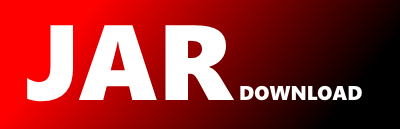
com.pulumi.vault.okta.kotlin.AuthBackend.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.okta.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import com.pulumi.vault.okta.kotlin.outputs.AuthBackendGroup
import com.pulumi.vault.okta.kotlin.outputs.AuthBackendUser
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.vault.okta.kotlin.outputs.AuthBackendGroup.Companion.toKotlin as authBackendGroupToKotlin
import com.pulumi.vault.okta.kotlin.outputs.AuthBackendUser.Companion.toKotlin as authBackendUserToKotlin
/**
* Builder for [AuthBackend].
*/
@PulumiTagMarker
public class AuthBackendResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuthBackendArgs = AuthBackendArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuthBackendArgsBuilder.() -> Unit) {
val builder = AuthBackendArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AuthBackend {
val builtJavaResource = com.pulumi.vault.okta.AuthBackend(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AuthBackend(builtJavaResource)
}
}
/**
* Provides a resource for managing an
* [Okta auth backend within Vault](https://www.vaultproject.io/docs/auth/okta.html).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.okta.AuthBackend("example", {
* description: "Demonstration of the Terraform Okta auth backend",
* organization: "example",
* token: "something that should be kept secret",
* groups: [{
* groupName: "foo",
* policies: [
* "one",
* "two",
* ],
* }],
* users: [{
* username: "bar",
* groups: ["foo"],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.okta.AuthBackend("example",
* description="Demonstration of the Terraform Okta auth backend",
* organization="example",
* token="something that should be kept secret",
* groups=[vault.okta.AuthBackendGroupArgs(
* group_name="foo",
* policies=[
* "one",
* "two",
* ],
* )],
* users=[vault.okta.AuthBackendUserArgs(
* username="bar",
* groups=["foo"],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.Okta.AuthBackend("example", new()
* {
* Description = "Demonstration of the Terraform Okta auth backend",
* Organization = "example",
* Token = "something that should be kept secret",
* Groups = new[]
* {
* new Vault.Okta.Inputs.AuthBackendGroupArgs
* {
* GroupName = "foo",
* Policies = new[]
* {
* "one",
* "two",
* },
* },
* },
* Users = new[]
* {
* new Vault.Okta.Inputs.AuthBackendUserArgs
* {
* Username = "bar",
* Groups = new[]
* {
* "foo",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/okta"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := okta.NewAuthBackend(ctx, "example", &okta.AuthBackendArgs{
* Description: pulumi.String("Demonstration of the Terraform Okta auth backend"),
* Organization: pulumi.String("example"),
* Token: pulumi.String("something that should be kept secret"),
* Groups: okta.AuthBackendGroupTypeArray{
* &okta.AuthBackendGroupTypeArgs{
* GroupName: pulumi.String("foo"),
* Policies: pulumi.StringArray{
* pulumi.String("one"),
* pulumi.String("two"),
* },
* },
* },
* Users: okta.AuthBackendUserTypeArray{
* &okta.AuthBackendUserTypeArgs{
* Username: pulumi.String("bar"),
* Groups: pulumi.StringArray{
* pulumi.String("foo"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.okta.AuthBackend;
* import com.pulumi.vault.okta.AuthBackendArgs;
* import com.pulumi.vault.okta.inputs.AuthBackendGroupArgs;
* import com.pulumi.vault.okta.inputs.AuthBackendUserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AuthBackend("example", AuthBackendArgs.builder()
* .description("Demonstration of the Terraform Okta auth backend")
* .organization("example")
* .token("something that should be kept secret")
* .groups(AuthBackendGroupArgs.builder()
* .groupName("foo")
* .policies(
* "one",
* "two")
* .build())
* .users(AuthBackendUserArgs.builder()
* .username("bar")
* .groups("foo")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:okta:AuthBackend
* properties:
* description: Demonstration of the Terraform Okta auth backend
* organization: example
* token: something that should be kept secret
* groups:
* - groupName: foo
* policies:
* - one
* - two
* users:
* - username: bar
* groups:
* - foo
* ```
*
* ## Import
* Okta authentication backends can be imported using its `path`, e.g.
* ```sh
* $ pulumi import vault:okta/authBackend:AuthBackend example okta
* ```
*/
public class AuthBackend internal constructor(
override val javaResource: com.pulumi.vault.okta.AuthBackend,
) : KotlinCustomResource(javaResource, AuthBackendMapper) {
/**
* The mount accessor related to the auth mount. It is useful for integration with [Identity Secrets Engine](https://www.vaultproject.io/docs/secrets/identity/index.html).
*/
public val accessor: Output
get() = javaResource.accessor().applyValue({ args0 -> args0 })
/**
* The Okta url. Examples: oktapreview.com, okta.com
*/
public val baseUrl: Output?
get() = javaResource.baseUrl().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* When true, requests by Okta for a MFA check will be bypassed. This also disallows certain status checks on the account, such as whether the password is expired.
*/
public val bypassOktaMfa: Output?
get() = javaResource.bypassOktaMfa().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The description of the auth backend
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
public val disableRemount: Output?
get() = javaResource.disableRemount().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Associate Okta groups with policies within Vault.
* See below for more details.
*/
public val groups: Output>
get() = javaResource.groups().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
authBackendGroupToKotlin(args0)
})
})
})
/**
* Maximum duration after which authentication will be expired
* [See the documentation for info on valid duration formats](https://golang.org/pkg/time/#ParseDuration).
*/
@Deprecated(
message = """
Deprecated. Please use `token_max_ttl` instead.
""",
)
public val maxTtl: Output?
get() = javaResource.maxTtl().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Okta organization. This will be the first part of the url `https://XXX.okta.com`
*/
public val organization: Output
get() = javaResource.organization().applyValue({ args0 -> args0 })
/**
* Path to mount the Okta auth backend. Default to path `okta`.
*/
public val path: Output?
get() = javaResource.path().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Okta API token. This is required to query Okta for user group membership.
* If this is not supplied only locally configured groups will be enabled.
*/
public val token: Output?
get() = javaResource.token().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies the blocks of IP addresses which are allowed to use the generated token
*/
public val tokenBoundCidrs: Output>?
get() = javaResource.tokenBoundCidrs().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Generated Token's Explicit Maximum TTL in seconds
*/
public val tokenExplicitMaxTtl: Output?
get() = javaResource.tokenExplicitMaxTtl().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum lifetime of the generated token
*/
public val tokenMaxTtl: Output?
get() = javaResource.tokenMaxTtl().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If true, the 'default' policy will not automatically be added to generated tokens
*/
public val tokenNoDefaultPolicy: Output?
get() = javaResource.tokenNoDefaultPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum number of times a token may be used, a value of zero means unlimited
*/
public val tokenNumUses: Output?
get() = javaResource.tokenNumUses().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Generated Token's Period
*/
public val tokenPeriod: Output?
get() = javaResource.tokenPeriod().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Generated Token's Policies
*/
public val tokenPolicies: Output>?
get() = javaResource.tokenPolicies().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The initial ttl of the token to generate in seconds
*/
public val tokenTtl: Output?
get() = javaResource.tokenTtl().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The type of token to generate, service or batch
*/
public val tokenType: Output?
get() = javaResource.tokenType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Duration after which authentication will be expired.
* [See the documentation for info on valid duration formats](https://golang.org/pkg/time/#ParseDuration).
*/
@Deprecated(
message = """
Deprecated. Please use `token_ttl` instead.
""",
)
public val ttl: Output?
get() = javaResource.ttl().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Associate Okta users with groups or policies within Vault.
* See below for more details.
*/
public val users: Output>
get() = javaResource.users().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
authBackendUserToKotlin(args0)
})
})
})
}
public object AuthBackendMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.okta.AuthBackend::class == javaResource::class
override fun map(javaResource: Resource): AuthBackend = AuthBackend(
javaResource as
com.pulumi.vault.okta.AuthBackend,
)
}
/**
* @see [AuthBackend].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AuthBackend].
*/
public suspend fun authBackend(name: String, block: suspend AuthBackendResourceBuilder.() -> Unit): AuthBackend {
val builder = AuthBackendResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AuthBackend].
* @param name The _unique_ name of the resulting resource.
*/
public fun authBackend(name: String): AuthBackend {
val builder = AuthBackendResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy