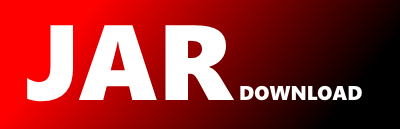
com.pulumi.vault.pkiSecret.kotlin.BackendConfigEst.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.pkiSecret.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import com.pulumi.vault.pkiSecret.kotlin.outputs.BackendConfigEstAuthenticators
import com.pulumi.vault.pkiSecret.kotlin.outputs.BackendConfigEstAuthenticators.Companion.toKotlin
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [BackendConfigEst].
*/
@PulumiTagMarker
public class BackendConfigEstResourceBuilder internal constructor() {
public var name: String? = null
public var args: BackendConfigEstArgs = BackendConfigEstArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BackendConfigEstArgsBuilder.() -> Unit) {
val builder = BackendConfigEstArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BackendConfigEst {
val builtJavaResource = com.pulumi.vault.pkiSecret.BackendConfigEst(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BackendConfigEst(builtJavaResource)
}
}
/**
* Allows setting the EST configuration on a PKI Secret Backend
* ## Import
* The PKI config cluster can be imported using the resource's `id`.
* In the case of the example above the `id` would be `pki-root/config/est`,
* where the `pki-root` component is the resource's `backend`, e.g.
* ```sh
* $ pulumi import vault:pkiSecret/backendConfigEst:BackendConfigEst example pki-root/config/est
* ```
*/
public class BackendConfigEst internal constructor(
override val javaResource: com.pulumi.vault.pkiSecret.BackendConfigEst,
) : KotlinCustomResource(javaResource, BackendConfigEstMapper) {
/**
* Fields parsed from the CSR that appear in the audit and can be used by sentinel policies.
*
*/
public val auditFields: Output>
get() = javaResource.auditFields().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Lists the mount accessors EST should delegate authentication requests towards (see below for nested schema).
*/
public val authenticators: Output
get() = javaResource.authenticators().applyValue({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
/**
* The path to the PKI secret backend to
* read the EST configuration from, with no leading or trailing `/`s.
*/
public val backend: Output
get() = javaResource.backend().applyValue({ args0 -> args0 })
/**
* If set, this mount will register the default `.well-known/est` URL path. Only a single mount can enable this across a Vault cluster.
*/
public val defaultMount: Output?
get() = javaResource.defaultMount().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Required to be set if default_mount is enabled. Specifies the behavior for requests using the default EST label. Can be sign-verbatim or a role given by role:.
*/
public val defaultPathPolicy: Output?
get() = javaResource.defaultPathPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, parse out fields from the provided CSR making them available for Sentinel policies.
*/
public val enableSentinelParsing: Output?
get() = javaResource.enableSentinelParsing().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether EST is enabled.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Configures a pairing of an EST label with the redirected behavior for requests hitting that role. The path policy can be sign-verbatim or a role given by role:. Labels must be unique across Vault cluster, and will register .well-known/est/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy