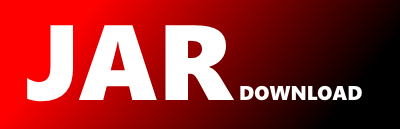
com.pulumi.vault.okta.kotlin.AuthBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.okta.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.vault.okta.AuthBackendArgs.builder
import com.pulumi.vault.okta.kotlin.inputs.AuthBackendGroupArgs
import com.pulumi.vault.okta.kotlin.inputs.AuthBackendGroupArgsBuilder
import com.pulumi.vault.okta.kotlin.inputs.AuthBackendUserArgs
import com.pulumi.vault.okta.kotlin.inputs.AuthBackendUserArgsBuilder
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a resource for managing an
* [Okta auth backend within Vault](https://www.vaultproject.io/docs/auth/okta.html).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const example = new vault.okta.AuthBackend("example", {
* description: "Demonstration of the Terraform Okta auth backend",
* organization: "example",
* token: "something that should be kept secret",
* groups: [{
* groupName: "foo",
* policies: [
* "one",
* "two",
* ],
* }],
* users: [{
* username: "bar",
* groups: ["foo"],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* example = vault.okta.AuthBackend("example",
* description="Demonstration of the Terraform Okta auth backend",
* organization="example",
* token="something that should be kept secret",
* groups=[vault.okta.AuthBackendGroupArgs(
* group_name="foo",
* policies=[
* "one",
* "two",
* ],
* )],
* users=[vault.okta.AuthBackendUserArgs(
* username="bar",
* groups=["foo"],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var example = new Vault.Okta.AuthBackend("example", new()
* {
* Description = "Demonstration of the Terraform Okta auth backend",
* Organization = "example",
* Token = "something that should be kept secret",
* Groups = new[]
* {
* new Vault.Okta.Inputs.AuthBackendGroupArgs
* {
* GroupName = "foo",
* Policies = new[]
* {
* "one",
* "two",
* },
* },
* },
* Users = new[]
* {
* new Vault.Okta.Inputs.AuthBackendUserArgs
* {
* Username = "bar",
* Groups = new[]
* {
* "foo",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/okta"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := okta.NewAuthBackend(ctx, "example", &okta.AuthBackendArgs{
* Description: pulumi.String("Demonstration of the Terraform Okta auth backend"),
* Organization: pulumi.String("example"),
* Token: pulumi.String("something that should be kept secret"),
* Groups: okta.AuthBackendGroupTypeArray{
* &okta.AuthBackendGroupTypeArgs{
* GroupName: pulumi.String("foo"),
* Policies: pulumi.StringArray{
* pulumi.String("one"),
* pulumi.String("two"),
* },
* },
* },
* Users: okta.AuthBackendUserTypeArray{
* &okta.AuthBackendUserTypeArgs{
* Username: pulumi.String("bar"),
* Groups: pulumi.StringArray{
* pulumi.String("foo"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.okta.AuthBackend;
* import com.pulumi.vault.okta.AuthBackendArgs;
* import com.pulumi.vault.okta.inputs.AuthBackendGroupArgs;
* import com.pulumi.vault.okta.inputs.AuthBackendUserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new AuthBackend("example", AuthBackendArgs.builder()
* .description("Demonstration of the Terraform Okta auth backend")
* .organization("example")
* .token("something that should be kept secret")
* .groups(AuthBackendGroupArgs.builder()
* .groupName("foo")
* .policies(
* "one",
* "two")
* .build())
* .users(AuthBackendUserArgs.builder()
* .username("bar")
* .groups("foo")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: vault:okta:AuthBackend
* properties:
* description: Demonstration of the Terraform Okta auth backend
* organization: example
* token: something that should be kept secret
* groups:
* - groupName: foo
* policies:
* - one
* - two
* users:
* - username: bar
* groups:
* - foo
* ```
*
* ## Import
* Okta authentication backends can be imported using its `path`, e.g.
* ```sh
* $ pulumi import vault:okta/authBackend:AuthBackend example okta
* ```
* @property baseUrl The Okta url. Examples: oktapreview.com, okta.com
* @property bypassOktaMfa When true, requests by Okta for a MFA check will be bypassed. This also disallows certain status checks on the account, such as whether the password is expired.
* @property description The description of the auth backend
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property groups Associate Okta groups with policies within Vault.
* See below for more details.
* @property maxTtl Maximum duration after which authentication will be expired
* [See the documentation for info on valid duration formats](https://golang.org/pkg/time/#ParseDuration).
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property organization The Okta organization. This will be the first part of the url `https://XXX.okta.com`
* @property path Path to mount the Okta auth backend. Default to path `okta`.
* @property token The Okta API token. This is required to query Okta for user group membership.
* If this is not supplied only locally configured groups will be enabled.
* @property tokenBoundCidrs Specifies the blocks of IP addresses which are allowed to use the generated token
* @property tokenExplicitMaxTtl Generated Token's Explicit Maximum TTL in seconds
* @property tokenMaxTtl The maximum lifetime of the generated token
* @property tokenNoDefaultPolicy If true, the 'default' policy will not automatically be added to generated tokens
* @property tokenNumUses The maximum number of times a token may be used, a value of zero means unlimited
* @property tokenPeriod Generated Token's Period
* @property tokenPolicies Generated Token's Policies
* @property tokenTtl The initial ttl of the token to generate in seconds
* @property tokenType The type of token to generate, service or batch
* @property ttl Duration after which authentication will be expired.
* [See the documentation for info on valid duration formats](https://golang.org/pkg/time/#ParseDuration).
* @property users Associate Okta users with groups or policies within Vault.
* See below for more details.
*/
public data class AuthBackendArgs(
public val baseUrl: Output? = null,
public val bypassOktaMfa: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val groups: Output>? = null,
@Deprecated(
message = """
Deprecated. Please use `token_max_ttl` instead.
""",
)
public val maxTtl: Output? = null,
public val namespace: Output? = null,
public val organization: Output? = null,
public val path: Output? = null,
public val token: Output? = null,
public val tokenBoundCidrs: Output>? = null,
public val tokenExplicitMaxTtl: Output? = null,
public val tokenMaxTtl: Output? = null,
public val tokenNoDefaultPolicy: Output? = null,
public val tokenNumUses: Output? = null,
public val tokenPeriod: Output? = null,
public val tokenPolicies: Output>? = null,
public val tokenTtl: Output? = null,
public val tokenType: Output? = null,
@Deprecated(
message = """
Deprecated. Please use `token_ttl` instead.
""",
)
public val ttl: Output? = null,
public val users: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.okta.AuthBackendArgs =
com.pulumi.vault.okta.AuthBackendArgs.builder()
.baseUrl(baseUrl?.applyValue({ args0 -> args0 }))
.bypassOktaMfa(bypassOktaMfa?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.groups(groups?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.maxTtl(maxTtl?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.organization(organization?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.token(token?.applyValue({ args0 -> args0 }))
.tokenBoundCidrs(tokenBoundCidrs?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenExplicitMaxTtl(tokenExplicitMaxTtl?.applyValue({ args0 -> args0 }))
.tokenMaxTtl(tokenMaxTtl?.applyValue({ args0 -> args0 }))
.tokenNoDefaultPolicy(tokenNoDefaultPolicy?.applyValue({ args0 -> args0 }))
.tokenNumUses(tokenNumUses?.applyValue({ args0 -> args0 }))
.tokenPeriod(tokenPeriod?.applyValue({ args0 -> args0 }))
.tokenPolicies(tokenPolicies?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenTtl(tokenTtl?.applyValue({ args0 -> args0 }))
.tokenType(tokenType?.applyValue({ args0 -> args0 }))
.ttl(ttl?.applyValue({ args0 -> args0 }))
.users(
users?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [AuthBackendArgs].
*/
@PulumiTagMarker
public class AuthBackendArgsBuilder internal constructor() {
private var baseUrl: Output? = null
private var bypassOktaMfa: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var groups: Output>? = null
private var maxTtl: Output? = null
private var namespace: Output? = null
private var organization: Output? = null
private var path: Output? = null
private var token: Output? = null
private var tokenBoundCidrs: Output>? = null
private var tokenExplicitMaxTtl: Output? = null
private var tokenMaxTtl: Output? = null
private var tokenNoDefaultPolicy: Output? = null
private var tokenNumUses: Output? = null
private var tokenPeriod: Output? = null
private var tokenPolicies: Output>? = null
private var tokenTtl: Output? = null
private var tokenType: Output? = null
private var ttl: Output? = null
private var users: Output>? = null
/**
* @param value The Okta url. Examples: oktapreview.com, okta.com
*/
@JvmName("dplplnsafbebqxog")
public suspend fun baseUrl(`value`: Output) {
this.baseUrl = value
}
/**
* @param value When true, requests by Okta for a MFA check will be bypassed. This also disallows certain status checks on the account, such as whether the password is expired.
*/
@JvmName("rgxsjulntfcuybif")
public suspend fun bypassOktaMfa(`value`: Output) {
this.bypassOktaMfa = value
}
/**
* @param value The description of the auth backend
*/
@JvmName("gwdfcorpatrchxsn")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("ikilixfqphtghhyx")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value Associate Okta groups with policies within Vault.
* See below for more details.
*/
@JvmName("ccqtnolducihtrhj")
public suspend fun groups(`value`: Output>) {
this.groups = value
}
@JvmName("obcrufcydxqclsdw")
public suspend fun groups(vararg values: Output) {
this.groups = Output.all(values.asList())
}
/**
* @param values Associate Okta groups with policies within Vault.
* See below for more details.
*/
@JvmName("anvmtjxdupnebldt")
public suspend fun groups(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy