role",
* mount=mongo.path,
* name="tf-test-role",
* organization_id="7cf5a45a9ccf6400e60981b7",
* project_id="5cf5a45a9ccf6400e60981b6",
* roles=["ORG_READ_ONLY"],
* ip_addresses="192.168.1.5, 192.168.1.6",
* cidr_blocks="192.168.1.3/35",
* project_roles=["GROUP_READ_ONLY"],
* ttl="60",
* max_ttl="120")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var mongo = new Vault.Mount("mongo", new()
* {
* Path = "%s",
* Type = "mongodbatlas",
* Description = "MongoDB Atlas secret engine mount",
* });
* var config = new Vault.MongoDBAtlas.SecretBackend("config", new()
* {
* Mount = mongo.Path,
* PrivateKey = "privateKey",
* PublicKey = "publicKey",
* });
* var role = new Vault.MongoDBAtlas.SecretRole("role", new()
* {
* Mount = mongo.Path,
* Name = "tf-test-role",
* OrganizationId = "7cf5a45a9ccf6400e60981b7",
* ProjectId = "5cf5a45a9ccf6400e60981b6",
* Roles = new[]
* {
* "ORG_READ_ONLY",
* },
* IpAddresses = "192.168.1.5, 192.168.1.6",
* CidrBlocks = "192.168.1.3/35",
* ProjectRoles = new[]
* {
* "GROUP_READ_ONLY",
* },
* Ttl = "60",
* MaxTtl = "120",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/mongodbatlas"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* mongo, err := vault.NewMount(ctx, "mongo", &vault.MountArgs{
* Path: pulumi.String("%s"),
* Type: pulumi.String("mongodbatlas"),
* Description: pulumi.String("MongoDB Atlas secret engine mount"),
* })
* if err != nil {
* return err
* }
* _, err = mongodbatlas.NewSecretBackend(ctx, "config", &mongodbatlas.SecretBackendArgs{
* Mount: mongo.Path,
* PrivateKey: pulumi.String("privateKey"),
* PublicKey: pulumi.String("publicKey"),
* })
* if err != nil {
* return err
* }
* _, err = mongodbatlas.NewSecretRole(ctx, "role", &mongodbatlas.SecretRoleArgs{
* Mount: mongo.Path,
* Name: pulumi.String("tf-test-role"),
* OrganizationId: pulumi.String("7cf5a45a9ccf6400e60981b7"),
* ProjectId: pulumi.String("5cf5a45a9ccf6400e60981b6"),
* Roles: pulumi.StringArray{
* pulumi.String("ORG_READ_ONLY"),
* },
* IpAddresses: pulumi.StringArray("192.168.1.5, 192.168.1.6"),
* CidrBlocks: pulumi.StringArray("192.168.1.3/35"),
* ProjectRoles: pulumi.StringArray{
* pulumi.String("GROUP_READ_ONLY"),
* },
* Ttl: pulumi.String("60"),
* MaxTtl: pulumi.String("120"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.mongodbatlas.SecretBackend;
* import com.pulumi.vault.mongodbatlas.SecretBackendArgs;
* import com.pulumi.vault.mongodbatlas.SecretRole;
* import com.pulumi.vault.mongodbatlas.SecretRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var mongo = new Mount("mongo", MountArgs.builder()
* .path("%s")
* .type("mongodbatlas")
* .description("MongoDB Atlas secret engine mount")
* .build());
* var config = new SecretBackend("config", SecretBackendArgs.builder()
* .mount(mongo.path())
* .privateKey("privateKey")
* .publicKey("publicKey")
* .build());
* var role = new SecretRole("role", SecretRoleArgs.builder()
* .mount(mongo.path())
* .name("tf-test-role")
* .organizationId("7cf5a45a9ccf6400e60981b7")
* .projectId("5cf5a45a9ccf6400e60981b6")
* .roles("ORG_READ_ONLY")
* .ipAddresses("192.168.1.5, 192.168.1.6")
* .cidrBlocks("192.168.1.3/35")
* .projectRoles("GROUP_READ_ONLY")
* .ttl("60")
* .maxTtl("120")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* mongo:
* type: vault:Mount
* properties:
* path: '%s'
* type: mongodbatlas
* description: MongoDB Atlas secret engine mount
* config:
* type: vault:mongodbatlas:SecretBackend
* properties:
* mount: ${mongo.path}
* privateKey: privateKey
* publicKey: publicKey
* role:
* type: vault:mongodbatlas:SecretRole
* properties:
* mount: ${mongo.path}
* name: tf-test-role
* organizationId: 7cf5a45a9ccf6400e60981b7
* projectId: 5cf5a45a9ccf6400e60981b6
* roles:
* - ORG_READ_ONLY
* ipAddresses: 192.168.1.5, 192.168.1.6
* cidrBlocks: 192.168.1.3/35
* projectRoles:
* - GROUP_READ_ONLY
* ttl: '60'
* maxTtl: '120'
* ```
*
* ## Import
* The MongoDB Atlas secret role can be imported using the full path to the role
* of the form: `/roles/` e.g.
* ```sh
* $ pulumi import vault:mongodbatlas/secretRole:SecretRole example mongodbatlas/roles/example-role
* ```
* @property cidrBlocks Whitelist entry in CIDR notation to be added for the API key.
* @property ipAddresses IP address to be added to the whitelist for the API key.
* @property maxTtl The maximum allowed lifetime of credentials issued using this role.
* @property mount Path where the MongoDB Atlas Secrets Engine is mounted.
* @property name The name of the role.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property organizationId Unique identifier for the organization to which the target API Key belongs.
* Required if `project_id` is not set.
* @property projectId Unique identifier for the project to which the target API Key belongs.
* Required if `organization_id` is not set.
* @property projectRoles Roles assigned when an org API key is assigned to a project API key. Possible values are `GROUP_CLUSTER_MANAGER`, `GROUP_DATA_ACCESS_ADMIN`, `GROUP_DATA_ACCESS_READ_ONLY`, `GROUP_DATA_ACCESS_READ_WRITE`, `GROUP_OWNER` and `GROUP_READ_ONLY`.
* @property roles List of roles that the API Key needs to have. Possible values are `ORG_OWNER`, `ORG_MEMBER`, `ORG_GROUP_CREATOR`, `ORG_BILLING_ADMIN` and `ORG_READ_ONLY`.
* @property ttl Duration in seconds after which the issued credential should expire.
*/
public data class SecretRoleArgs(
public val cidrBlocks: Output>? = null,
public val ipAddresses: Output>? = null,
public val maxTtl: Output? = null,
public val mount: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val organizationId: Output? = null,
public val projectId: Output? = null,
public val projectRoles: Output>? = null,
public val roles: Output>? = null,
public val ttl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.mongodbatlas.SecretRoleArgs =
com.pulumi.vault.mongodbatlas.SecretRoleArgs.builder()
.cidrBlocks(cidrBlocks?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.ipAddresses(ipAddresses?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.maxTtl(maxTtl?.applyValue({ args0 -> args0 }))
.mount(mount?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.organizationId(organizationId?.applyValue({ args0 -> args0 }))
.projectId(projectId?.applyValue({ args0 -> args0 }))
.projectRoles(projectRoles?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.roles(roles?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.ttl(ttl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretRoleArgs].
*/
@PulumiTagMarker
public class SecretRoleArgsBuilder internal constructor() {
private var cidrBlocks: Output>? = null
private var ipAddresses: Output>? = null
private var maxTtl: Output? = null
private var mount: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var organizationId: Output? = null
private var projectId: Output? = null
private var projectRoles: Output>? = null
private var roles: Output>? = null
private var ttl: Output? = null
/**
* @param value Whitelist entry in CIDR notation to be added for the API key.
*/
@JvmName("erayyaymoyltnxjo")
public suspend fun cidrBlocks(`value`: Output>) {
this.cidrBlocks = value
}
@JvmName("jfaoumxmbvxymwqn")
public suspend fun cidrBlocks(vararg values: Output) {
this.cidrBlocks = Output.all(values.asList())
}
/**
* @param values Whitelist entry in CIDR notation to be added for the API key.
*/
@JvmName("egihlwxgdrokshyr")
public suspend fun cidrBlocks(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy